How to Insert String Into a String in Python
- Inserting a String into Another String Using String Concatenation
-
Inserting a String into Another String Using
str.format()
- Inserting a String into Another String Using F-strings
-
Inserting a String into Another String Using
str.replace()
-
Inserting a String into Another String Using
join()
- Conclusion
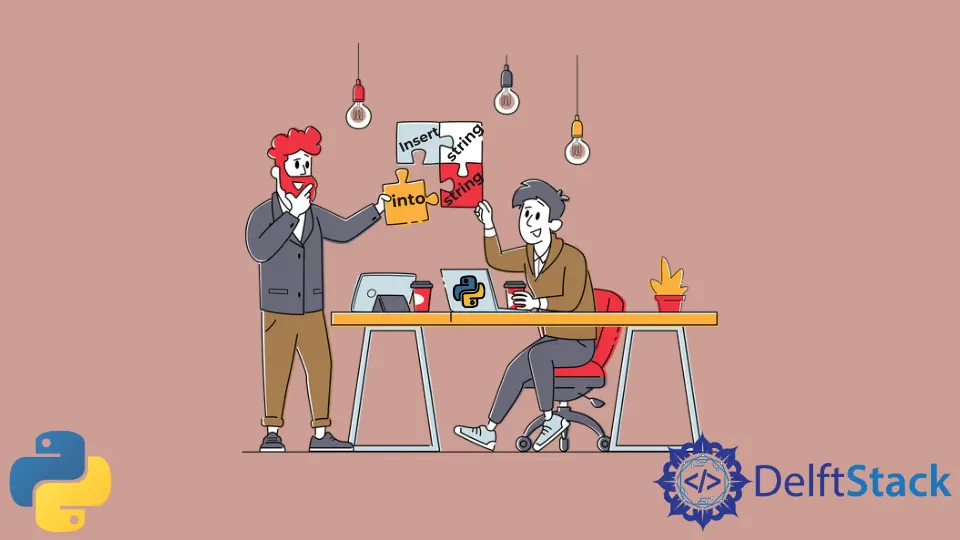
Inserting a string into another string is a common task in Python programming, essential for tasks like modifying messages, crafting personalized content, or organizing data efficiently. This fundamental operation is a key skill for Python developers, as it enables dynamic text manipulation for various applications.
Python offers various methods to accomplish this, and our guide will take you through these techniques step by step. Our objective is to equip Python developers with the necessary expertise to adeptly navigate different scenarios that require string insertion.
Inserting a String into Another String Using String Concatenation
String concatenation involves combining multiple strings into one, and it provides a straightforward way to insert a string into another at a specified position.
Let’s begin by presenting the complete working code that demonstrates how to insert a string into another using string concatenation.
# Original string
original_string = "Hello, what are doing?"
# String to insert
inserted_string = "you"
# Index to insert the string
index_to_insert = 16
# Using string concatenation to insert the string
result = (
original_string[:index_to_insert]
+ inserted_string
+ " "
+ original_string[index_to_insert:]
)
# Display the result
print(result)
Step-by-Step Code Explanation:
-
Original String Setup:
original_string = "Hello, what are doing?"
We start with an original string that serves as the base string into which we want to insert another string.
-
String to Insert:
inserted_string = "you"
Define the string that you want to insert into the original string.
-
Index to Insert:
index_to_insert = 16
Determine the index at which you want to insert the new string. In this example, we chose the index 16.
-
String Concatenation:
result = ( original_string[:index_to_insert] + inserted_string + " " + original_string[index_to_insert:] )
Use string concatenation to combine four parts:
- The substring of the original string before the insertion point (
original_string[:index_to_insert]
). - The string to be inserted (
inserted_string
). - The add some space (
" "
). - The substring of the original string after the insertion point (
original_string[index_to_insert:]
).
- The substring of the original string before the insertion point (
-
Display the Result:
print(result)
Finally, print the result to see the modified string with the inserted substring.
Output:
Hello, what are you doing?
String concatenation provides a simple and effective way to insert a string into another string in Python. Understanding the mechanics of string concatenation, as demonstrated in this article, empowers developers to manipulate strings efficiently. While other methods exist, such as using f-strings, str.format()
, or methods like str.replace()
, string concatenation remains a versatile and widely used approach for string manipulation in Python.
Inserting a String into Another String Using str.format()
This approach offers readability, flexibility, and ease of use, making it a powerful tool for string interpolation and manipulation.
str.format()
Method Syntax
The basic syntax of the str.format()
method is as follows:
"Template string with {} placeholders".format(values)
Here, {}
acts as a placeholder within the string where you want to insert a value.
The .format()
method takes arguments (values), which are then inserted into these placeholders in order.
Let’s kick things off with a complete working code example that showcases how to leverage str.format()
to insert a string into another.
# Original string
original_string = "Hello, what are doing?"
# String to insert
inserted_string = "you"
# Using str.format() to insert the string
result = "{}{}{}".format(original_string[:16], inserted_string, original_string[16:])
# Display the result
print(result)
Step-by-Step Code Explanation:
-
Using
str.format()
:result = "{}{}{}".format(original_string[:16], inserted_string, original_string[16:])
Employ the
str.format()
method, which provides a clean and readable way to format strings. In this case, the curly braces{}
act as placeholders for the values to be inserted. -
Placeholders in
str.format()
:{}
: The first set of curly braces represents the placeholder for the substring of the original string before the insertion point (original_string[:16]
).{}
: The second set of curly braces is the placeholder for the string to be inserted (inserted_string
).{}
: The third set of curly braces serves as the placeholder for the substring of the original string after the insertion point (original_string[16:]
).
Leveraging str.format()
for string insertion in Python enhances code readability and maintainability. The use of placeholders provides a clear structure, making it easy to understand the intended modifications to the original string. As Python developers, mastering this technique adds a valuable tool to your string manipulation arsenal. While alternative methods exist, such as string concatenation or f-strings, understanding and utilizing str.format()
empowers you to write cleaner and more expressive code.
Inserting a String into Another String Using F-strings
String manipulation is a common task in Python programming, and F-strings (formatted string literals
) offer a concise and expressive way to achieve this. In this comprehensive guide, we will explore how to insert a string into another using the powerful F-string feature in Python.
Python F-strings, introduced in Python 3.6, revolutionized how string formatting is handled, making it more straightforward and readable. The primary characteristic of F-strings is their simple and intuitive syntax. They are marked by an f
or F
preceding the string, and within these strings, curly braces {}
are used to enclose variables or expressions. This syntax is a significant departure from the more verbose and less intuitive methods like .format()
or the %
operator.
Another notable feature of F-strings is the ability to perform inline expression evaluation. This means you can insert any valid Python expression inside the curly braces, and it will be evaluated at runtime. This direct incorporation of expressions within the string simplifies many operations that would otherwise require additional steps, such as concatenation or variable formatting. It’s a feature that not only saves time but also enhances the clarity of the code by keeping related information together.
Let’s start with a complete working code example that demonstrates how to use F-strings for string insertion:
# Original string
original_string = "Hello, what are doing?"
# String to insert
inserted_string = "you "
# Using F-string to insert the string
result = f"{original_string[:16]}{inserted_string}{original_string[16:]}"
# Display the result
print(result)
Step-by-Step Code Explanation:
-
Using F-string for String Insertion:
result = f"{original_string[:16]}{inserted_string}{original_string[16:]}"
Utilize the F-string format, denoted by the
f
prefix, to create a formatted string. Inside the curly braces{}
, you can include expressions that will be evaluated at runtime. -
F-string Expression Parts:
{original_string[:16]}
: Represents the substring of the original string before the insertion point.{inserted_string}
: Represents the string to be inserted.{original_string[16:]}
: Represents the substring of the original string after the insertion point.
F-strings provide a concise and readable way to insert a string into another string in Python. With their expressive syntax and ability to include expressions, F-strings make string manipulation more intuitive and elegant.
