How to Compare Lists in Python
- Using the ‘==’ Operator
- Using the ‘set()’ Function
- Using List Comprehensions
- Using the ‘collections.Counter’ Class
- Conclusion
- FAQ
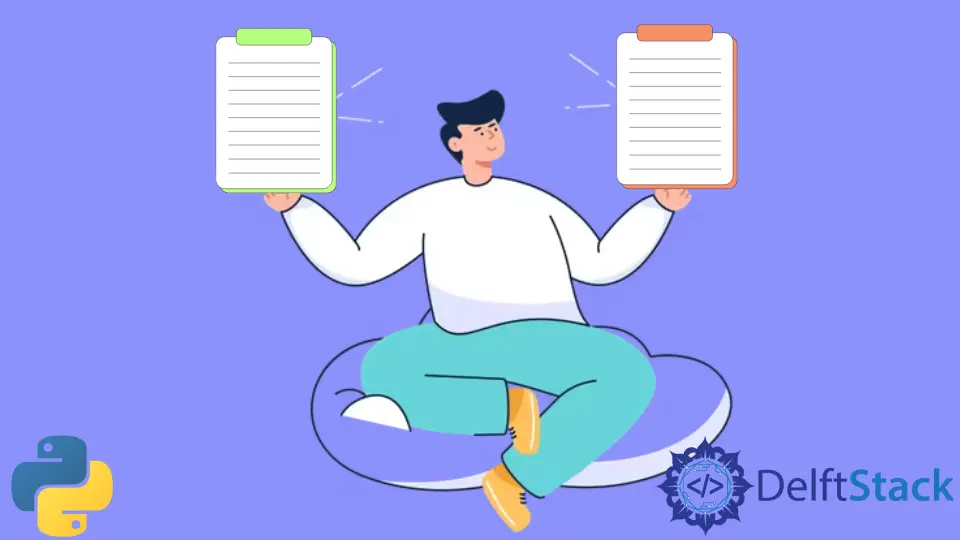
When working with data in Python, comparing lists is a common task that developers often encounter. Whether you’re checking for duplicates, finding differences, or identifying common elements, knowing how to effectively compare lists can save you time and effort.
In this tutorial, we will explore various methods for comparing lists in Python, complete with code examples and detailed explanations. By the end of this guide, you’ll have a solid understanding of how to tackle list comparisons, making your coding experience smoother and more efficient. Let’s dive in!
Using the ‘==’ Operator
One of the simplest ways to compare two lists in Python is by using the equality operator (==
). This operator checks whether the two lists contain the same elements in the same order. If they do, it returns True
; otherwise, it returns False
. This method is straightforward and works well for most use cases where order matters.
list1 = [1, 2, 3, 4]
list2 = [1, 2, 3, 4]
list3 = [4, 3, 2, 1]
comparison1 = list1 == list2
comparison2 = list1 == list3
print(comparison1)
print(comparison2)
Output:
True
False
Using the ==
operator is beneficial for quick checks, especially when you want to verify if two lists are identical. However, keep in mind that this method is sensitive to the order of elements. If the order differs, even with the same elements, it will return False
. This method is ideal when you need a straightforward comparison without additional complexity.
Using the ‘set()’ Function
Another effective way to compare lists is by converting them into sets using the set()
function. Sets are unordered collections of unique elements, which makes them perfect for checking for commonality or differences between two lists. This method disregards the order of elements and only focuses on the unique values.
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
set1 = set(list1)
set2 = set(list2)
common_elements = set1.intersection(set2)
unique_to_list1 = set1.difference(set2)
unique_to_list2 = set2.difference(set1)
print(common_elements)
print(unique_to_list1)
print(unique_to_list2)
Output:
{3, 4}
{1, 2}
{5, 6}
Using sets for comparison is particularly useful when you want to identify common elements or find out which items are unique to each list. The intersection()
method retrieves items present in both sets, while the difference()
method shows what is unique to each list. This approach is efficient, especially when dealing with large datasets, as it reduces the complexity of the comparison.
Using List Comprehensions
List comprehensions provide a powerful and concise way to compare lists in Python. You can use them to filter elements based on specific conditions, making it easy to find differences or commonalities between two lists. This method is both readable and efficient.
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
common_elements = [item for item in list1 if item in list2]
unique_to_list1 = [item for item in list1 if item not in list2]
unique_to_list2 = [item for item in list2 if item not in list1]
print(common_elements)
print(unique_to_list1)
print(unique_to_list2)
Output:
[3, 4]
[1, 2]
[5, 6]
List comprehensions are not only elegant but also allow for custom conditions to be applied during comparisons. This flexibility makes them a favorite among developers who need to perform more complex comparisons. The resulting lists can be easily manipulated or processed further, making this method highly versatile for various applications.
Using the ‘collections.Counter’ Class
For more advanced comparisons, especially when dealing with lists that may contain duplicates, the Counter
class from the collections
module is a great option. It counts the occurrences of each element in the list, allowing for a more nuanced comparison.
from collections import Counter
list1 = [1, 2, 2, 3, 4]
list2 = [2, 3, 3, 5, 6]
counter1 = Counter(list1)
counter2 = Counter(list2)
common_elements = counter1 & counter2
unique_to_list1 = counter1 - counter2
unique_to_list2 = counter2 - counter1
print(common_elements)
print(unique_to_list1)
print(unique_to_list2)
Output:
Counter({2: 2, 3: 1})
Counter({1: 1, 2: 1, 4: 1})
Counter({3: 2, 5: 1, 6: 1})
The Counter
class is particularly useful when you need to account for the frequency of elements in your comparisons. The &
operator finds common elements along with their counts, while the -
operator identifies unique elements based on their occurrences. This method is ideal for scenarios where duplicates matter, providing a clear view of how lists relate to one another.
Conclusion
Comparing lists in Python can be achieved through various methods, each suited for different scenarios. Whether you choose to use the ==
operator for simple equality checks, sets for unique comparisons, list comprehensions for custom filtering, or the Counter
class for frequency-based comparisons, understanding these methods will enhance your programming skills. As you work with data and lists, applying the right comparison technique will lead to more efficient and effective code. Happy coding!
FAQ
- How can I check if two lists are identical?
You can use the equality operator (==) to check if two lists are identical in both content and order.
-
What if I want to compare lists without considering the order of elements?
You can convert the lists to sets using the set() function, which will allow you to compare them without considering the order. -
How do I find unique elements in each list?
You can use list comprehensions or the difference() method on sets to identify unique elements in each list. -
Can I compare lists with duplicate values?
Yes, using the collections.Counter class allows you to compare lists while accounting for the frequency of each element. -
What is the most efficient way to compare large lists?
Using sets or the Counter class can be more efficient for large lists, as they reduce complexity and provide faster comparisons.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python