How to Implicit Wait With Selenium in Python
- What is Implicit Wait?
- Setting Implicit Wait Globally
- Managing Implicit Waits with Page Loads
- Best Practices for Using Implicit Waits
- Conclusion
- FAQ
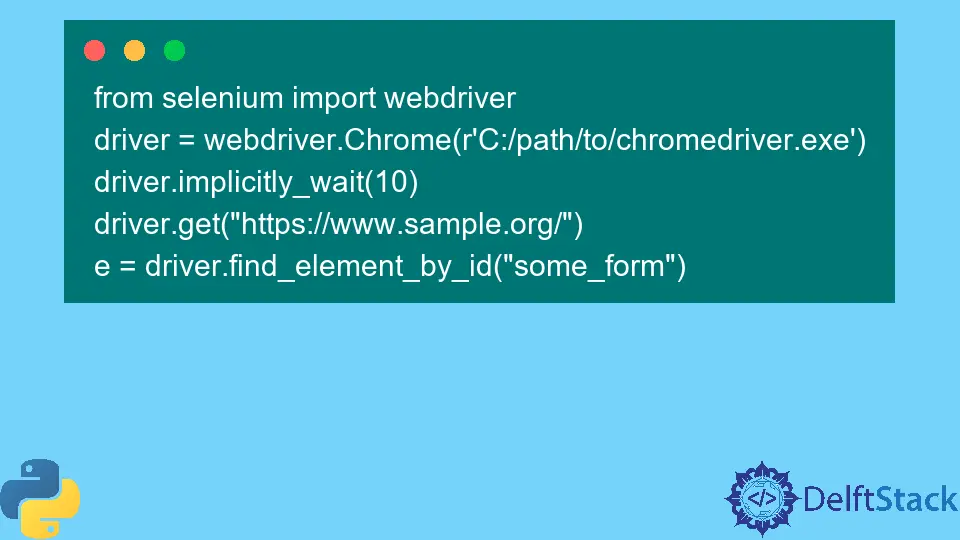
In the fast-paced world of web automation, ensuring that your scripts run smoothly can be a challenge, especially when dealing with dynamic web elements. One of the most effective ways to manage this is by using implicit waits in Selenium with Python.
This tutorial will guide you through the process of setting up implicit waits, allowing your scripts to handle elements that may not be immediately available on the page. By the end of this article, you’ll have a solid understanding of how to implement implicit waits, making your web automation tasks more efficient and reliable. So, let’s dive into the world of Selenium and discover how to make your automation scripts more robust!
What is Implicit Wait?
Implicit wait is a crucial feature in Selenium that allows your script to wait for a specified amount of time when trying to locate an element before throwing a NoSuchElementException
. This prevents your script from failing due to elements not being immediately available on the webpage. Instead of continuously polling for the element, the implicit wait tells Selenium to wait for a certain duration before it gives up. This can be particularly useful in scenarios where elements load dynamically or take time to appear.
To set up an implicit wait in Selenium with Python, you can use the following code snippet:
from selenium import webdriver
driver = webdriver.Chrome()
driver.implicitly_wait(10) # Wait for up to 10 seconds
driver.get("https://example.com")
element = driver.find_element_by_id("some_id")
In this example, we first import the necessary module and create a new instance of the Chrome driver. The implicitly_wait(10)
line tells Selenium to wait for up to 10 seconds when trying to find elements. If the element is found before the time elapses, the script will continue immediately.
Output:
Element found successfully or timeout after 10 seconds
This setup is simple yet powerful, allowing you to handle dynamic content without the need for excessive polling or manual delays.
Setting Implicit Wait Globally
One of the benefits of using implicit waits in Selenium is that you can set them globally for all elements in your script. This means that once you define an implicit wait, all subsequent element searches will adhere to that wait time. This can streamline your code and make it easier to manage.
Here’s how to set an implicit wait globally:
from selenium import webdriver
driver = webdriver.Chrome()
driver.implicitly_wait(15) # Set global wait time to 15 seconds
driver.get("https://example.com")
element1 = driver.find_element_by_id("first_id")
element2 = driver.find_element_by_id("second_id")
In this code, we set the implicit wait to 15 seconds. This means every time we try to find an element, Selenium will wait up to 15 seconds for it to appear. This global setting simplifies your code by eliminating the need to specify wait times for each element search individually.
Output:
First element found successfully or timeout after 15 seconds
Second element found successfully or timeout after 15 seconds
Using global implicit waits can help ensure that your script runs smoothly across multiple element searches, particularly in scenarios where loading times may vary.
Managing Implicit Waits with Page Loads
When working with web pages that have complex loading behaviors, managing implicit waits effectively can be a game-changer. Sometimes, you may want to adjust your implicit wait settings based on the context of your page or the elements you are interacting with.
For instance, if you know that a certain page takes longer to load, you might want to temporarily increase your implicit wait:
from selenium import webdriver
driver = webdriver.Chrome()
# Set a default implicit wait
driver.implicitly_wait(10)
driver.get("https://example.com/slow_page")
driver.implicitly_wait(20) # Increase wait for this specific page
element = driver.find_element_by_id("slow_element")
Here, we begin with a default implicit wait of 10 seconds. Once we navigate to a page that we know is slow to load, we increase the wait to 20 seconds. This flexibility allows your script to adapt to varying conditions, ultimately improving its reliability.
Output:
Slow element found successfully or timeout after 20 seconds
By managing implicit waits in this way, you can ensure that your script is both efficient and resilient, even when dealing with unpredictable load times.
Best Practices for Using Implicit Waits
While implicit waits can significantly enhance your Selenium scripts, there are some best practices to keep in mind. Overusing implicit waits or setting them too high can lead to longer execution times and may mask underlying issues with your web application. Here are some tips to ensure you use implicit waits effectively:
-
Set Reasonable Wait Times: Aim for a balance; too short may lead to frequent timeouts, while too long can slow down your tests unnecessarily.
-
Use Explicit Waits for Specific Scenarios: In cases where you need more control, consider using explicit waits. These allow you to wait for specific conditions, such as visibility or clickability of elements.
-
Avoid Mixing Implicit and Explicit Waits: Mixing the two can lead to unpredictable waits and should generally be avoided.
-
Monitor Performance: Keep an eye on your script’s performance and adjust wait times as necessary.
By following these best practices, you can maximize the effectiveness of implicit waits in your Selenium projects.
Conclusion
Implicit waits in Selenium with Python are a powerful tool that can help streamline your web automation tasks. By allowing your scripts to handle dynamic elements more gracefully, you can reduce the likelihood of errors and improve overall efficiency. Remember to set reasonable wait times, manage your waits based on page load behavior, and consider using explicit waits for more specific scenarios. With these strategies, you’ll be well on your way to mastering Selenium and making your web automation tasks more reliable.
FAQ
-
What is an implicit wait in Selenium?
Implicit wait is a command that tells Selenium to wait for a specified amount of time when trying to find an element before throwing an exception. -
How do I set an implicit wait in Selenium with Python?
You can set an implicit wait by using theimplicitly_wait()
method on your WebDriver instance, specifying the number of seconds to wait. -
Can I change the implicit wait time during execution?
Yes, you can change the implicit wait time at any point in your script by callingimplicitly_wait()
again with a new value. -
What happens if an element is not found within the implicit wait time?
If the element is not found within the specified time, Selenium will throw aNoSuchElementException
. -
Should I use implicit waits or explicit waits?
It depends on your needs. Implicit waits are simpler for general use, while explicit waits provide more control for specific conditions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python