How to Send Emails to Multiple Addresses Using Python
- Send Emails to Multiple Addresses Using Python
-
the
smtplib
Module in Python -
Send an Email Using
smtplib
in Python - Control Access to Less Secure Apps
-
Send an Email Using
smtplib
in Python
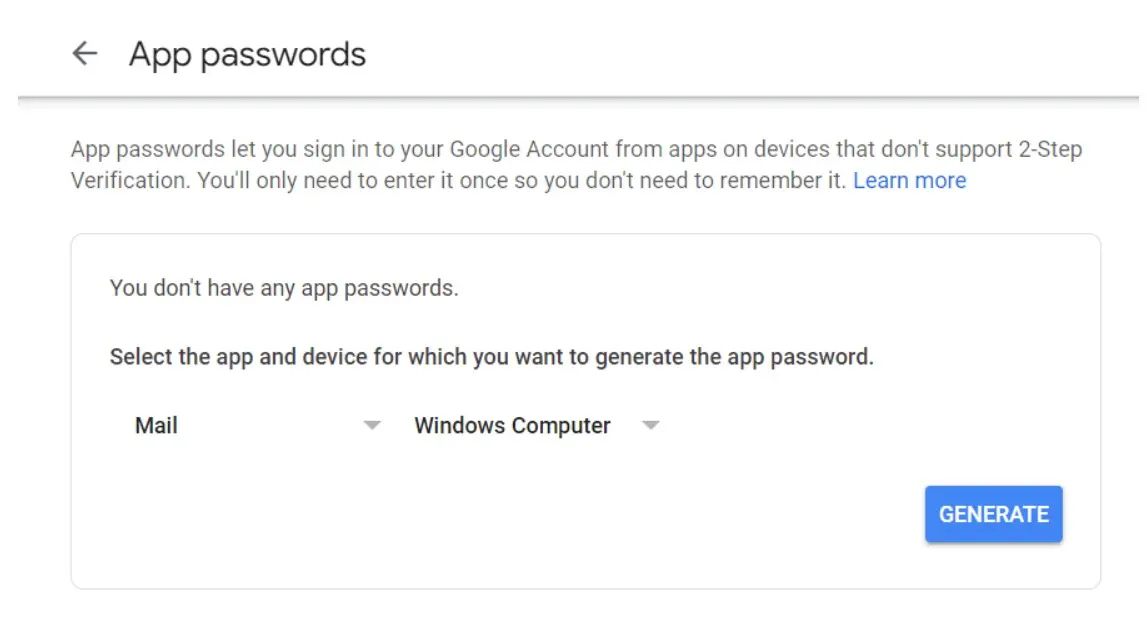
In this article, we will demonstrate how to use the Python programming language to send emails to various recipients using various email addresses. Specifically, we will be sending emails to many different people.
Send Emails to Multiple Addresses Using Python
Python is more than simply a programming language for building websites or working with Artificial Intelligence.
It is a language that can be used on the backend, front end, or the whole stack of a web application. Therefore its versatility makes it a helpful tool.
Python has now evolved into a language that can also be used to automate emails. Tasks that involve email can be easily automated using Python.
We need a few lines of code to use Python to send emails to several different recipients at the same time.
In this Python article, we will demonstrate how to use Python to send emails to several recipients simultaneously.
It is not very difficult to send emails using Python, particularly if you use a module such as smtplib
. This library will take care of all you need to know to connect to a mail server and deliver the message for you.
We will take you step-by-step through configuring a Python script so that it can send emails to many different addresses. In addition, we will include some example codes for you to use as we get started.
If we want to utilize smtplib
, you first need to establish a connection to the server. We will need to supply the server’s location and our login and password to access the file.
After establishing the connection, we can begin sending messages to one another.
To send a message, we will need first to build a message object and then supply the headers for the from
, to
, and topic fields. We can also add cc
and bcc
recipients.
After the message has been produced, we will have the option to add text, HTML, or attachments.
When we are ready to send the message, we can use the sendmail()
function included in the smtplib
module. This technique requires a list of receivers, then communicates with each individual on the list.
So let’s begin with smtplib
.
the smtplib
Module in Python
The smtplib
is a built-in module in Python. It has client session objects.
The smtplib
defines an SMTP
client session object. This object can be used to deliver email to any computer connected to the internet that is running an SMTP
or ESMTP
listener daemon.
Application programmers are not expected to use this module in their work directly. System administrators who are building scripts to automate the activities of system management will find it valuable to have access to this resource.
the smtplib
Module Classes
The smtplib
module defines the following classes:
SMTP
- An SMTP client session objectSMTP_SSL
- An SMTP client session over an SSL connectionIMAP4
- AnIMAP4
client session objectPOP3
- APOP3
client session object
All of these classes have the same methods and attributes.
the smtplib
Module Exceptions
The smtplib
module also defines the following exceptions:
SMTPException
- Base class for all SMTP-related exceptions.SMTPServerDisconnected
- The SMTP server disconnected unexpectedly.SMTPResponseException
- The SMTP server returned an error code other than2xx
.SMTPSenderRefused
- The SMTP server refused to accept the sender’s address.SMTPRecipientsRefused
- The SMTP server refused to accept all recipient addresses.SMTPDataError
- The SMTP server does not accept the message data.SMTPConnectError
- The SMTP server could not be connected.SMTPHeloError
- The SMTP server did not respond properly to theHELO
command.SMTPNotSupportedError
- The SMTP server does not support the requested feature.SMTPAuthenticationError
- The SMTP server did not accept the username/password.
Let’s move to the main topic of sending emails using Python.
Send an Email Using smtplib
in Python
It’s no secret that Google has been cracking down on Less Secure App
access to Gmail accounts. This has been a great source of frustration for many users, especially those who rely on Python to send emails through their Gmail accounts.
Unfortunately, Google no longer allows Less Secure App
access to Gmail accounts. If we want to use Python’s smtplib
module to send emails through our Gmail account, we will need to take some extra steps to ensure your account is secure.
So, the first thing we will do is control access to Less Secure Apps
.
Control Access to Less Secure Apps
As you may have heard, Google has stopped providing support for a feature known as Less Secure App
. This feature allowed you to access your Gmail account using a program like Microsoft Outlook, Thunderbird, or Apple Mail.
This may sound like pain; it’s a good thing, though! Less Secure Apps
are a major security risk, and by Google no longer supporting them, they’re helping to keep your account safe.
As in our case, we want Python’s smtplib
to access our Gmail, don’t worry! We don’t need to give our Gmail account password.
We can switch securely by following these simple steps:
-
Use a Secure Connection
Always use a secure connection when sending emails through your Gmail account. Gmail offers two different types of connections: SSL and TLS.
SSL (Secure Sockets Layer) is the older of the two protocols and is not as secure as TLS. However, it’s still more secure than an unencrypted connection.
TLS (Transport Layer Security) is a newer and more secure protocol than SSL. Gmail will automatically use TLS if it’s available.
-
Log in to Gmail Account
Before we send any email using the Python module
smtplib
, we must log into our account first because we need our email and a password generated from our Gmail account. -
Manage Account
Once we log into our account, we need to click on the icon of our Gmail profile. We will see a drop-down and an option with
[Manage your Google Account]
.We will have to click on it to move to the next step.
-
Security
After clicking on
[Manage your Google Account]
, we will be redirected to the next page where we can find theSecurity
option. We will have to click on it. -
Signing in to Google
After we click on
Security
, we will be redirected to another part of Gmail where we can find theSigning in to Google
option.The image below shows us that
Signing in to Google
has two options.-
2-Step verification
-
App Password
-
-
Enable
2-step Verification
And another important security measure is to enable two-factor authentication for your Gmail account. Two-factor authentication adds an extra layer of security by requiring you to enter a code from your phone and your password.
Now we will have to turn on the
2-step Verification
first. Which is very easy; you can do it within a few seconds. -
App Passwords
After we are done with 2 step verification, we will have to go with
App Passwords
to generate a password from our Gmail account.When we click on it, we will be redirected to the below-mentioned page.
Now we have to check options in Select App
and Select Device
.
In our case, we will select Mail
and Windows Computer
or any other device to move further and get a password. After this, we will click on the Generate
button.
Once we click the Generate
button, we will be redirected to another page where we can see Google has generated a password for our email, which we can use while using Python’s smtplib
to send emails.
Now that we have securely generated a password for our email. We can now move to Python to send emails using smtplib
.
Send an Email Using smtplib
in Python
The smtplib
module makes it easy to send emails using Python. There are just a few steps you need to take to send an email using smtplib
.
First, we need to create an SMTP object. Next, we need to connect to the server using the connect()
method.
And finally, we can send an email using the msg.set_content
method. Let’s dive deep into the steps of code.
-
Import Library
We will first import
smtplib
. This is the protocol, and this protocol helps in sending the email. -
Email Format
We use
email.message import EmailMessage
to use email format in Python. LikeSubject
,From
,To
, andSend
. -
Email Address
Now we will write our email addresses in inverted commas. The email we want to send email from.
-
Password
We will now write the generated password that we have earlier generated through Gmail.
-
Access Gmail Database
To access Gmail’s database, we will use
smtplib.SMTP_SSL('smtp.gmail.com', 465)
. It allows us to get connected with Gmail’s database. -
Log in
Now we will use the
server.login
command to log into the email through the generated password. -
Send
We will now use
v=server.send_message
to send the message through email. -
Print
After the email is sent successfully, we will see a printed message,
Email has been sent successfully
.
Example code:
import smtplib
from email.message import EmailMessage
EMAIL_ADDRESS = "iqraanwar097@gmail.com"
EMAIL_PASSWORD = "***************"
email = EmailMessage()
email["Subject"] = input(str("Email Subject: "))
email["From"] = "Your Name"
email["To"] = "abidork41@gmail.com"
email.set_content("Write Your message here")
server = smtplib.SMTP_SSL("smtp.gmail.com", 465)
server.login(EMAIL_ADDRESS, EMAIL_PASSWORD)
server.send_message(email)
server.quit()
print("Email has been sent successfully")
Output:
Email Subject: Email sent using python
Email has been sent successfully
This is how we can send an email to one email address. But if we want to send emails to multiple addresses, we can separate our emails using a comma and send emails to as many addresses as we want.
Example code:
import smtplib
from email.message import EmailMessage
EMAIL_ADDRESS = "abs@gmail.com"
EMAIL_PASSWORD = "*************"
email = EmailMessage()
email["Subject"] = input(str("Email Subject: "))
email["From"] = "Your Name"
email["To"] = "abidork41@gmail.com,abidork41@gmail.com,abidork41@gmail.com"
email.set_content("Write Your message here")
server = smtplib.SMTP_SSL("smtp.gmail.com", 465)
server.login(EMAIL_ADDRESS, EMAIL_PASSWORD)
server.send_message(email)
server.quit()
print("Email has been sent successfully to all the addresses")
Output:
Email Subject: I have a news for you all
Email has been sent successfully to all the addresses
Our email is sent to all the mentioned addresses.
So this brings us to the end of the discussion! We can quickly and easily send emails to multiple people at once using Python, which only requires a few lines of code on your part.
We hope you find this article helpful in understanding how to send emails to multiple addresses using Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn