How to Compare Strings in Python
- How to Compare Strings in Python Using Relational Operators
-
How to Compare Strings in Python Using the
is
Operator - How to Compare Strings in Python Using User-Defined Logic
- How to Compare Strings in Python Using Regular Expressions
- Conclusion
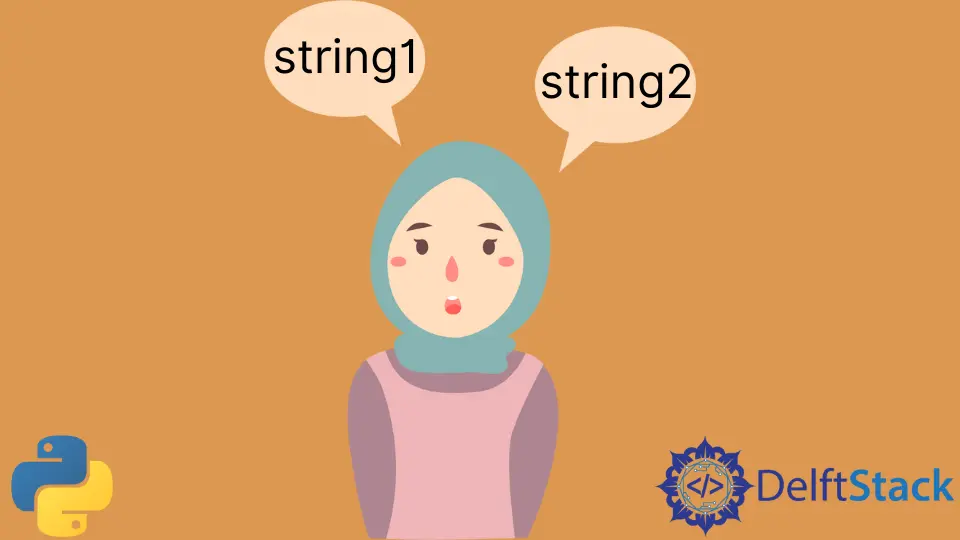
In Python programming, string comparison is a fundamental operation that plays a crucial role in various applications. Whether it’s validating user input, sorting data, or implementing complex algorithms, the ability to compare strings efficiently is an indispensable skill for developers.
This article delves into the diverse methods available for comparing strings in Python, exploring built-in operators, user-defined logic, and the powerful realm of regular expressions. By understanding the nuances of these techniques, you can make informed decisions to tailor string comparisons to your specific needs.
How to Compare Strings in Python Using Relational Operators
One of the most straightforward methods for comparing strings is using relational operators, which allow us to perform comparisons between strings based on their Unicode values.
Relational Operators
Relational or comparison operators are used to compare values and determine the relationship between them. In Python, the following relational operators can be used to compare strings:
Operator | Description |
---|---|
== |
Checks if two strings are equal. |
!= |
Checks if two strings are not equal. |
< |
Checks if one string is lexicographically less than another. |
> |
Checks if one string is lexicographically greater than another. |
<= |
Checks if one string is lexicographically less than or equal to another. |
>= |
Checks if one string is lexicographically greater than or equal to another. |
Use the Equal (==
) and Not Equal (!=
) Operators
The ==
operator checks if two strings are equal, while !=
checks if they are not. Here’s an example:
str1 = "Hello"
str2 = "hello"
if str1 == str2:
print("The strings are equal.")
else:
print("The strings are not equal.")
Code Output:
In this case, the output indicates that the strings are not equal since the comparison is case-sensitive.
Use the Less Than (<
) and Greater Than (>
) Operators
The <
operator checks if one string is lexicographically less than another, and >
checks if one string is lexicographically greater.
This means that it checks the Unicode code point of each character from left to right. The first character that differs determines the outcome of the comparison.
Here’s an example:
str1 = "apple"
str2 = "banana"
if str1 < str2:
print("str1 comes before str2.")
else:
print("str1 comes after str2.")
Code Output:
Here, the output indicates that str1
is lexicographically less than str2
.
Use the Less Than or Equal (<=
) and Greater Than or Equal (>=
) Operators
The <=
operator checks if one string is lexicographically less than or equal to another, and >=
checks if one string is lexicographically greater than or equal. Here’s an example:
str1 = "apple"
str2 = "banana"
if str1 <= str2:
print("str1 is less than or equal to str2.")
else:
print("str1 is greater than str2.")
Code Output:
In this case, the output determines that str1
is lexicographically less than or equal to str2
.
Case-Insensitive String Comparison
It’s important to note that using Python string comparison operators is case-sensitive. For example, hello
and Hello
are considered different strings.
If you want to perform a case-insensitive comparison, you can convert the strings to lowercase (or uppercase) using the lower()
or upper()
methods before using the comparison operators.
Here’s an example:
str1 = "Hello"
str2 = "hello"
if str1.lower() == str2.lower():
print("The strings are equal (case-insensitive).")
else:
print("The strings are not equal (case-insensitive).")
Code Output:
How to Compare Strings in Python Using the is
Operator
In Python, the is
operator is used for identity comparison, checking if two variables refer to the same object in memory. While it is commonly used for comparing objects, including strings, it is important to note that it checks for identity rather than equality.
When used for string comparison, it checks if both variables point to the identical string object. This is different from the equality operator (==
), which compares the content of the strings rather than their identity.
It is crucial to differentiate between equality (whether the values are the same) and identity (whether the variables point to the same memory location).
