How to Compare String Case Insensitive String in Python
-
Case Insensitive String Comparison With the
lower()
Method -
Case Insensitive String Comparison With the
upper()
Method -
Case Insensitive String Comparison With the
casefold()
Method
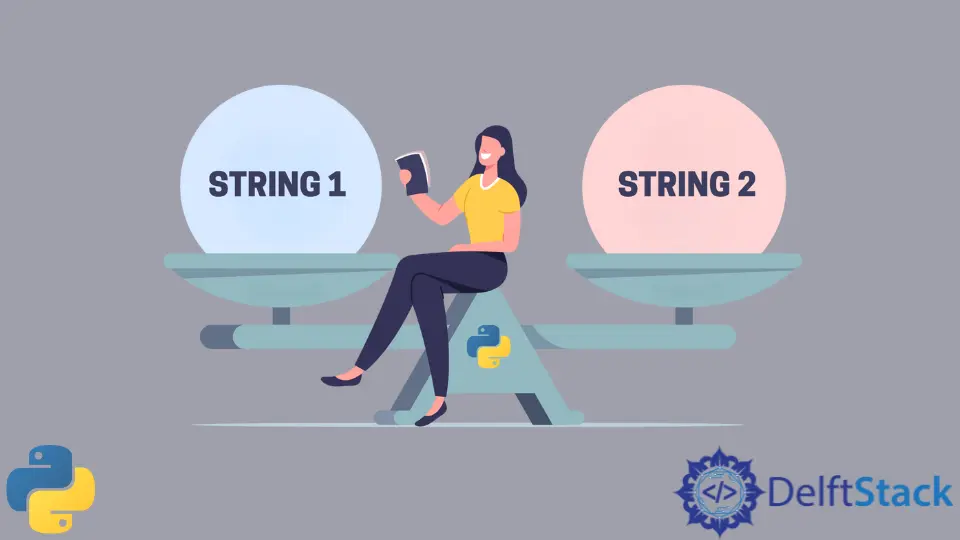
This tutorial will discuss some methods to do a case insensitive comparison of two or more string variables in Python.
Case Insensitive String Comparison With the lower()
Method
Python string has a built-in lower()
method that converts all the characters in the string to the lower case. It returns a string with all the characters converted into lower case alphabets. We can convert two strings to the lower case with the lower()
method and then compare them case-insensitively.
normal_str1 = "Hello World!"
lower_str1 = normal_str1.lower()
print(lower_str1)
Output:
hello world!
Now do the same with the second string variable normal_str2
.
normal_str2 = "HELLO WORLD!"
lower_str2 = normal_str2.lower()
print(lower_str2)
Output:
hello world!
As we can see, both strings have been converted to all lower case characters. The next step is to compare both string variables and display the output.
normal_str1 = "Hello World!"
lower_str1 = normal_str1.lower()
normal_str2 = "HELLO WORLD!"
lower_str2 = normal_str2.lower()
if(lower_str1 == lower_str2):
print("Both variables are equal")
else:
print("Both variables are not equal")
Output:
Both variables are equal
Case Insensitive String Comparison With the upper()
Method
The last session has introduced how to carry out the case insensitive string comparison using the lower()
method. The logic behind using the upper()
method is the same. We want to change the existing string variables into either uppercase or lowercase characters in both methods. The upper()
method is the string class’s built-in method to convert all the characters of a string variable to uppercase.
normal_str1 = "Hello World!"
upper_str1 = normal_str1.upper()
normal_str2 = "hello world!"
upper_str2 = normal_str2.upper()
if upper_str1 == upper_str2:
print("Both variables are equal")
else:
print("Both variables are not equal")
Output:
Both variables are equal
Case Insensitive String Comparison With the casefold()
Method
The casefold()
method is a more aggressive method for converting a string variable to lowercase characters. For example,
There is a German letter, 'ß'
that is already a lowercase letter. So, lower()
method does not do anything to 'ß'
. But casefold()
converts 'ß'
to "ss"
.
normal_str = "ß"
casefold_str = normal_str.casefold()
lower_str = normal_str.lower()
print("Case folded form of ß is : " + casefold_str)
print("Lower cased form of ß is : " + lower_str)
Output:
Case folded form of ß is : ss
Lower cased form of ß is : ß
The casefold()
method returns a string variable in which all the characters are aggressively converted into lowercase. This new string variable can then be compared to carry out a case insensitive comparison.
normal_str1 = "Hello World ß!"
casefold_str1 = normal_str1.casefold()
normal_str2 = "Hello World ss!"
casefold_str2 = normal_str2.casefold()
if casefold_str1 == casefold_str2:
print("Both variables are equal")
else:
print("Both variables are not equal")
Output:
Both variables are equal
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn