How to Reverse Integer in Python
- Use the Mathematical Approach to Check if a Number is a Palindrome in Python
- Use the String Reversal Approach to Check if a Number is Palindrome in Python
- Use the List Reversal Approach to Find if a Number is a Palindrome in Python
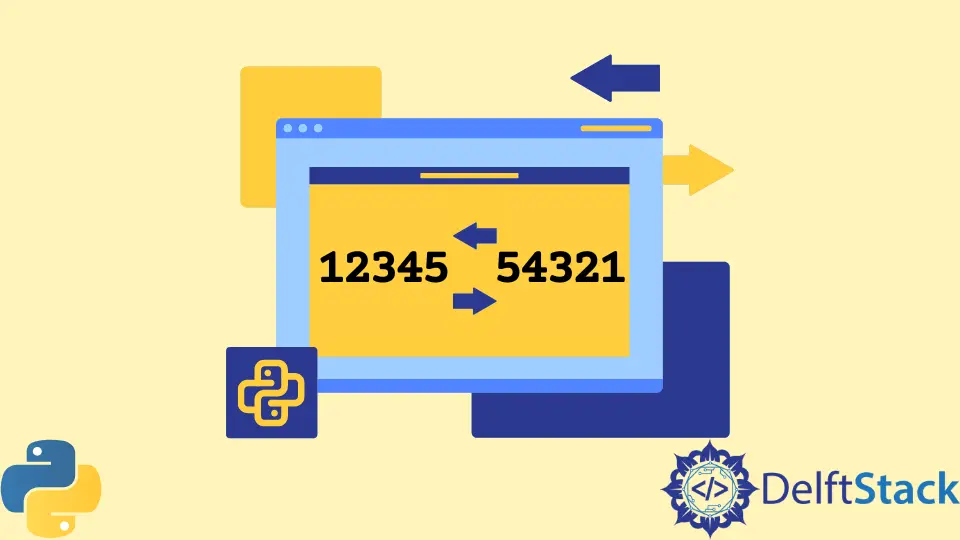
A word or a number, when its characters or the digits are reversed, and if it remains the same, then it is called a palindrome. This article will introduce some methods to check if a number is a palindrome by reversing the provided number.
Use the Mathematical Approach to Check if a Number is a Palindrome in Python
We can check if a number is a palindrome using the mathematical approach. Firstly, this method reverses the number and then checks the reversed number with the original number. If both values are the same, then the number is a palindrome. We can apply mathematical operations like modulo, addition, multiplication, and division to reverse a number.
For example, ask for input from the user using the input()
function and store it to the original_number
variable. Create a variable copy_number
and store the copy of original_number
in it. Create another variable, reversed_number
, and initialize it to 0
. Use the while
loop to check if the original_number
is greater than 0
. Inside the loop, store the remainder of the orignal_number
divided by 10
in a remainder
variable. Use the modulo %
to find the remainder. Then, multiply the reversed_number
by 10
and add remainder
to it. Store the value in the reversed_number
variable. Next, divide the original_number
by 10
and store the value in the same orginal_number
variable. Check if the value of copy_number
and reversed_number
is the same, outside the loop. If the values are same, then print the message saying it is a palindrome and vice versa.
In the example below, the state of the original_number
changes in each iteration, so we stored a copy of it in the variable copy_number
. The number given by the user is 12321
, which is the same when it is reversed, and the program shows that it is a palindrome. Thus, we can use the mathematical approach to check if a number is a palindrome.
Example Code:
# python 3.x
original_number = int(input("Enter an integer: "))
copy_number = original_number
reversed_number = 0
while original_number > 0:
remainder = original_number % 10
reversed_number = reversed_number * 10 + remainder
original_number = original_number // 10
if copy_number == reversed_number:
print(copy_number, "is a palindrome number")
else:
print(copy_number, "is not a palindrome number")
Output:
Enter an integer: 12321
12321 is a palindrome number
Use the String Reversal Approach to Check if a Number is Palindrome in Python
We can use the string reversal approach in Python to check if a number is a palindrome. We can use the [::-1]
short-hand operation for the palindrome check. It is a one-liner for reversing an iterable, i.e., lists, strings, tuples, etc. As the operator works with the string, we can convert the integer value to a string, reverse the string and then convert it to an integer.
For example, take an integer input from the user and store it in the number
variable. Convert the number
into the string with the str()
function and use the [::-1]
operation with it. Wrap the whole expression with the int()
function to convert the reversed value into an integer. Finally, use the if
condition to compare the number
variable with the reversed string and display the message accordingly.
In the example below, we took an integer number as input, converted it to a string, and reversed it. Then reversed number is compared to the integer and compared with the number
variable. When we reverse the number 454
, it becomes the same, but when we reverse the number 456
, it becomes 654
. Thus, we found if the number
variable is a palindrome.
Example Code:
# python 3.x
number = int(input("Enter a number : "))
if number == int(str(number)[::-1]):
print(number, "is palindrome.")
else:
print(number, "is not palindrome.")
Output:
Enter a number : 454
454 is palindrome.
Enter a number : 456
456 is not palindrome.
Use the List Reversal Approach to Find if a Number is a Palindrome in Python
Using the List reversal method, we can reverse the number and check whether it is a palindrome. We can ask for an integer input and convert it into a string using the str()
function. The list()
function can be used that converts the string to a list. We can use the reverse()
function to reverse the list items. We can convert the list items into a string using the ' '
separator with the join()
method. Finally, we can return the integer representation of the generated string. Thus, we can compare the reversed number with the original number to check if it is a palindrome.
For example, create a variable original_number
and store the input asked from the user. Convert the number into a string applying the str()
function and then list applying the list()
function. Store the result in the lst_number
variable. Use the reverse()
function on the lst_number
to reverse the list elements. Use the ''
separator to call the join()
method on lst_number
to convert the list items to a single string. Use the int()
function to convert the string into an integer and compare it with the original_number
. Thus, we can find if the number is a palindrome.
Example Code:
# python 3.x
original_number = int(input("Enter an integer : "))
lst_number = list(str(original_number))
lst_number.reverse()
reversed_number = "".join(lst_number)
if original_number == int(reversed_number):
print(original_number, "is palindrome.")
else:
print(original_number, "is not palindrome.")
Output:
Enter an integer : 99
99 is palindrome.