Static Class Variables in Python
- Understanding Static Class Variables
- Accessing Static Class Variables
- Modifying Static Class Variables
- Best Practices for Using Static Class Variables
- Conclusion
- FAQ
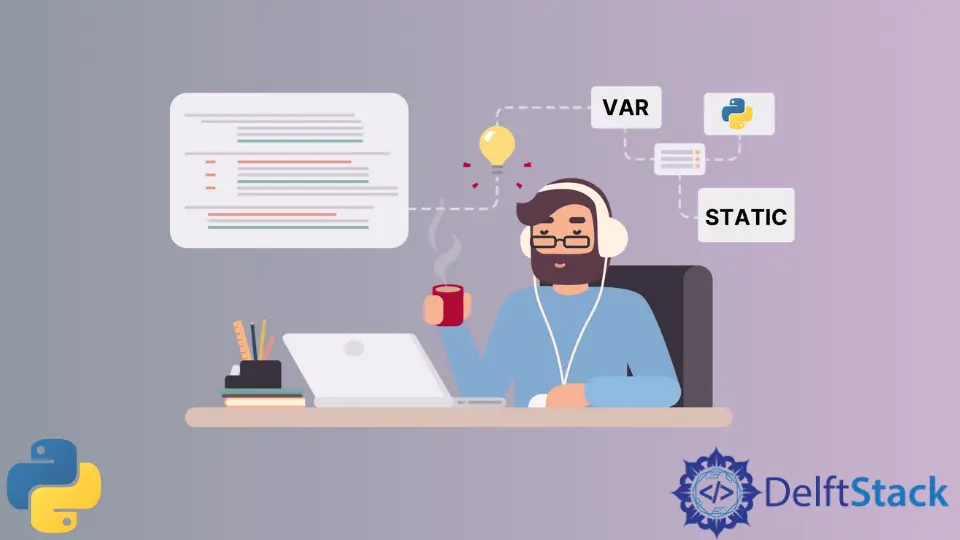
Static class variables in Python are an essential concept for developers looking to manage data shared across instances of a class. Unlike instance variables, which are tied to specific instances of a class, static class variables are shared among all instances.
This tutorial will guide you through the process of defining and using static class variables in Python, enhancing your understanding of class-level data management. Whether you’re a novice or an experienced programmer, grasping this concept will help you write more efficient and organized code. Let’s dive into the world of static class variables and explore how they can optimize your programming practices.
Understanding Static Class Variables
In Python, a static class variable is defined within a class but outside any instance methods. This means that it is not tied to a specific instance of the class, allowing all instances to access the same variable. Static variables are useful for storing data that should be consistent across all instances, such as configuration settings or shared resources.
To define a static class variable, you simply assign a value to a variable within the class body. Here’s a basic example to illustrate this concept:
class MyClass:
static_var = 0
def __init__(self, value):
MyClass.static_var += value
instance1 = MyClass(5)
instance2 = MyClass(10)
print(MyClass.static_var)
Output:
15
In this example, static_var
is a static class variable initialized to zero. Each time an instance of MyClass
is created, the __init__
method adds the provided value to static_var
. As a result, when we create instance1
with a value of 5 and instance2
with a value of 10, the total of static_var
becomes 15. This illustrates how static class variables can maintain a cumulative state across multiple instances.
Accessing Static Class Variables
Accessing static class variables is straightforward. You can refer to them using the class name or through an instance of the class. However, it’s generally recommended to use the class name for clarity. This practice helps convey to anyone reading your code that the variable is shared across all instances.
Here’s how you can access static class variables:
class Counter:
count = 0
def __init__(self):
Counter.count += 1
counter1 = Counter()
counter2 = Counter()
print(Counter.count)
print(counter1.count)
print(counter2.count)
Output:
2
2
2
In this example, the Counter
class has a static variable count
that tracks the number of instances created. Each time a new instance is initialized, count
is incremented. When we print Counter.count
, counter1.count
, and counter2.count
, all yield the same result: 2. This demonstrates how static class variables maintain a consistent value across instances and can be accessed in a clear and efficient manner.
Modifying Static Class Variables
Modifying static class variables is a common requirement in many applications. You can change the value of a static variable directly using the class name or through an instance. However, modifying it through an instance can sometimes lead to confusion, as it may seem like you are changing an instance variable.
Here’s an example that showcases how to modify static class variables:
class Configuration:
setting = "default"
@classmethod
def update_setting(cls, new_setting):
cls.setting = new_setting
print(Configuration.setting)
Configuration.update_setting("new_value")
print(Configuration.setting)
Output:
default
new_value
Initially, the Configuration
class has a static variable setting
with a value of “default”. The update_setting
class method allows us to change the value of setting
. After calling this method with “new_value”, the output reflects the updated value. This highlights the flexibility of static class variables and the importance of using class methods for modifications to ensure clarity and maintainability in your code.
Best Practices for Using Static Class Variables
While static class variables can be incredibly useful, there are some best practices to keep in mind to ensure your code remains clean and understandable. First, always use descriptive names for static variables to convey their purpose clearly. This practice will help other developers (or even yourself in the future) understand the code’s intent at a glance.
Additionally, consider using class methods for any operations that modify static variables. This approach helps maintain a clear structure and avoids potential confusion about whether a variable is static or instance-specific. Lastly, document the purpose of your static variables within the class to provide context for anyone who might work with your code later.
Here’s an example that combines these best practices:
class UserRegistry:
active_users = 0
@classmethod
def register_user(cls):
cls.active_users += 1
@classmethod
def get_active_users(cls):
return cls.active_users
UserRegistry.register_user()
UserRegistry.register_user()
print(UserRegistry.get_active_users())
Output:
2
In this example, the UserRegistry
class keeps track of the number of active users through the static variable active_users
. By using class methods to manipulate this variable, we maintain clarity and enhance the maintainability of the code. Following these best practices will help you leverage the power of static class variables effectively.
Conclusion
In summary, static class variables in Python provide a powerful way to manage shared data across multiple instances of a class. Understanding how to define, access, and modify these variables is crucial for writing efficient and organized code. By following best practices, such as using descriptive names and class methods for modifications, you can ensure your code remains clear and maintainable. Whether you are building a small application or a large system, mastering static class variables will enhance your programming skills and improve your overall code quality.
FAQ
-
What are static class variables in Python?
Static class variables are variables defined within a class but outside any instance methods. They are shared across all instances of the class. -
How do I define a static class variable?
You define a static class variable by assigning a value to a variable within the class body, outside of any methods. -
Can I access static class variables through an instance?
Yes, you can access static class variables through an instance, but it is recommended to use the class name for clarity. -
How do I modify a static class variable?
You can modify a static class variable using the class name or through a class method. Using class methods is a clearer approach. -
What are the best practices for using static class variables?
Use descriptive names, employ class methods for modifications, and document the purpose of static variables to maintain clarity and code quality.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn