How to Send an Email With Attachments in Python
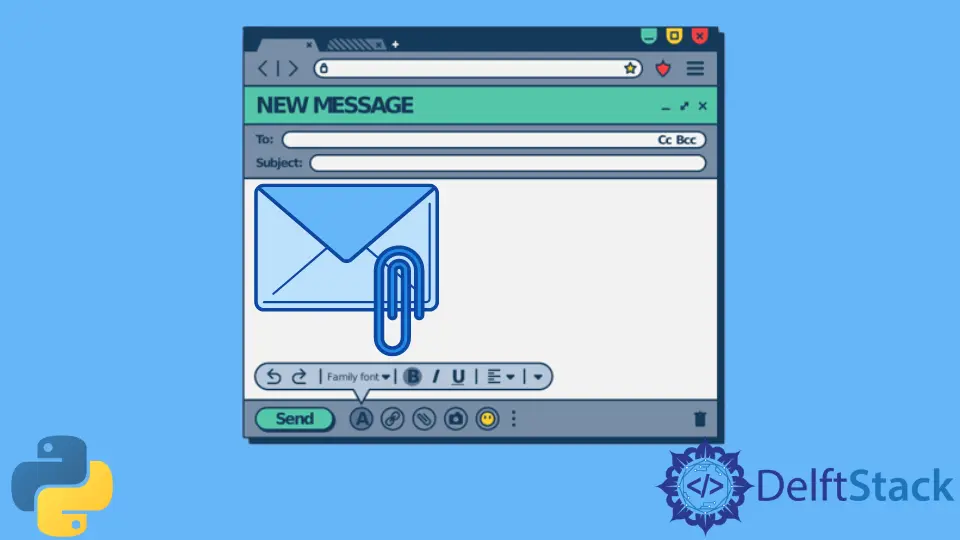
Today’s article teaches us how Python is used to send emails with attachments. For that, we don’t require an external library to send mail. Instead, we import a module named smtplib
and use it because the mail is sent through the SMTP
protocol. Let’s see how we can do it.
Send an Email With Attachments in Python
Python treats all message content as ordinary text when it sends a text message. Even if we add HTML tags in a text message, they won’t be structured following HTML syntax, and the message will still appear as a primary text.
However, Python can send an HTML message in its native format. Therefore, we can choose a MIME
version, content type, and character set when sending an email to send an HTML email.
Python includes a package referred to as smtplib
. The mail is sent via SMTP
(Simple Mail Transfer Protocol). For mailing, it produces SMTP
client session objects.
Correct (valid) source & destination email addresses, server addresses and port numbers are required for SMTP
. For instance, the port number for Google is 587
. Remember, the server address and port number will change based on what service we are using. For this article, we are using Google.
First, we must import the mail-sending module smtplib
. We also use the MIME
(Multipurpose Internet Mail Extension) module to increase adaptability.
We may save the sender, recipient, and other information using the MIME
header. The MIME
is also required to attach the file to the email. To send messages, we utilize Google’s Gmail service.
To comply with Google’s security requirements, we must make certain adjustments. For example, the following code may not function if such settings are missing or Google does not support access from third-party apps.
We must specify less secure app Access settings in the Google account to provide access. Further, we cannot use less secure access if two-step verification is enabled.
Steps to Send an Email With Attachments in Python
First, we have to create MIME
, then add sender, receiver, mail title and attached body into the created MIME
. After that, open the file attached to the email in binary mode.
For the attachments, add a header, open an SMTP
session with a working port number, 587
, and quit the session.
Example Code:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
mail_content = """Hello,
This mail is to inform you about Today's meeting.
"""
sender_address = "sender@gmail.com"
sender_pass = "-------"
receiver_address = "receiver@gmail.com"
message = MIMEMultipart()
message["From"] = sender_address
message["To"] = receiver_address
message["Subject"] = "Info about meeting."
message.attach(MIMEText(mail_content, "plain"))
session = smtplib.SMTP("smtp.gmail.com", 587)
session.starttls()
session.login(sender_address, sender_pass)
text = message.as_string()
session.sendmail(sender_address, receiver_address, text)
session.quit()
print("Mail Sent")
Output:
Mail Sent
Here is another example of how to send an email with attachments in Python.
Example Code:
import smtplib
from pathlib import Path
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email.mime.text import MIMEText
import email.utils
from email import encoders
from smtpd import COMMASPACE
def send_mail(
send_from,
send_to,
subject,
message,
files=[],
server="localhost",
port=587,
username="",
password="",
use_tls=True,
):
msg = MIMEMultipart()
msg["From"] = send_from
import email.utils
msg["To"] = COMMASPACE.join(send_to)
msg["Date"] = email.utils.formatdate(localtime=True)
msg["Subject"] = subject
msg.attach(MIMEText(message))
for path in files:
part = MIMEBase("application", "octet-stream")
with open(path, "rb") as file:
part.set_payload(file.read())
encoders.encode_base64(part)
part.add_header(
"Content-Disposition", "attachment; filename={}".format(Path(path).name)
)
msg.attach(part)
smtp = smtplib.SMTP(server, port)
if use_tls:
smtp.starttls()
smtp.login(username, password)
smtp.sendmail(send_from, send_to, msg.as_string())
smtp.quit()
Output:
/Users/apple/PycharmProjects/email/venv/bin/python /Users/apple/PycharmProjects/email/main.py
Process finished with exit code 0
Remember, the first step to sending email in Python is to make your Google account less secure; otherwise, it will generate a connection refused error
. Make sure that the two-step authentication (2FA) is removed to make it work.
Second, we must import the mailing sending Module, and valid source and destination email addresses and port numbers are required for SMTP
. For example, the port number for Google is 587
.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn