How to Validate Email Address in Python
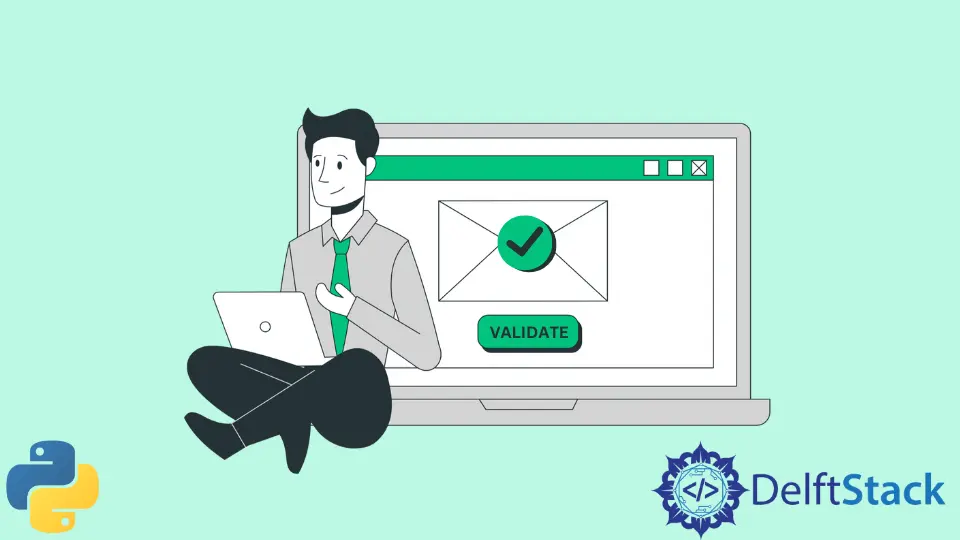
Email validation is an important part of the user journey within a closed application, and it is important, especially for security and reducing user churn. There are different methods to validate the email, and understanding the structure of the typical email address is important.
There are built-in features such as regex
and libraries such as dnspython
that help with these processes. This article will explain how to use these features and libraries to validate emails in Python.
Use regex
to Validate Email in Python
Regular Expression, popularly called regex
, is a sequence of characters that specifies a search pattern in the text and is helpful for search algorithms in strings and doing find
and find and replace
.
With regex
, we can find certain patterns within the email address we receive from the user. Every email address has the same pattern, [username]@[smtp server].com
.
We can use regex
to find the username
part and the SMTP server
part. To understand how to use regex
, you must know about metacharacter, special sequences, and sets.
Using this knowledge, we can create and compile a regex
pattern using the compile
method and use the match
method to compare the string of the user’s email address to verify it. In Python, to use regex
, we need the re
library, which supports the regular expression.
import re
user_email = input("Enter your Email Address: ")
pattern = re.compile(r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?")
if not re.match(pattern, user_email):
print(f"{user_email} is not a valid email address")
else:
print(f"Your {user_email} is a valid email address")
Output:
PS C:\Users\akinl\Documents\Python> python email.py
Enter your Email Address: akin@gm.com
Your akin@gm.com is a valid email address
PS C:\Users\akinl\Documents\Python> python email.py
Enter your Email Address: dx@s
dx@s is not a valid email address
The \"?([-a-zA-Z0-9.?{}]+@\w+\.\w+)\"?
string contains the regex pattern that we will compare the user’s email address to.
With the first input, akin@gm.com
, the email contains all the required patterns and structure to make a valid email from the username to the SMTP server. However, for dx@s
, the SMTP server is non-existent.
We can also check for a match using the fullmatch
method, which is most preferred with Python 3.x. The code is similar, but all we need to change is the match
method to fullmatch
.
The difference between match
and fullmatch
is that match
matches only at the beginning while fullmatch
matches all through the string text to the end.
import re
user_email = input("Enter your Email Address: ")
pattern = re.compile(r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?")
if not re.fullmatch(pattern, user_email):
print(f"{user_email} is not a valid email address")
else:
print(f"Your {user_email} is a valid email address")
Output:
PS C:\Users\akinl\Documents\Python> python email.py
Enter your Email Address: akin@gmail.com
Your akin@gmail.com is a valid email address
With this, we can check for a valid email structure, though we can’t check whether the SMTP server exists, which is equally important. That’s where the next feature comes in.
Use dnspython
to Validate Email in Python
With the dnspython
library, we can validate the SMTP part of the email to see if it is actual and not made up. After we check and validate the email, we can split the part of the SMTP server and check using the resolve
method.
Since dnspython
is not a built-in library, we need to install it via pip
.
pip install dnspython
After installing dnspython
, we can import it into our code and use the resolve
method to check for a valid SMTP server.
import dns.resolver
try:
answers = dns.resolver.resolve("google.com", "MX")
for rdata in answers:
print("Host", rdata.exchange, "has preference", rdata.preference)
print(bool(answers))
except dns.exception.DNSException as e:
print("Email Doesn't Exist")
Output:
Host smtp.google.com. has preference 10
True
To check the SMTP server, we pass the MX
attribute to the resolve
method to check the mail exchange
record, and the returned rdata
is useful to see the exchange
and preference
attribute values.
However, what is important is to check whether there are values within the answer
binding using the bool
function. If there are no values there, it means that the SMTP server
is not valid.
To make a comprehensive email validation check, we can combine the regex
and dnspython
codes.
import dns.resolver
import re
user_email = input("Enter your Email Address: ")
pattern = re.compile(r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?")
if not re.fullmatch(pattern, user_email):
print(f"{user_email} is not a valid email address.")
else:
domain = user_email.rsplit("@", 1)[-1]
try:
answers = dns.resolver.resolve(domain, "MX")
if bool(answers):
print(f"Your {user_email} is a valid email address.")
except dns.exception.DNSException as e:
print("Not a valid email server.")
Output:
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: akin@gmail.com
Your akin@gmail.com is a valid email address.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: ds.cem@fex.co
Your ds.cem@fex.co is a valid email address.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: wer@fghj.com
Your wer@fghj.com is a valid email address.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: df@werabsjaksa.com
Not a valid email server.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: des@python.exe
Not a valid email server.
The try/except
section helps to deal with certain exceptions when the SMTP server
doesn’t exist.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn