How to Select Options From Dropdown Menu With Selenium in Python
- Select Options From the Dropdown Menu With Selenium in Python
-
Use the
select_by_index()
Function to Select Options From the Dropdown Menu Using Selenium in Python -
Use the
select_by_value()
Function to Select Options From Dropdown Menu Using Selenium in Python -
Use the
select_by_visible_text()
Function to Select Options From Dropdown Menu Using Selenium in Python - Conclusion
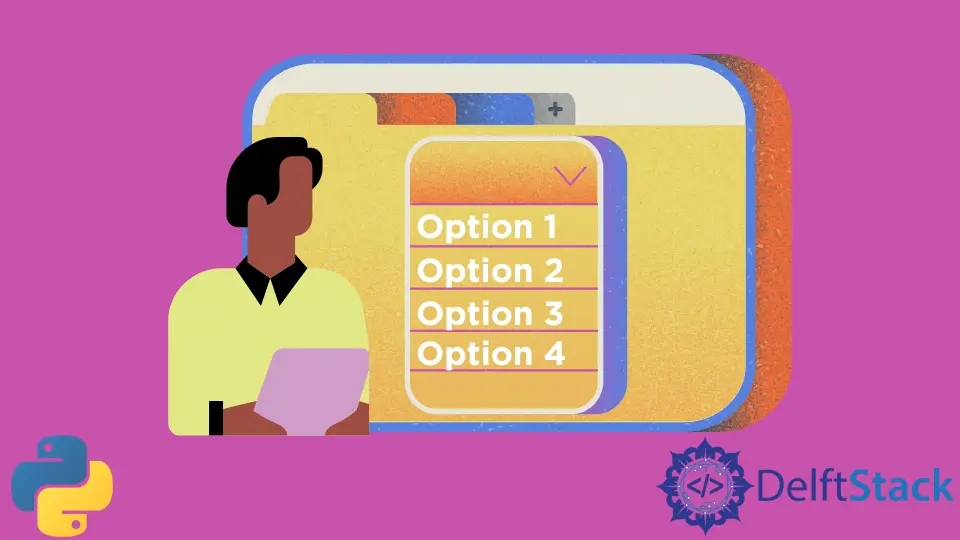
The selenium package is used in Python to automate tasks with Python scripts on a web browser. This can be simple as clicking a button on a web page or as complex as filling a form on the given web page.
This tutorial will discuss selecting from the dropdown menu on a web page using selenium in Python.
Select Options From the Dropdown Menu With Selenium in Python
First, we will be required to initiate an object of the webdriver
class to create a browser window. We will redirect to the required website using the get()
function with this object.
Then, we need to find the HTML element for the dropdown menu. We can use different methods to find this element based on various attributes.
We can use the find_element_by_id()
function to find the element using the id
attribute, the find_element_by_class_name()
function to find it using the class
attribute, and more.
After finding the element, we need to use the Select
class found in the selenium.webdriver.support.ui
. We need to create an object of this class using the Select()
constructor with the retrieved element of the dropdown list.
This object selects options from the dropdown menu using different functions.
These methods are discussed below. Note that these methods will throw the NoSuchElementException
if there is no matching option.
Use the select_by_index()
Function to Select Options From the Dropdown Menu Using Selenium in Python
The select_by_index()
function selects the given option from the menu based on the index
attribute. Note that it does not count the options but uses the index
attribute.
For example,
from selenium.webdriver.support.ui import Select
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/chromedriver.exe")
driver.get("https://www.delftstack.com/")
e = driver.find_element_by_id("Menu_Button")
d = Select(x)
drop.select_by_index(1)
In the above example, we create a webdriver
class object and redirect the window to the website with the get()
function. We retrieve the element for the dropdown menu using the find_element_by_id()
function.
The Select
class object is created with the retrieved element. The select_by_index()
function is used with this object to select the required option.
Use the select_by_value()
Function to Select Options From Dropdown Menu Using Selenium in Python
This method can also select the options that match the value of the value
attribute. The options that match this argument are chosen.
See the code below.
from selenium.webdriver.support.ui import Select
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/chromedriver.exe")
driver.get("https://www.delftstack.com/")
e = driver.find_element_by_id("Menu_Button")
d = Select(x)
drop.select_by_value("value")
The above example will select options that match the given value
attribute.
Use the select_by_visible_text()
Function to Select Options From Dropdown Menu Using Selenium in Python
The select_by_visible_text()
function can be used to select options from the dropdown menu. It selects the option based on its text.
For example,
from selenium.webdriver.support.ui import Select
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/chromedriver.exe")
driver.get("https://www.delftstack.com/")
e = driver.find_element_by_id("Menu_Button")
d = Select(x)
drop.select_by_visible_text("text")
In the above example, we select the option that matches the given text of the option.
Conclusion
This tutorial discussed selecting options from a dropdown menu using selenium in Python. We discussed the use of the Select
class and its different functionalities.
Different methods can choose the option based on various attributes. We can also return the list of selected options using the all_selected_options()
method with the object of this class.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python