How to Round to Two Decimals in Python
-
Use the
round()
Function to Round to Two Decimal Places in Python -
Use the
math
Library to Round to Two Decimal Places in Python -
Use the
%
Formatting to Round to Two Decimal Places in Python -
Use the
format()
Function to Round to Two Decimal Places in Python -
Use the
f-strings
to Round to Two Decimal Places in Python
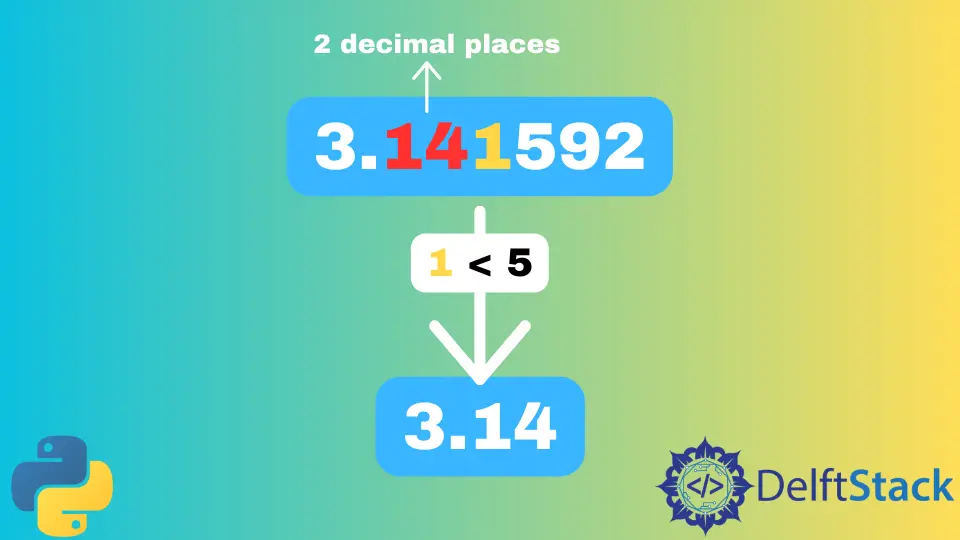
This tutorial demonstrates Python’s five ways to round numbers to two decimal places. These approaches include round()
function, math
library, string formatting, format()
function, and f-strings
.
We will use the float
data type to illustrate each. Let’s start with the most straightforward method for rounding a number.
Use the round()
Function to Round to Two Decimal Places in Python
The round()
function is used to round off numbers to the specified decimal places. The original number is returned if it contains less than the given decimal places.
To round a number, we need to provide the number and total decimal places as the parameters of this function.
Example Code:
a = 7.852
print(round(a, 2))
Output:
7.85
As we can see, we’ve rounded a number to two decimal places in the above example.
Use the math
Library to Round to Two Decimal Places in Python
The math
library provides different functionalities to perform complicated mathematical calculations.
The ceil()
and floor()
functions of the math
library are used to round a number downward or upward to its closest integer, respectively.
We can use these functions to create custom methods to round up and down a given float value to two decimal places.
Example Code:
import math
def r_down(number, decimals=2):
a = 10 ** decimals
return math.floor(number * a) / a
print(r_down(7.852))
def r_up(number, decimals=2):
a = 10 ** decimals
return math.ceil(number * a) / a
print(r_up(7.852))
Output:
7.85
7.86
The above code demonstrates two user-defined functions. The first method, r_down
, uses the math.floor()
function to round down a given number to two decimal places.
The second function, r_up,
uses the math.ceil()
function to round up a number to two decimal places.
Use the %
Formatting to Round to Two Decimal Places in Python
In Python, we use string formatting to view the final result in our desired form. We can also use string formatting to round off numbers, but it converts the final result to a string. Remember, we can use type casting to convert the data type back to float
.
Python provides different ways to perform string formatting. The most basic formatting can be done using the %
sign. For example, using the %.2f
format, we can view numbers with only two decimal places.
Example Code:
a = 7.852
print("%.2f" % a)
Output:
7.85
Use the format()
Function to Round to Two Decimal Places in Python
The format()
function provides another way for string formatting in Python. We can provide the desired style of the output string within the function along with the variable. We can use the .2f
format within this function as well.
Example Code:
a = 7.852
print("{:.2f}".format(a))
Output:
7.85
Use the f-strings
to Round to Two Decimal Places in Python
The f-strings
is a new addition in Python 3 and provides an elegant and efficient way to format strings. We can also use it to round to two decimal places in Python.
Example Code:
a = 7.852
print(f"{a:.2f}")
Output:
7.85
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn