How to Fix the TypeError: Object of Type NoneType Has No Len() in Python
-
Cause of the
TypeError: object of type 'NoneType' has no len()
in Python -
Fix the
TypeError: object of type 'NoneType' has no len()
in Python
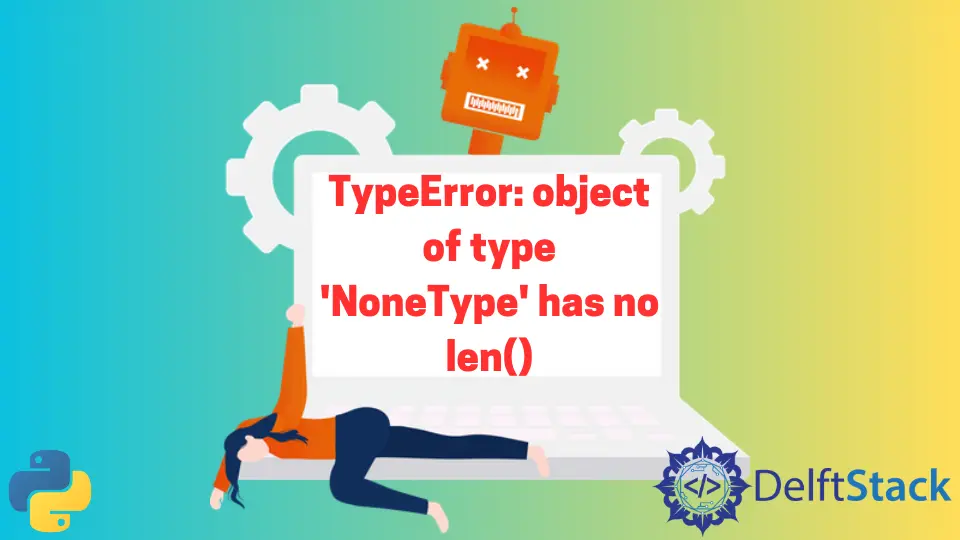
In Python, the len
function is used to find the length of any collection. But, when you try to pass a None
value to the len
function, this causes a TypeError: object of type 'datatype' has no len()
error because the len
function does not support the None
value.
Cause of the TypeError: object of type 'NoneType' has no len()
in Python
As discussed, in Python None
value has no length; the len
function does not support it. To understand it better, let’s have an example.
Code example:
none_val = None
print(type(none_val))
print(len(none_val))
Output:
<class 'NoneType'>
TypeError: object of type 'NoneType' has no len()
As you can see in the above example, we are finding the length with the len
function of the none_val
variable whose value is None
. But this has caused a TypeError: object of type 'NoneType' has no len()
.
Let’s see whether the len
function supports the None
type to understand the reason for this error.
none_val = None
print(dir(none_val))
Output:
['__bool__', '__class__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__']
As you can see in the list provided by the dir()
function, it is used to enlist all the built-in functions of the object. In this case, we have passed the object of the None
type to the dir
function, which displayed all the built-in supported functions.
But the point to note here is that the len
function is not included in the above list.
Fix the TypeError: object of type 'NoneType' has no len()
in Python
Now, let’s try to fix the TypeError: object of type 'NoneType' has no len()
.
my_list = [1, 2, 3, 4, 5]
my_tpl = (1, 2, 3, 4, 5)
none_list = [None, None, None, None, None]
print("This length of my_list is ", len(my_list))
print("This length of my_tpl is ", len(my_tpl))
print("This length of none_list is ", len(none_list))
Output:
This length of my_list is 5
This length of my_tpl is 5
This length of none_list is 5
As you can see, when we try to find the length of a collection like a list or a tuple with the len
function, it runs smoothly because len
supports collections.
Also, you can find the length of any collection that contains None
values. See the list none_list
as displayed in the above example.
And to verify that any collection supports the len
function, you can pass the collection to the dir
function. See the example below.
my_list = [1, 2, 3, 4, 5]
print(dir(my_list))
Output:
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
As you can see, this list has the len
function, which means the collection list my_list
supports the len
function.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python