How to Capture Screenshot With Selenium in Python
-
Use the
save_screenshot()
Function to Capture a Screenshot Usingselenium
in Python -
Use the
get_screenshot_as_file()
Function to Capture a Screenshot Usingselenium
in Python -
Use the
Screenshot-Selenium
Package to Capture a Screenshot Usingselenium
in Python - Conclusion
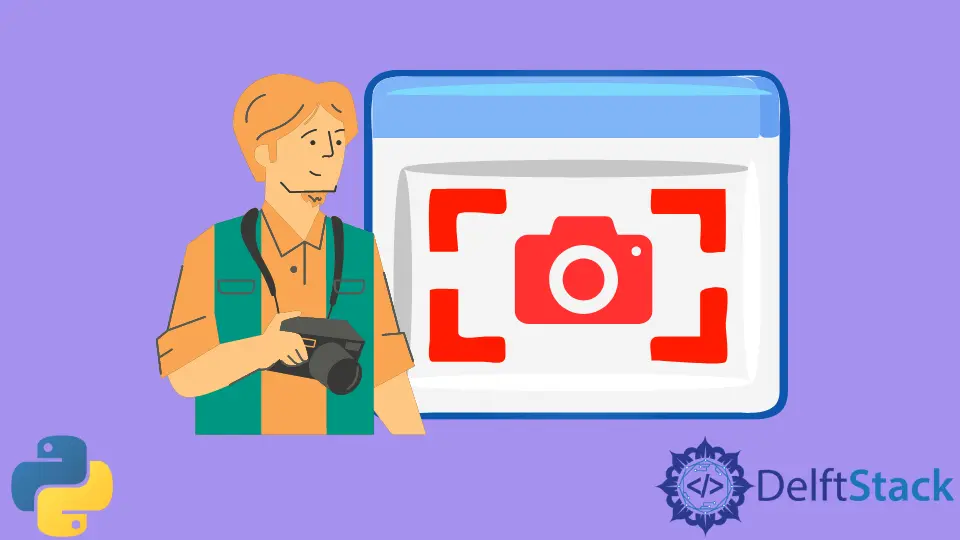
We can perform tasks using a Python script on an automated web browser with selenium
. We can capture the browser’s screen as a screenshot using different methods.
This tutorial will demonstrate how to capture a screenshot of the automated browser using selenium
in Python.
Use the save_screenshot()
Function to Capture a Screenshot Using selenium
in Python
The selenium
package provides the save_screenshot()
function to take a screenshot of the web page opened in the automated browser.
We can specify the filename (PNG format) and the path for the screenshot within the function. This function is used with the webdriver
object.
See the code below.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/driverpath/chromedriver.exe")
driver.get("https://www.delftstack.com/")
driver.save_screenshot("filename.png")
In the above example, we create a webdriver
object and create a connection to the required URL with the get()
function. Then the save_screenshot()
function is used to capture the screenshot and save it.
Use the get_screenshot_as_file()
Function to Capture a Screenshot Using selenium
in Python
The get_screenshot_as_file()
function also creates a file with the screenshot of the webdriver
object. We can save the file at its desired path by mentioning it in the function.
It returns True
if the file is created successfully. If there are any exceptions, the function will return False
.
For example:
from selenium import webdriver
driver = webdriver.Chrome(r"C:/driverpath/chromedriver.exe")
driver.get("https://www.delftstack.com/")
driver.get_screenshot_as_file("filename1.png")
Use the Screenshot-Selenium
Package to Capture a Screenshot Using selenium
in Python
The Selenium-Screenshot
package can be used to capture screenshots of the HTML elements of the webdriver
.
This package is compatible with Python 3 and can be installed using pip install Selenium-Screenshot
. We can also use this to capture the clippings of HTML elements individually.
We can use the full_screenshot()
function to capture the entire browser window. We need to create an object of the Screenshot
class first to use this.
The webdriver
object and the filename are specified within the full_screenshot()
function.
See the code below.
from selenium import webdriver
from Screenshot import Screenshot_Clipping
s = Screenshot_Clipping.Screenshot()
driver = webdriver.Chrome(r"C:/driverpath/chromedriver.exe")
driver.get("https://www.delftstack.com/")
s.full_Screenshot(driver, save_path=r".", image_name="name.png")
We use the full_screenshot()
function in the above example to capture the screenshot. We provide the name and path for the file with the image_name
parameter and save_path
parameter.
Conclusion
We discussed how to take screenshots using selenium
in Python. We can use the save_screenshot()
and get_screenshot_as_file()
functions to save the screenshot as a PNG file.
Alternatively, we can also install a sub-package called Selenium-Screenshot
and use it to capture the web page on the browser.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python