How to SendGrid in Python
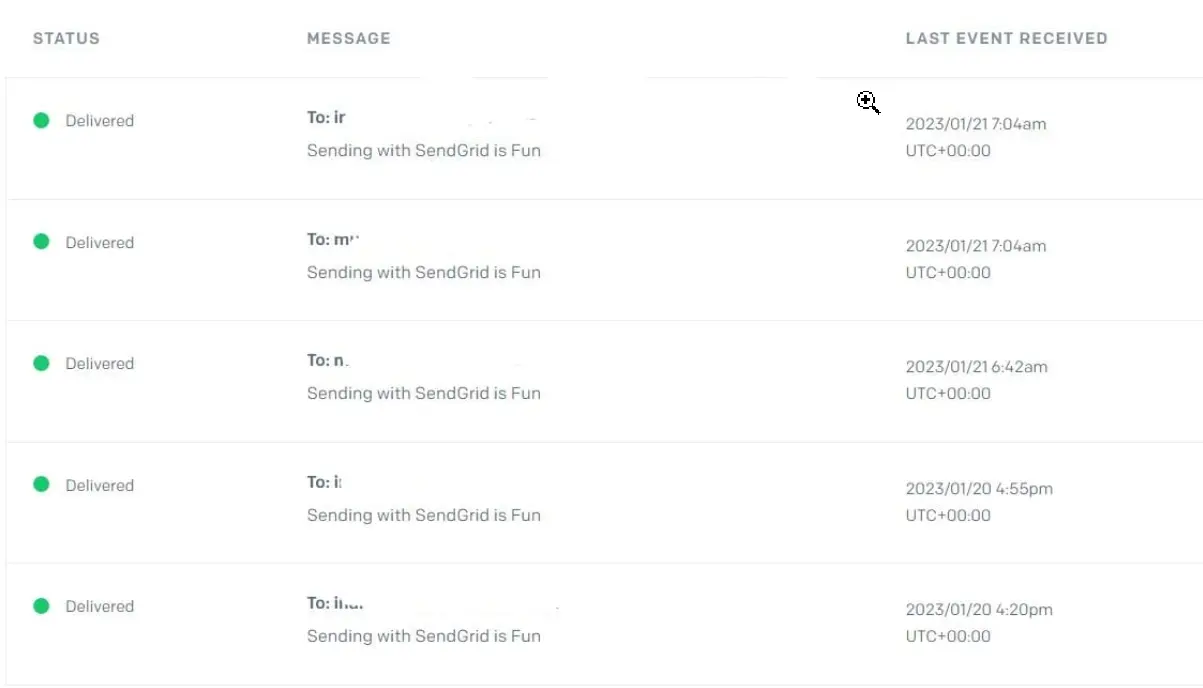
The parent organization for SendGrid
(a customer communication platform for transactional and marketing email) is Twilio
.
Mainly the task is to send emails in a dynamic form. This tends to minimize complexity and time consumption.
Initially, we will have to set up the personal detail to sign in, and then you will get a dashboard with multiple features (after your account is verified via email). You can send emails easily by going to the Marketing
section and then to the Sender
section.
The best experience is only achieved if you have a two-factor authenticated account. Otherwise, your account goes under a review phase, which might take a lot of time.
In the next section, we will point down the steps of handling the account creation detail. Follow up the steps one by one.
-
Go to
SendGrid
. -
Create your account.
-
You will be required to activate the
Two Factor Authentication
. -
Once an account has been created and verified, go to the
Settings
tab on the left navigation panel. -
Select the
API Keys
option from the drop-down menu. -
Click on the
Create API Key
tab to create a secretAPI Key
for your app. -
Set an
API Key Name
. -
Choose
Full Access
from the radio buttons. -
Lastly, click on
Create and View
.
Make sure you have saved the API key somewhere safe. Technically, it is shown explicitly viewed once.
In the next part, you will set up the sender email.
-
Navigate to
Marketing
and then clickSenders
. -
In the top right corner of the
Sender Management
page, clickCreate New Sender
. -
Fill in all of the fields on the page and then click
Save
.
There might be a confirmation mail sent to the mail for securing the mail you added as sender.
For authentication, you can use your contact number. If you are facing any problems, you can download Google Authy
and use the contact number you will use in Authy
.
This passive process might save you.
But in this phase, if you send an email, the sender will have to check the mail every time to confirm. The following coding section will show how we can use the API key to send emails to multiple receivers. You can learn more about this process in our article on How to Send Emails to Multiple Addresses Using Python.
Use the SendGrid
API Key to Send Multiple Emails
Initially, we will have to create a project folder on our local computer. Also, we will initiate a Python virtual environment.
You must open the terminal/cmd in that folder to create it. Enter the following commands.
python -m venv YourFolderName
cd YourFolderName
We will create a script file in this folder (folder name we used: sendgrid
). We created main.py
as our script file. Next, we will install the dependencies.
Before that, the virtual environment needs to be activated. So, in the sendgrid
folder, we will open the terminal and follow up on the commands.
Scripts\activate
pip install sendgrid
pip install python-dotenv
Now create a .env
file (the file to store the environment variable). And there, we will keep the following piece of code.
export API_KEY=YOUR_SENDGRID_API_KEY
Here, in the API_KEY
variable, we will keep the previously saved API key from the SendGrid
website. Next, we will go for the coding section.
Code Snippet:
import os
import dotenv
from sendgrid import SendGridAPIClient
from sendgrid.helpers.mail import Mail, Email, To, Content
dotenv.load_dotenv()
sgc = SendGridAPIClient(api_key=os.environ.get("API_KEY"))
from_email = Email("sender@example.com") # Change to your verified sender
# Change to your recipient
to_email = [To("receiver1@example.com"), To("receiver2@example.com")]
subject = "Sending with SendGrid is Fun"
content = Content("text/plain", "and easy to do anywhere, even with Python")
mail = Mail(from_email, to_email, subject, content, is_multiple=True)
# is_multiple is used to specify that the mail has multiple recipients
# Get a JSON-ready representation of the Mail object
mail_json = mail.get()
# Send an HTTP POST request to /mail/send
try:
response = sgc.client.mail.send.post(request_body=mail_json)
print(response.status_code)
print(response.headers)
except Exception as e:
print(e)
The dotenv
will import the API key as a dictionary, and after calling it, we will see the update in the website dashboard in every transmission. We have sent multiple emails in one run.
The output shows the preview we will have in the SendGrid
dashboard and activity feed.
Output: