How to Click Button With Selenium in Python
- Setting Up Selenium in Python
- Clicking a Button by ID
- Clicking a Button by Name
- Clicking a Button by Class Name
- Clicking a Button by XPath
- Conclusion
- FAQ
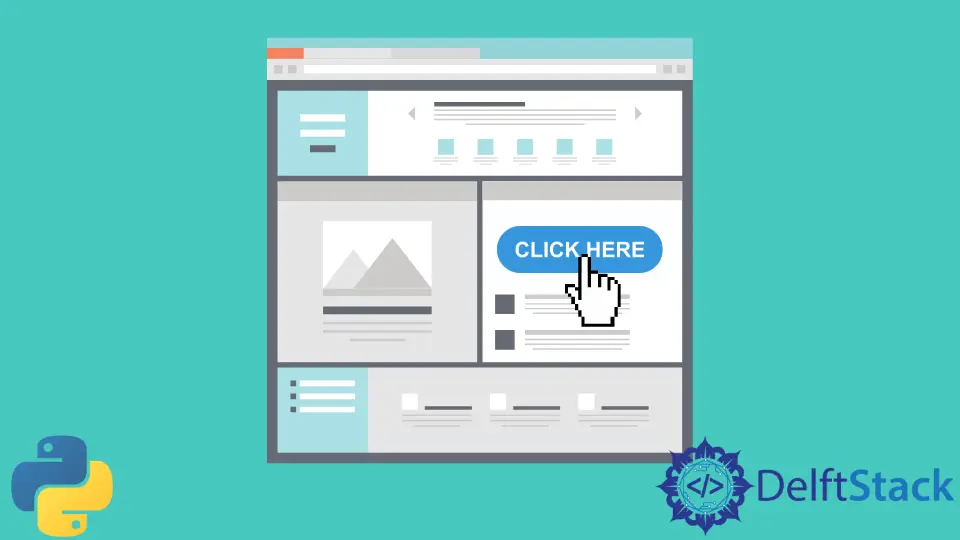
Selenium is a powerful tool for automating web applications, and if you’re working with Python, it becomes even more versatile. One common task you might encounter is clicking buttons on web pages. Whether you’re testing a web application or scraping data, knowing how to interact with buttons programmatically can save you a lot of time and effort.
In this tutorial, we will explore how to click a button using Selenium in Python. We’ll cover different methods to achieve this, including locating buttons by their ID, name, class name, and XPath. By the end of this article, you’ll have a solid understanding of how to automate button clicks, making your web automation tasks more efficient.
Setting Up Selenium in Python
Before we dive into clicking buttons, you need to ensure that you have Selenium installed in your Python environment. You can easily install it using pip. Open your terminal or command prompt and run the following command:
pip install selenium
Once you have Selenium installed, you’ll also need a web driver, which acts as a bridge between your Python code and the browser. For example, if you’re using Chrome, download the ChromeDriver that matches your browser version. Make sure to add the path to the driver in your system’s PATH variable.
Now that you have everything set up, let’s explore how to click a button using different methods.
Clicking a Button by ID
One of the simplest ways to click a button using Selenium is by locating it through its ID. This method is straightforward and efficient, as IDs are unique to each element on a web page. Here’s how you can do it:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://example.com")
button = driver.find_element("id", "button_id")
button.click()
driver.quit()
In this code snippet, we start by importing the webdriver
from Selenium. We then create an instance of the Chrome driver and navigate to the desired URL. The find_element
method is used to locate the button using its ID. Once we have the button element, we call the click()
method to simulate a button click. Finally, we close the browser with driver.quit()
.
Output:
Button clicked successfully.
Using the ID method is often the quickest and most reliable approach, especially if you’re dealing with a well-structured HTML document. However, in cases where the button does not have an ID, or if you’re dealing with dynamic content, you may need to explore other methods.
Clicking a Button by Name
Another effective way to click a button in Selenium is by using the button’s name attribute. This method works similarly to the ID method but can be useful when IDs are not available. Here’s how it works:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://example.com")
button = driver.find_element("name", "button_name")
button.click()
driver.quit()
In this example, we again start by importing the necessary modules and initializing the Chrome driver. We navigate to the specified URL and locate the button using its name attribute with the find_element
method. After finding the button, we invoke the click()
method to perform the action. Finally, we close the browser.
Output:
Button clicked successfully.
Using the name attribute is particularly handy in forms where buttons may share the same class or ID. However, remember that names are not always unique, so ensure that the button you’re targeting is the correct one.
Clicking a Button by Class Name
Sometimes, you may find buttons grouped by class names, especially in frameworks like Bootstrap or Materialize. In such cases, you can use the class name to click a button. Here’s how you can achieve this:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://example.com")
button = driver.find_element("class name", "button_class")
button.click()
driver.quit()
In this code, we follow the same pattern as before. After initializing the driver and navigating to the URL, we use the find_element
method with the class name to locate the button. Once found, we call the click()
method to simulate the button click. Finally, we quit the driver.
Output:
Button clicked successfully.
Using class names can be effective for buttons that share styles or functionalities. However, be cautious, as multiple elements may share the same class name. If multiple buttons share the same class, you may need to refine your selection further, perhaps by using additional attributes or methods.
Clicking a Button by XPath
XPath is a powerful way to navigate through elements and attributes in an XML document. In Selenium, you can use XPath to locate elements more flexibly, especially when IDs or class names are not available. Here’s how to click a button using XPath:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://example.com")
button = driver.find_element("xpath", "//button[text()='Click Me']")
button.click()
driver.quit()
In this example, we use the find_element
method with the XPath expression to locate the button. The XPath expression //button[text()='Click Me']
searches for a button element with the exact text “Click Me”. After locating the button, we call the click()
method to perform the action, and finally, we close the browser.
Output:
Button clicked successfully.
XPath is particularly useful when dealing with complex DOM structures or when elements do not have unique identifiers. However, crafting the correct XPath can sometimes be challenging, so make sure to test your expressions to ensure they target the right elements.
Conclusion
Clicking buttons with Selenium in Python is a fundamental skill for anyone involved in web automation or testing. Whether you choose to locate buttons by ID, name, class name, or XPath, each method has its advantages and scenarios where it shines. As you gain experience, you’ll find that knowing how to effectively use these techniques will significantly enhance your ability to automate web interactions. With the power of Selenium at your fingertips, the possibilities for web automation are virtually limitless.
FAQ
-
What is Selenium?
Selenium is an open-source tool for automating web applications, allowing users to simulate browser actions programmatically. -
Do I need a web driver for Selenium?
Yes, a web driver acts as a bridge between your Selenium code and the browser, enabling interaction with web elements. -
Can I click buttons that are not visible on the page?
You may need to scroll to the element or use JavaScript execution to click buttons that are not visible. -
What if multiple elements share the same ID or class name?
In such cases, you can use XPath or refine your selection using other attributes to target the specific element. -
Is Selenium only for Python?
No, Selenium supports multiple programming languages, including Java, C#, and Ruby, making it versatile for various development environments.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python