Inserting a String into Another String Using str.replace()
The str.replace()
method provides a powerful way to insert a substring into another string. In this comprehensive guide, we’ll explore how to use str.replace()
for string insertion, understanding its nuances and versatility.
str.replace()
Method Syntax
The syntax for Python’s str.replace()
method is as follows:
str.replace(old, new[, count])
old
is the substring you want to replace.new
is the substring that will replace theold
substring.count
is an optional argument that specifies the maximum number of occurrences to replace. If omitted, all occurrences of theold
substring will be replaced.
Let’s start with a complete working code example that demonstrates how to utilize str.replace()
to insert a string into another:
# Original string
original_string = "Hello, what are doing?"
# String to insert
inserted_string = "you"
# Using str.replace() to insert the string
result = original_string.replace("are", f"are {inserted_string}")
# Display the result
print(result)
Step-by-Step Code Explanation:
-
Using
str.replace()
for String Insertion:result = original_string.replace("are", f"are {inserted_string}")
Employ the
str.replace()
method, which replaces a specified substring with another substring. In this case, we are replacing the substringare
with the concatenated stringare you
. -
Parameters of
str.replace()
:"are"
: The substring to be replaced.f"are {inserted_string}"
: The substring to insert in place of the original substring.
The str.replace()
method offers a straightforward and efficient way to insert a string into another string in Python. This method is particularly useful when you want to replace a specific substring with another, making it a versatile solution for a variety of string manipulation tasks.
Inserting a String into Another String Using join()
The join()
method provides an elegant solution for inserting a string into another. In this comprehensive guide, we will explore the intricacies of using join()
to perform string insertion and understand its versatility in Python programming.
join()
Function Syntax
separator.join(iterable)
separator
specifies the string that will be placed between the elements of the iterable during concatenation.iterable
is a collection of strings, such as a list or tuple, which will be joined together.
This method returns a new string, which is the concatenation of the strings in the iterable, separated by the specified separator
.
Let’s start with a complete working code example that demonstrates how to use join()
to insert a string into another:
# Original string
original_string = "Hello, what are doing?"
# String to insert
inserted_string = "you "
# Using join() to insert the string
result = "".join([original_string[:16], inserted_string, original_string[16:]])
# Display the result
print(result)
Step-by-Step Code Explanation:
-
Using
join()
for String Insertion:result = "".join([original_string[:16], inserted_string, original_string[16:]])
Utilize the
join()
method to concatenate a list of strings. In this case, the list consists of three elements:- The substring of the original string before the insertion point (
original_string[:16]
). - The string to be inserted (
inserted_string
). - The substring of the original string after the insertion point (
original_string[16:]
).
- The substring of the original string before the insertion point (
-
List Elements in
join()
:original_string[:16]
: Represents the substring of the original string before the insertion point.inserted_string
: Represents the string to be inserted.original_string[16:]
: Represents the substring of the original string after the insertion point.
The join()
method in Python provides a flexible and readable approach to insert a string into another string. While commonly used for joining elements of a list, its application for string insertion showcases its versatility.
Conclusion
In conclusion, this comprehensive guide has explored various methods to insert a string into another string in Python, each offering unique benefits and suitable for different scenarios in Python programming.
String concatenation stands out for its simplicity and direct approach, allowing developers to insert a string at a specified index easily. The str.format()
method, with its readability and flexible placeholder system, offers a more structured way of inserting strings, especially useful in scenarios requiring dynamic string construction.
F-strings, with their concise syntax and ability to perform inline evaluations, represent a significant advancement in Python’s string formatting capabilities. They offer an intuitive and efficient way to embed expressions directly within string literals, enhancing code clarity and simplicity.
The str.replace()
method, on the other hand, excels in scenarios where a specific substring needs to be replaced with another, making it a potent tool for both simple and complex string manipulations.
Finally, the join()
method, typically known for its use in concatenating sequences, also finds a unique application in string insertion, showcasing its flexibility and the depth of Python’s string manipulation capabilities.
Each method, be it string concatenation, str.format()
, F-strings, str.replace()
, or join()
, adds a valuable dimension to a Python developer’s toolkit, enabling efficient and expressive string manipulation. Understanding these techniques and their appropriate use cases is crucial for any Python programmer looking to adeptly handle various string-related tasks.