For example:
# Python string comparison using the `is` operator
str1 = "hello"
str2 = "hello"
str3 = "world"
# Using the `is` operator
print(f"str1 is str2: {str1 is str2}")
print(f"str1 is str3: {str1 is str3}")
In this example code, we have three strings: str1
and str2
, both set to hello
, and str3
set to world
. The is
operator is then employed to compare these strings.
The first line (str1 is str2
) checks if str1
and str2
reference the same string object. Since identical string literals often share the same memory location in Python, this evaluates to True
.
The second line (str1 is str3
) checks if str1
and str3
reference the same string object. Since they have different values, even though the content is the same, this evaluates to False
.
Remember that the is
operator is not used for comparing the content of strings but rather their identity in memory.
Code Output:
This output reflects the results of applying the is
operator for string comparison. Use the is
operator judiciously, especially when you specifically want to check if two variables reference the same string object in memory.
How to Compare Strings in Python Using User-Defined Logic
Besides using built-in operators, we can implement our custom logic for Python string comparison. This approach allows us to compare strings based on specific criteria, such as length or other user-defined factors.
Let’s consider an example where we create a user-defined function to compare the lengths of two strings:
# User-defined logic for comparing strings
def compare_lengths(s1, s2):
len_s1 = len(s1)
len_s2 = len(s2)
if len_s1 > len_s2:
print(f"{s1} is longer than {s2}")
elif len_s1 == len_s2:
print("Both strings have equal length")
else:
print(f"{s2} is longer than {s1}")
# Example usage
str1 = "apple"
str2 = "orange"
compare_lengths(str1, str2)
In the provided code, we define a function compare_lengths
that takes two string parameters, s1
and s2
.
Inside the function, we calculate the lengths of both the strings using the len()
function. We then use an if-elif-else
statement to compare the lengths and print a message accordingly.
If len_s1
is greater than len_s2
, we print that s1
is longer than s2
. If the lengths are equal, we print that both strings have equal length. Otherwise, we print that s2
is longer than s1
.
The example usage at the end demonstrates how to call this function with two strings, apple
and orange
.
Code Output:
This output shows the result of the string length comparison based on the user-defined logic. By employing custom logic, we can tailor string comparisons to specific requirements, providing flexibility in our Python programs.
How to Compare Strings in Python Using Regular Expressions
In Python, regular expressions offer a powerful way to compare strings by defining patterns. Regular expressions (often abbreviated as regex) provide a flexible and concise method for matching specific sequences of characters.
Consider the following example, where we use the re
module to compare two strings based on a specific pattern:
import re
# Strings for comparison
str1 = "apple"
str2 = "orange"
# Function to check a pattern using regular expressions
def check_pattern(s):
if re.match("ap[a-z]+", s):
print(f"Pattern matched: {s}")
else:
print(f"Pattern not matched: {s}")
# Example usage
check_pattern(str1)
check_pattern(str2)
In this example code, the check_pattern
function is defined to take a string parameter s
. Inside the function:
- We use
re.match(pattern, string)
to check if the string matches a specific pattern. - The pattern
ap[a-z]+
checks if the string starts withap
followed by one or more lowercase letters. - If the pattern matches, we print that the pattern is matched; otherwise, we print that the pattern is not matched.
The example usage demonstrates how to call this function with two strings, apple
and orange.
Code Output:
Case-Insensitive String Comparison Using Regular Expressions
Regular expressions also allow for case-insensitive comparisons by using the re.IGNORECASE
flag. Here’s an example:
import re
pattern = r"hello"
string = "Hello, World!"
if re.search(pattern, string, re.IGNORECASE):
print("String contains 'hello' (case-insensitive)")
else:
print("String does not contain 'hello'")
In this case, the re.IGNORECASE
flag enables case-insensitive comparison.
Code Output:
Matching Patterns Anywhere in the String Using Regular Expressions
If you want to check if a pattern exists anywhere in the string, you can use re.search()
:
import re
pattern = r"Python"
string = "I love Python programming."
if re.search(pattern, string):
print("String contains 'Python'")
else:
print("String does not contain 'Python'")
The re.search()
function looks for the pattern anywhere in the string.
Code Output:
Complex String Comparisons Using Regular Expressions
Regular expressions become particularly powerful when dealing with complex patterns. For example, suppose you want to check if a string follows a specific format:
import re
pattern = r"\d{3}-\d{2}-\d{4}" # Matches a Social Security Number format
ssn = "123-45-6789"
if re.match(pattern, ssn):
print("String is a valid Social Security Number")
else:
print("String is not a valid Social Security Number")
In this case, the pattern \d{3}-\d{2}-\d{4}
represents the format for a Social Security Number.
Code Output:
Conclusion
Comparing strings in Python involves various techniques, each suited to specific scenarios. By understanding and utilizing different methods, developers gain the flexibility to tailor comparisons to their specific needs.
The use of Python comparison operators allows for straightforward comparisons based on Unicode values, while the is
operator checks for identity, determining if two strings reference the same object in memory.
User-defined logic provides a customizable approach, enabling comparisons based on criteria such as string length. Additionally, regular expressions offer a powerful and flexible means of matching patterns within strings.
Choosing the appropriate method depends on the context of the comparison, considering factors like case sensitivity, specific patterns, or custom criteria. As Python provides a rich set of tools for string manipulation and comparison, you can select the method that best fits the requirements of your applications.
To further improve your coding skills in Python, after learning about comparing strings, the next specific topic you can explore is comparing lists in Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn