How to Compare Python Tuples
- Understanding Tuple Comparison
- Method 1: Using the Equality Operator
- Method 2: Using the Inequality Operator
- Method 3: Using Comparison Operators
-
Method 4: Using the
all()
Function for Element-wise Comparison - Conclusion
- FAQ
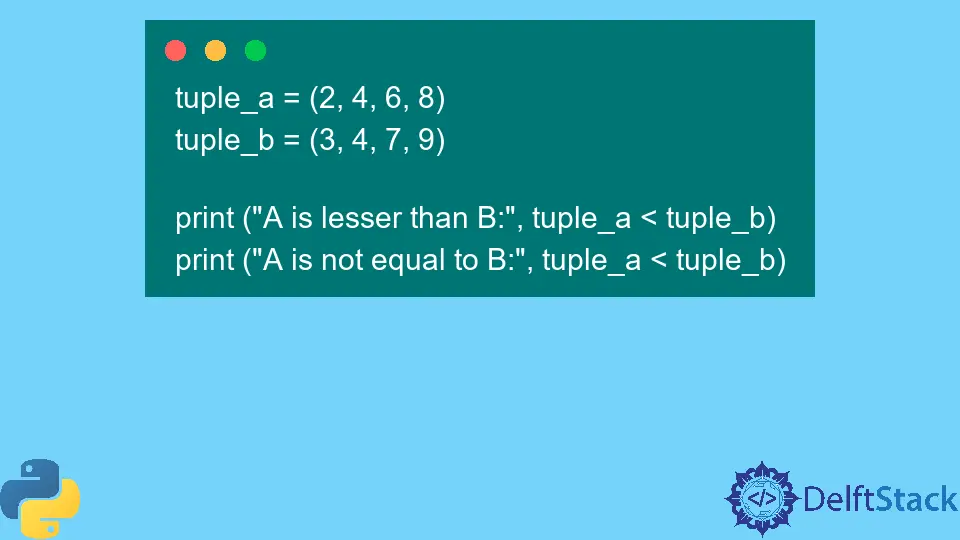
When working with Python, you might often find yourself needing to compare tuples. Tuples are immutable sequences, meaning once they are created, they cannot be modified. This characteristic makes them particularly useful for storing fixed collections of items. Comparing tuples can help you determine their equality, order, and even their contents.
In this article, we’ll explore various methods for comparing tuples in Python, from simple equality checks to more complex comparisons. Whether you’re a beginner or an experienced programmer, you’ll find valuable insights here that can enhance your understanding of tuples in Python.
Understanding Tuple Comparison
Before diving into the methods for comparing tuples, it’s essential to grasp how Python handles tuple comparison. Python compares tuples lexicographically, which means it compares the first elements of each tuple, then the second, and so on. If the first elements are equal, it moves on to the next elements until it finds a difference or reaches the end of the tuples. If one tuple is shorter than the other and all previous elements are equal, the shorter tuple is considered less than the longer one.
This natural ordering makes it straightforward to compare tuples containing comparable elements, such as numbers or strings.
Method 1: Using the Equality Operator
The simplest way to compare two tuples is by using the equality operator (==
). This method checks if both tuples have the same elements in the same order. If they do, it returns True
; otherwise, it returns False
.
Here’s a quick example:
tuple1 = (1, 2, 3)
tuple2 = (1, 2, 3)
tuple3 = (1, 2, 4)
result1 = tuple1 == tuple2
result2 = tuple1 == tuple3
Output:
True
Output:
False
In this example, tuple1
and tuple2
are identical, so the first comparison returns True
. However, tuple1
and tuple3
differ in the last element, leading to a False
result for the second comparison. This method is straightforward and effective for checking equality between tuples.
Method 2: Using the Inequality Operator
In addition to checking for equality, you can also use the inequality operator (!=
) to determine if two tuples are not equal. This operator works similarly to the equality operator but returns True
if the tuples are different and False
if they are the same.
Here’s how you can implement this:
tuple1 = (5, 6, 7)
tuple2 = (5, 6, 8)
result1 = tuple1 != tuple2
result2 = tuple1 != (5, 6, 7)
Output:
True
Output:
False
In this case, tuple1
and tuple2
are not equal because of the last element, so the first result is True
. The second comparison checks tuple1
against itself, which is why it returns False
. This method is useful for quickly identifying differences between tuples.
Method 3: Using Comparison Operators
Python allows you to use comparison operators such as <
, <=
, >
, and >=
to compare tuples. These operators will compare tuples lexicographically, just like the equality and inequality operators.
For example:
tuple1 = (1, 2, 3)
tuple2 = (1, 2, 4)
tuple3 = (1, 2)
result1 = tuple1 < tuple2
result2 = tuple1 > tuple3
Output:
True
Output:
True
In this example, tuple1
is less than tuple2
because the third elements differ, and tuple1
is greater than tuple3
since tuple3
is shorter and all its elements match tuple1
. This method is particularly useful when you need to sort tuples or determine their order relative to one another.
Method 4: Using the all()
Function for Element-wise Comparison
If you need to compare tuples element by element, you can use the built-in all()
function in combination with a generator expression. This method allows you to check if all corresponding elements in two tuples are equal.
Here’s how you can do it:
tuple1 = (1, 2, 3)
tuple2 = (1, 2, 3)
tuple3 = (1, 2, 4)
result1 = all(a == b for a, b in zip(tuple1, tuple2))
result2 = all(a == b for a, b in zip(tuple1, tuple3))
Output:
True
Output:
False
In this case, zip()
pairs elements from both tuples, and all()
checks if all pairs are equal. The first comparison returns True
, while the second returns False
because the last elements differ. This method is particularly handy when comparing larger tuples or when you want to implement custom comparison logic.
Conclusion
Comparing tuples in Python is a straightforward process thanks to the various operators and functions available. Whether you need to check for equality, inequality, or order, Python provides intuitive ways to handle these comparisons. Understanding how to effectively compare tuples can help you write more efficient and cleaner code. As you continue to work with tuples, keep these methods in mind to enhance your programming skills and improve your overall coding experience.
FAQ
-
How do I check if two tuples are equal?
You can use the equality operator (==
) to check if two tuples are equal. If they have the same elements in the same order, it returnsTrue
. -
Can I compare tuples with different lengths?
Yes, Python compares tuples lexicographically. If the elements are equal up to the length of the shorter tuple, the shorter tuple is considered less than the longer one. -
What happens if I compare tuples with different data types?
Python will raise aTypeError
if you try to compare tuples containing incompatible data types. -
Is it possible to compare tuples containing nested tuples?
Yes, you can compare tuples containing nested tuples using the same comparison operators. Python will compare them element by element. -
What is the best way to compare large tuples?
For large tuples, using theall()
function with a generator expression is an efficient way to compare elements one by one.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn