How to Remove First Element From a List in Python
-
Use the
pop()
Method to Remove the First Element From a List in Python -
Use the
remove()
Method to Remove the First Element From a List in Python -
Use the
del
Keyword to Delete an Element From a List in Python - Use the List Slicing Method to Remove the First Element From a List in Python
-
Use the
numpy.delete()
Function to Remove the First Element From a List in Python -
Use the
popleft()
Function to Remove the First Element From a List in Python
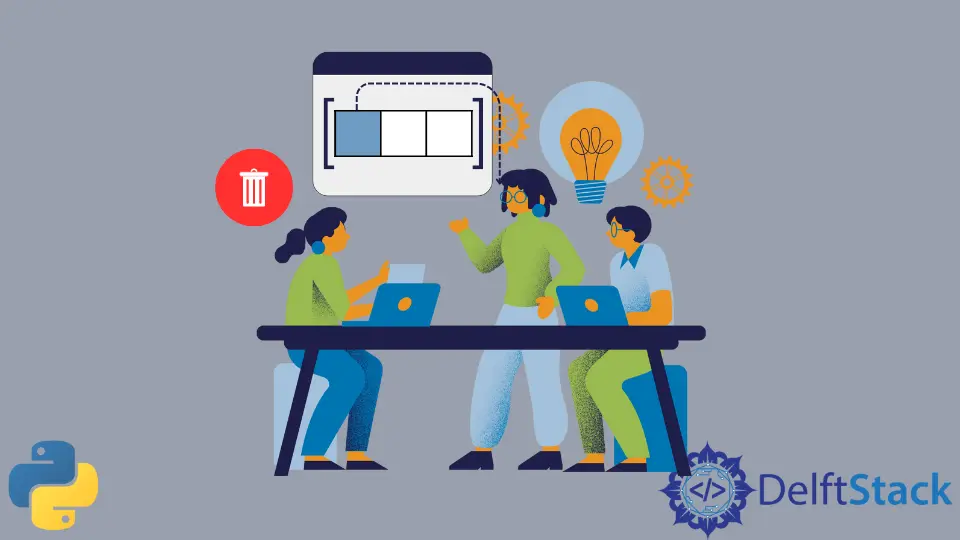
This tutorial will discuss different methods of how to remove the first element from a list.
Use the pop()
Method to Remove the First Element From a List in Python
The pop()
method can remove an element from a specific index. We have to specify the index from where the element is to be removed.
In our case, we have to remove the first element, so we have to use the index 0
.
For example,
list1 = ["ram", "ravi", "shyaam"]
list1.pop(0)
print(list1)
Output:
['ravi','shyaam']
If no index is specified, then it removes the last element.
Use the remove()
Method to Remove the First Element From a List in Python
The remove
method can delete any required element from the list. Here instead of the index, we have to write the name of the element to be removed.
In our case, we will write the first element of the list.
For example,
list1 = ["ram", "ravi", "shyaam"]
list1.remove("ram")
print(list1)
Output:
['ravi','shyaam']
Suppose we don’t know the first element, and checking can be time-consuming again and again. To avoid this, we can also use the remove()
method in the following way.
list1 = ["ram", "ravi", "shyaam"]
list1.remove(list1[0])
print(list1)
Output:
['ravi','shyaam']
Use the del
Keyword to Delete an Element From a List in Python
The del
keyword also removes the element from a specific index. We will be writing 0 in the brackets as it specifies the first element from the list.
For example,
list1 = ["ram", "ravi", "shyaam"]
del list1[0]
print(list1)
Output:
['ravi','shyaam']
Use the List Slicing Method to Remove the First Element From a List in Python
We can use the slicing method also to remove the first element. This method is the most commonly used by the programmer to solve this problem.
Here, we specify the starting element from where we want our list to have and the last value needed. In our case, we will be starting from index 1 to n-1 to delete the first element.
See the code below.
list1 = ["ram", "ravi", "shyaam"]
list1 = list1[1:]
print(list1)
Output:
['ravi','shyaam']
Use the numpy.delete()
Function to Remove the First Element From a List in Python
We can use the delete()
function from the NumPy
module. First, we convert the list to an array using numpy.array()
function, and then using the delete()
method to delete the required element.
For example,
import numpy as np
list1 = ["ram", "ravi", "shyaam"]
arr = np.array(list1)
arr = np.delete(arr, 0)
print(arr)
Output:
['ravi','shyaam']
Use the popleft()
Function to Remove the First Element From a List in Python
The popleft()
function removes the element one by one from the starting. But first, we convert the list into deque and then use this method. After the required transformation, we reverse the deque back to the list and then print the output.
The collections
module needs to be imported first to use this method.
See the code below.
import collections
list1 = ["ram", "ravi", "shyaam"]
# convert list to deque
deq = collections.deque(list1)
# removing from left side
deq.popleft()
# convert deque back to list
list1 = list(deq)
print(list1)
Output:
['ravi', 'shyaam']
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python