Python while ループと複数の条件
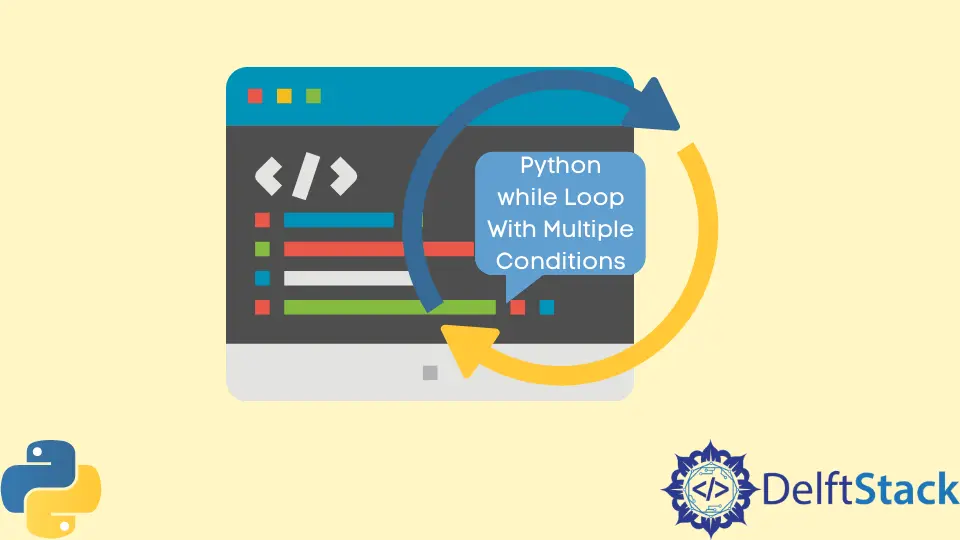
Python の while
ループは、while
ステートメントの条件、つまりテスト条件が真になるまでコードを実行するのに役立つループです。このループは、ユーザーが事前に実行する反復回数がわからない場合に使用されます。while
ループが複数の条件で使用される場合が多くあります。
このチュートリアルでは、複数の条件で while
ループを使用する方法を説明します。
複数の条件で Python の while
ループを作るために and
と or
の論理演算子を使う
and
論理演算子は、最初に式全体を評価し、次にその評価に基づいて結果を返します。2つの条件のいずれかが満たされないか、真でない場合、ステートメントは偽と見なされ、コードは実行されません。
例:
x = 10
y = 20
initial_count = 0
while initial_count < x and initial_count < y:
print(f"count: {initial_count}, x = {x}, y = {y}")
initial_count += 1
出力:
count: 0, x = 10, y = 20
count: 1, x = 10, y = 20
count: 2, x = 10, y = 20
count: 3, x = 10, y = 20
count: 4, x = 10, y = 20
count: 5, x = 10, y = 20
count: 6, x = 10, y = 20
count: 7, x = 10, y = 20
count: 8, x = 10, y = 20
count: 9, x = 10, y = 20
この方法では、最初に、それぞれ 10 と 20 に等しい 2つの変数 x と y を作成します。次に、ゼロに等しいカウントを初期化します。これらすべてを実行した後、while
ループから開始します。これにより、上記のコードに示すように 2つの条件が作成されます。最後に、論理 and
演算子が機能します。このオペレーターは、最初にユーザーが作成した 2つの条件を 1つに結合し、次に両方の条件を全体としてチェックします。両方の条件が真であることが判明した場合、ループが実行されます。そうでなければ、そうではありません。また、この演算子は、最初のステートメントが真であると判断した後、評価を停止します。この場合のように、ループは 10 カウント後に動作を停止しました。
or
論理演算子に移りましょう。and
論理演算子と同様に、or
演算子も指定された条件を評価し、両方の条件を 1つの条件として結合します。この演算子を使用する利点は、2つの条件ステートメントのいずれかが真であっても、コードが実行されることです。両方のステートメントが false であることが判明した場合、コードは実行されません。
例:
x = 10
y = 20
initial_count = 0
while initial_count < x or initial_count < y:
print(f"count: {initial_count}, x = {x}, y = {y}")
initial_count += 1
出力:
count: 0, x = 10, y = 20
count: 1, x = 10, y = 20
count: 2, x = 10, y = 20
count: 3, x = 10, y = 20
count: 4, x = 10, y = 20
count: 5, x = 10, y = 20
count: 6, x = 10, y = 20
count: 7, x = 10, y = 20
count: 8, x = 10, y = 20
count: 9, x = 10, y = 20
count: 10, x = 10, y = 20
count: 11, x = 10, y = 20
count: 12, x = 10, y = 20
count: 13, x = 10, y = 20
count: 14, x = 10, y = 20
count: 15, x = 10, y = 20
count: 16, x = 10, y = 20
count: 17, x = 10, y = 20
count: 18, x = 10, y = 20
count: 19, x = 10, y = 20
このメソッドでは、ループは最初のステートメントの後で停止しないことに注意してください。これは、オペレーターが 2 番目の条件も真であることに気付いたためです。最初のステートメントが真で、2 番目のステートメントが真でない場合、ループは 10 カウント後に停止します。
複数の条件で not
論理演算子を使用して Python の while
ループを作成する
複数の条件で while
ループを使用できる論理演算子がもう 1つあり、その演算子は not
演算子と呼ばれます。この演算子は、ブールステートメントの反対の値を返すために使用されます。たとえば、ブール状態が not False
の場合は true が返され、ブールステートメントが not True
の場合は false が返されます。
例:
x = 10
y = 20
initial_count = 0
while not initial_count > x and not initial_count > y:
print(f"count: {initial_count}, x = {x}, y = {y}")
initial_count += 1
出力:
count: 0, x = 10, y = 20
count: 1, x = 10, y = 20
count: 2, x = 10, y = 20
count: 3, x = 10, y = 20
count: 4, x = 10, y = 20
count: 5, x = 10, y = 20
count: 6, x = 10, y = 20
count: 7, x = 10, y = 20
count: 8, x = 10, y = 20
count: 9, x = 10, y = 20
count: 10, x = 10, y = 20
ここで、while
ステートメントは、初期カウントが x
以下で、初期カウントが y
以下の場合、次のコードのみを返すことを意味します。また、上記のコードでは論理演算 and
も使用されていることに注意してください。つまり、and
演算子は最初の not
ステートメントが true であると判断し、2 番目の not
ステートメントを評価しません。最後に、ループは反復を開始し、10 のカウントで終了します。
したがって、例として while
ループで 2つの条件のみを取り上げたように、これらのステートメントをさらに追加できます。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn