Python while Loop With Multiple Conditions
-
Use
and
andor
Logical Operators to Make a Pythonwhile
Loop With Multiple Conditions -
Use
not
Logical Operators to Make a Pythonwhile
Loop With Multiple Conditions
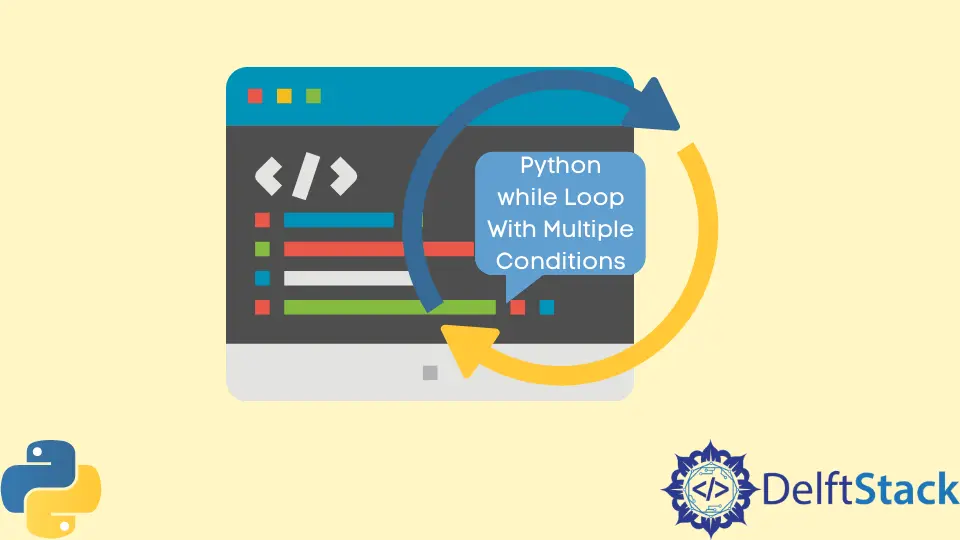
The while
loop in Python is a loop that helps run the code until the condition in the while
statement, i.e., the test condition, becomes true. This loop is used when the user doesn’t know the number of iterations to be carried out beforehand. There are many cases in which a while
loop is used in multiple conditions.
In this tutorial, we will see how to use while
loops with multiple conditions.
Use and
and or
Logical Operators to Make a Python while
Loop With Multiple Conditions
The and
logical operator evaluates the whole expression first and then returns the result based on that evaluation. If any one of the two conditions is not satisfied or is not true, then the statement will be considered false, and the code won’t run.
Example:
x = 10
y = 20
initial_count = 0
while initial_count < x and initial_count < y:
print(f"count: {initial_count}, x = {x}, y = {y}")
initial_count += 1
Output:
count: 0, x = 10, y = 20
count: 1, x = 10, y = 20
count: 2, x = 10, y = 20
count: 3, x = 10, y = 20
count: 4, x = 10, y = 20
count: 5, x = 10, y = 20
count: 6, x = 10, y = 20
count: 7, x = 10, y = 20
count: 8, x = 10, y = 20
count: 9, x = 10, y = 20
In this method, we first make two variables, x, and y, equal to 10 and 20, respectively. Then we initialize the count equal to zero. After doing all this, we start with our while
loop, which makes two conditions as shown in the above code. Finally, the logical and
operator comes into play. This operator first combines the two conditions created by the user into one and then checks both the conditions as a whole. If both the conditions turn out to be true, then the loop executes; otherwise, it doesn’t. Also, this operator stops evaluating after finding the first statement to be true. Like in this case, the loop stopped working after the count of 10.
Let’s move on to the or
logical operator. Just like the and
logical operator, the or
operator also evaluates the given conditions and then combines both the conditions as one condition. The advantage of using this operator is that even if one of the two conditional statements is true, the code will execute. If both the statements turn out to be false, then the code will not run.
Example:
x = 10
y = 20
initial_count = 0
while initial_count < x or initial_count < y:
print(f"count: {initial_count}, x = {x}, y = {y}")
initial_count += 1
Output:
count: 0, x = 10, y = 20
count: 1, x = 10, y = 20
count: 2, x = 10, y = 20
count: 3, x = 10, y = 20
count: 4, x = 10, y = 20
count: 5, x = 10, y = 20
count: 6, x = 10, y = 20
count: 7, x = 10, y = 20
count: 8, x = 10, y = 20
count: 9, x = 10, y = 20
count: 10, x = 10, y = 20
count: 11, x = 10, y = 20
count: 12, x = 10, y = 20
count: 13, x = 10, y = 20
count: 14, x = 10, y = 20
count: 15, x = 10, y = 20
count: 16, x = 10, y = 20
count: 17, x = 10, y = 20
count: 18, x = 10, y = 20
count: 19, x = 10, y = 20
Note that in this method, the loop doesn’t stop after the first statement. It is because the operator finds out that the second condition is also true. If the first statement was true and the second statement was not true, then the loop would stop after the count of 10.
Use not
Logical Operators to Make a Python while
Loop With Multiple Conditions
There is one more logical operator by which we can use the while
loop in multiple conditions, and that operator is called the not
operator. This operator is used to return the opposite value of the boolean statement. For example, if the boolean state is not False
, then true will be returned, and if the boolean statement is not True
, then false will be returned.
Example:
x = 10
y = 20
initial_count = 0
while not initial_count > x and not initial_count > y:
print(f"count: {initial_count}, x = {x}, y = {y}")
initial_count += 1
Output:
count: 0, x = 10, y = 20
count: 1, x = 10, y = 20
count: 2, x = 10, y = 20
count: 3, x = 10, y = 20
count: 4, x = 10, y = 20
count: 5, x = 10, y = 20
count: 6, x = 10, y = 20
count: 7, x = 10, y = 20
count: 8, x = 10, y = 20
count: 9, x = 10, y = 20
count: 10, x = 10, y = 20
Here, the while
statements mean that if the initial count is not greater than x
and the initial count is not greater than y
, only return the following code. Also, note that the logical operation and
is also used in the above code, which means the and
operator finds the first not
statement true and doesn’t evaluate the second not
statement. Finally, the loop starts iterating and ends at the count of 10.
So, just like we have taken only two conditions in the while
loop as an example, more of these statements can be added.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn