How to Sort List by Another List in Python
-
Use the
zip()
andsorted()
Functions to Sort the List Based on Another List in Python -
Use NumPy’s
argsort()
to Sort the List Based on Another List in Python -
Use the
more_itertools.sort_together
Function to Sort the List Based on Another List in Python -
Use
zip()
andoperator.itemgetter()
to Sort the List Based on Another List in Python -
Use the
pandas
Library to Sort the List Based on Another List in Python -
Use
OrderedDict()
to Sort the List Based on Another List in Python - Conclusion
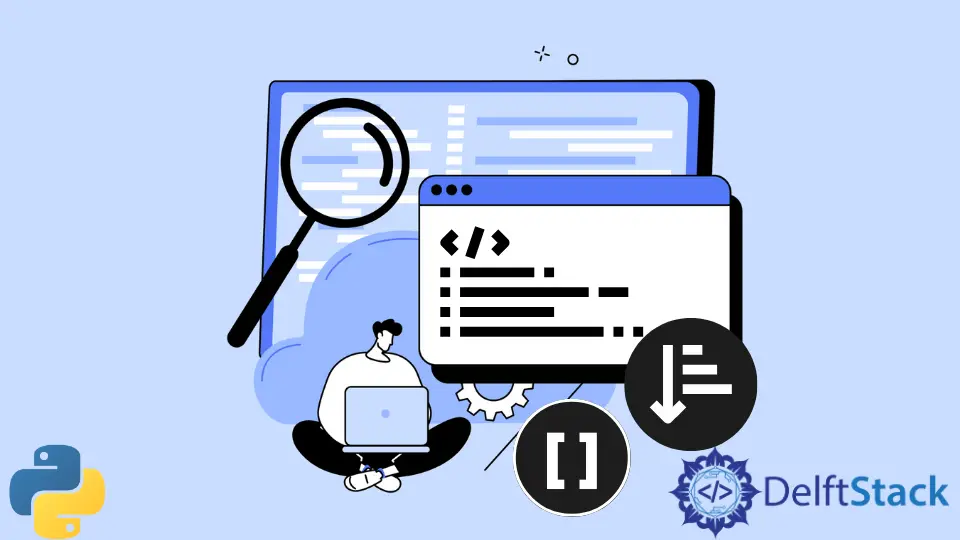
In Python, situations may arise where it becomes essential to sort one list based on the values of another list.
This article will explore various approaches to achieving this task, providing a detailed walkthrough of each method.
Use the zip()
and sorted()
Functions to Sort the List Based on Another List in Python
One way to sort one list based on the values of another list involves using [the zip()
function]({{relref “/HowTo/Python/zip python 3.en.md”}}) to pair corresponding elements from both lists and then applying the sorted()
function with a custom key.
The zip()
function in Python combines elements from multiple iterables into tuples. In the context of sorting lists, zip()
helps create pairs of elements from the two lists, allowing us to sort one list based on the values of the other.
The sorted()
function then takes these pairs and sorts them based on a specified key.
Let’s consider two lists, list1
and list2
. We want to sort list2
based on the values of list1
.
list1 = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
list2 = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k"]
combined_lists = list(zip(list1, list2))
sorted_lists = sorted(combined_lists, key=lambda x: x[0])
sorted_list2 = [item[1] for item in sorted_lists]
print(sorted_list2)
In the provided Python code, first, we utilize zip(list1, list2)
to create pairs of corresponding elements from list1
and list2
, forming a new list named combined_lists
. This step essentially aligns the elements from both lists in pairs.
Next, we apply the sorted()
function to combined_lists
with the key
parameter set to a lambda function (lambda x: x[0]
). This lambda function serves as the sorting key, indicating that the sorting should be based on the first element of each tuple in combined_lists
.
As a result, sorted_lists
now contains the pairs from combined_lists
sorted according to the values of list1
.
To obtain the final sorted list, we use a list comprehension ([item[1] for item in sorted_lists]
). This expression iterates over the tuples in sorted_lists
and extracts the second element of each tuple, representing the values from list2
.
The result is stored in the variable sorted_list2
. The concluding step involves printing the sorted list (print(sorted_list2)
), displaying the elements of list2
in an order determined by the values in list1
.
Output:
['b', 'd', 'g', 'a', 'j', 'c', 'e', 'i', 'k', 'h', 'f']
In this example, list2
has been sorted based on the values of list1
.
Use NumPy’s argsort()
to Sort the List Based on Another List in Python
Another method we can use to sort a list based on another list is by using NumPy’s argsort()
function. This function returns the indices that would sort an array, which can be used to sort one list based on the values of another.
The key idea is to use the sorting indices obtained from argsort()
to rearrange the elements of the second list.
Consider two lists, list1
and list2
. Our goal is to sort list2
based on the values of list1
.
import numpy as np
list1 = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
list2 = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k"]
indices = np.argsort(list1)
sorted_list2 = [list2[i] for i in indices]
print(sorted_list2)
In this code snippet, we use NumPy’s argsort()
to obtain the indices that would sort list1
. The obtained indices are then utilized to rearrange the elements of list2
, resulting in sorted_list2
.
The line indices = np.argsort(list1)
calculates the sorting indices for list1
. These indices represent the order in which the elements of list1
would be sorted.
The list comprehension [list2[i] for i in indices]
constructs sorted_list2
by iterating over the sorted indices and retrieving the corresponding elements from list2
. The final sorted list is then printed using print(sorted_list2)
.
Output:
['b', 'd', 'g', 'a', 'j', 'c', 'e', 'i', 'k', 'h', 'f']
Use the more_itertools.sort_together
Function to Sort the List Based on Another List in Python
The more_itertools
library provides a convenient function called sort_together
that can also be used to sort a list based on the values from another list in Python.
The more_itertools.sort_together
function is designed to sort multiple iterables together. It takes one primary iterable, usually a list, and sorts it.
The key feature is that it rearranges the other iterables in the same order as the primary iterable, effectively ensuring that corresponding elements across multiple iterables remain synchronized after sorting.
Take a look at the code example below:
from more_itertools import sort_together
names = ["Alice", "Bob", "Charlie", "David"]
ages = [25, 30, 22, 35]
sorted_names, sorted_ages = sort_together([ages, names])
print("Sorted Names:", sorted_names)
print("Sorted Ages:", sorted_ages)
In this example, we have two lists: names
and ages
. We want to sort the names
list based on the values in the ages
list.
The sort_together
function is called with a list containing both ages
and names
. It sorts the primary list (ages
) and ensures that the order of elements in the other lists (names
in this case) remains consistent.
After sorting, the sorted names and ages are assigned to sorted_names
and sorted_ages
, respectively. The sorted lists are then printed to the console.
Output:
Sorted Names: (22, 25, 30, 35)
Sorted Ages: ('Charlie', 'Alice', 'Bob', 'David')
In this output, notice that the names
list is now sorted alphabetically based on the corresponding ages from the ages
list.
more_itertools.sort_together
is a useful tool for sorting multiple lists concurrently. It’s particularly useful when dealing with complex data structures where synchronization is crucial.
Use zip()
and operator.itemgetter()
to Sort the List Based on Another List in Python
Another concise and efficient way to sort a list based on another list in Python is by using the zip()
function and the operator.itemgetter()
method. This approach allows us to create pairs of elements from different lists and sort them based on the values of one of the lists.
The zip()
function is used to combine two lists element-wise, creating tuples of corresponding elements. To sort the first list based on the values of the second list, we employ operator.itemgetter()
as the key function in the sorted()
function.
This allows us to specify the index of the element to be used for sorting within the tuples created by zip()
.
Consider the code example provided below:
from operator import itemgetter
def sort_list_by_values(main_list, values_list):
combined = zip(values_list, main_list)
sorted_list = sorted(combined, key=itemgetter(0))
result = list(map(itemgetter(1), sorted_list))
return result
main_list = ["apple", "banana", "orange", "grape"]
values_list = [3, 1, 4, 2]
sorted_main_list = sort_list_by_values(main_list, values_list)
print("Sorted List:", sorted_main_list)
The sort_list_by_values
function takes two lists as arguments: main_list
and values_list
. Using zip()
, it combines the two lists into tuples, pairing corresponding elements. The resulting tuples consist of (value from values_list
, corresponding element from main_list
).
Next, the sorted()
function is applied to sort the tuples based on the values from values_list
. The itemgetter(0)
specifies that sorting should be done based on the first element of each tuple, which is the value from values_list
.
Finally, the sorted tuples are converted back to a list of the corresponding elements from main_list
using map()
and itemgetter(1)
. The sorted and reordered list is then returned.
Output:
Sorted List: ['banana', 'grape', 'apple', 'orange']
Use the pandas
Library to Sort the List Based on Another List in Python
We can also use the pandas
library to sort a list based on the values from another list in Python. It provides a DataFrame
object, a two-dimensional labeled data structure, where we can store and manipulate our data.
To sort a list based on values from another list, we can create a DataFrame
with the two lists as columns and then use the sort_values()
method. This method allows us to specify a column as the key for sorting, effectively achieving the desired outcome.
Consider two lists, list1
and list2
, where we want to sort list1
based on the corresponding values in list2
. The following example demonstrates how to accomplish this using pandas
:
import pandas as pd
list1 = [3, 1, 4, 1, 5, 9, 2, 6]
list2 = ["c", "a", "d", "a", "e", "i", "b", "f"]
df = pd.DataFrame({"list1": list1, "list2": list2})
sorted_df = df.sort_values(by="list2")
sorted_list1 = sorted_df["list1"].tolist()
print(sorted_list1)
In this example, we start by importing the pandas
library as pd
. We then create a DataFrame df
with columns list1
and list2
using the two lists.
The sort_values()
method is then applied to the DataFrame
, specifying list2
as the sorting key. This results in a DataFrame
, sorted_df
, where list1
is sorted based on the corresponding values in list2
.
Finally, we extract the sorted values from list1
and convert them to a list.
Output:
[1, 1, 2, 3, 4, 5, 6, 9]
This output represents the sorted values of list1
based on the corresponding values in list2
. The order is determined by the values in list2
in ascending order (alphabetical order in this case).
Use OrderedDict()
to Sort the List Based on Another List in Python
Sorting a list based on the values from another list in Python can also be achieved using the OrderedDict
class from the collections
module. OrderedDict
is a dictionary subclass that maintains the order of keys based on the order in which they are inserted.
The idea behind using OrderedDict
is to create a mapping between values from the second list (the one based on which we want to sort) and their corresponding indices from the first list. We then sort the items of the OrderedDict
based on the keys (values from the second list) and obtain the sorted indices.
Using these indices, we can construct the sorted list.
Consider the same lists, list1
and list2
, where we want to sort list1
based on the corresponding values in list2
.
from collections import OrderedDict
list1 = [3, 1, 4, 1, 5, 9, 2, 6]
list2 = ["c", "a", "d", "a", "e", "i", "b", "f"]
ordered_dict = OrderedDict(zip(list2, list1))
sorted_dict = OrderedDict(sorted(ordered_dict.items()))
sorted_list1 = list(sorted_dict.values())
print(sorted_list1)
In this example, we start by creating an OrderedDict
named ordered_dict
using the zip()
function to pair values from list2
with their corresponding values from list1
. Next, we use the sorted()
function on the items of the OrderedDict
, which returns a sorted list of tuples based on the keys (values from list2
).
Finally, we extract the sorted values from list1
by retrieving the values of the sorted items and converting them to a list.
Output:
[1, 2, 3, 4, 5, 6, 9]
Conclusion
Python offers a plethora of methods to sort one list based on the values of another list, each catering to specific needs and preferences.
Whether it’s through the zip()
function, the NumPy library, the more_itertools
package, operator.itemgetter()
, the pandas
library, or the OrderedDict
from the collections
module, Python provides an array of tools for developers to streamline the sorting process based on their unique requirements.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python