Python 中不区分大小写的字符串比较
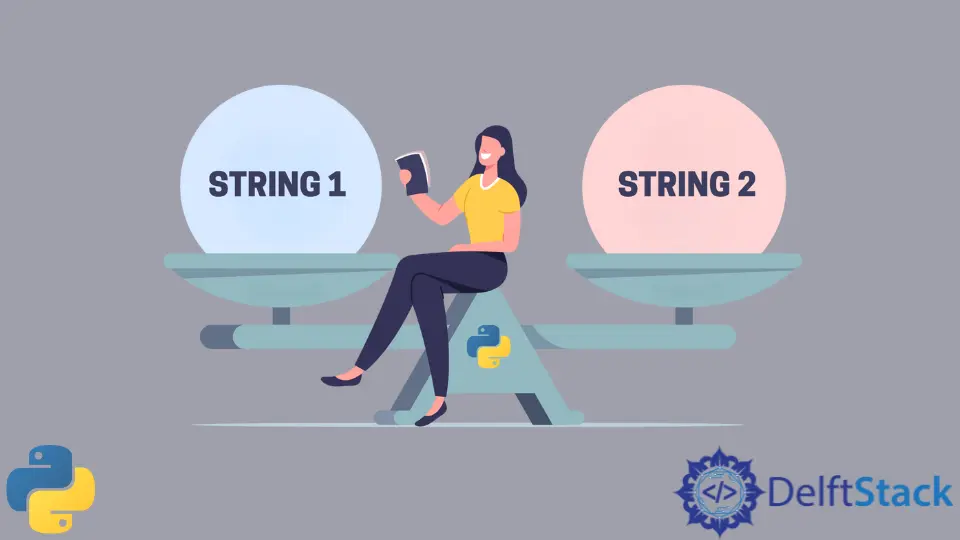
本教程将讨论在 Python 中对两个或多个字符串变量进行不区分大小写比较的一些方法。
使用 lower()
方法进行不区分大小写的字符串比较
Python 字符串有一个内置的 lower()
方法,可以将字符串中的所有字符转换为小写字母。它返回一个将所有字符转换为小写字母的字符串。我们可以使用 lower()
方法将两个字符串转换为小写,然后对它们进行不区分大小写的比较。
normal_str1 = "Hello World!"
lower_str1 = normal_str1.lower()
print(lower_str1)
输出:
hello world!
现在对第二个字符串变量 normal_str2
做同样的操作。
normal_str2 = "HELLO WORLD!"
lower_str2 = normal_str2.lower()
print(lower_str2)
输出:
hello world!
正如我们所看到的,两个字符串都被转换为所有小写字符。下一步是比较两个字符串变量并显示输出。
normal_str1 = "Hello World!"
lower_str1 = normal_str1.lower()
normal_str2 = "HELLO WORLD!"
lower_str2 = normal_str2.lower()
if lower_str1 == lower_str2:
print("Both variables are equal")
else:
print("Both variables are not equal")
输出:
Both variables are equal
使用 upper()
方法进行不区分大小写的字符串比较
上节课介绍了如何使用 lower()
方法进行不区分大小写的字符串比较。使用 upper()
方法的逻辑是一样的。我们要在这两种方法中把现有的字符串变量改成大写或小写字符。upper()
方法是字符串类的内置方法,可以将一个字符串变量的所有字符转换为大写字母。
normal_str1 = "Hello World!"
upper_str1 = normal_str1.upper()
normal_str2 = "hello world!"
upper_str2 = normal_str2.upper()
if upper_str1 == upper_str2:
print("Both variables are equal")
else:
print("Both variables are not equal")
输出:
Both variables are equal
使用 casefold()
方法进行不区分大小写的字符串比较
casefold()
方法是一种更激进的方法,用于将字符串变量转换为小写字符。例如:
有一个德文字母'ß'
已经是小写字母了,所以 lower()
方法对'ß'
没有任何作用。因此,lower()
方法对'ß'
没有任何作用。但是 casefold()
可以将'ß'
转换为"ss"
。
normal_str = "ß"
casefold_str = normal_str.casefold()
lower_str = normal_str.lower()
print("Case folded form of ß is : " + casefold_str)
print("Lower cased form of ß is : " + lower_str)
输出:
Case folded form of ß is : ss
Lower cased form of ß is : ß
casefold()
方法返回一个字符串变量,在这个变量中,所有的字符都被积极地转换为小写。然后,这个新的字符串变量可以进行比较,进行不区分大小写的比较。
normal_str1 = "Hello World ß!"
casefold_str1 = normal_str1.casefold()
normal_str2 = "Hello World ss!"
casefold_str2 = normal_str2.casefold()
if casefold_str1 == casefold_str2:
print("Both variables are equal")
else:
print("Both variables are not equal")
输出:
Both variables are equal
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn