Python Threading Queue
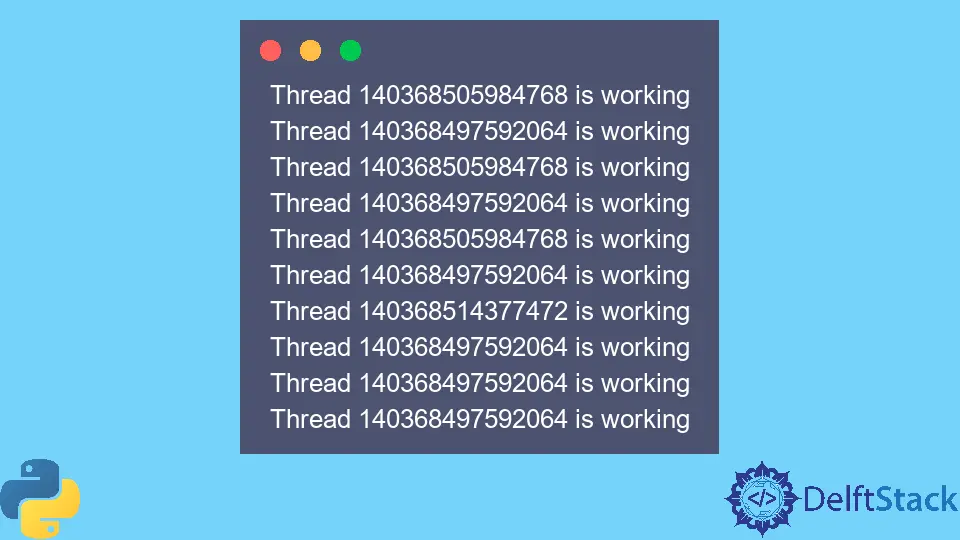
This tutorial will discuss limiting the number of active threads in Python.
Threads in Python
Threading in Python allows multiple threads of execution to run simultaneously within a single process. Each thread runs independently of the others, allowing for concurrent execution and improved performance.
Threading is particularly useful for executing tasks that are I/O bound or have a long execution time, as it allows other threads to continue executing while one thread is blocked.
Python provides a built-in threading
module for creating and managing threads. The Thread
class within the module can be used to create a new thread of execution, and the start()
method can be used to begin the execution of the thread.
The join()
method can be used to wait for a thread to finish its execution.
Besides the threading
module, Python also provides the concurrent.futures
module, which provides a higher-level interface for asynchronously executing callables. This module provides the ThreadPoolExecutor
class, which can be used to create a pool of worker threads that can be used to execute callables in parallel.
Threading can be a powerful tool for improving the performance of Python programs, but it should be used with caution, as it can also introduce complexity and the potential for race conditions and other synchronization issues.
Limit Threads with a Queue in Python
The Queue
class in Python provides a thread-safe and FIFO (first-in, first-out) data structure for storing items that need to be processed by multiple threads. It can be used to coordinate the flow of data between threads and to limit the number of threads that can be executed at the same time.
If we want to limit the number of threads that can be executed simultaneously using a queue, we can use the maxsize
parameter inside the Queue
class. Here’s an example of how to use a queue to limit the total number of threads.
import threading
import queue
from queue import Queue
from threading import Thread
def function_to_be_executed():
print(f"Thread {threading.get_ident()} is working")
return
q = Queue(maxsize=3)
for i in range(10):
try:
thread = Thread(target=function_to_be_executed)
q.put(thread, block=False)
except queue.Full:
q.get().join(timeout=0)
thread = Thread(target=function_to_be_executed)
q.put(thread, block=False)
thread = q.get()
thread.start()
while not q.empty():
thread = q.get()
thread.join(timeout=1)
Output:
Thread 140368505984768 is working
Thread 140368497592064 is working
Thread 140368505984768 is working
Thread 140368497592064 is working
Thread 140368505984768 is working
Thread 140368497592064 is working
Thread 140368514377472 is working
Thread 140368497592064 is working
Thread 140368497592064 is working
Thread 140368497592064 is working
The above code creates a Queue
object with a maximum size of 3, which means that only 3 threads can be in the queue at any given time. Then, 10 threads are created and added to the queue using the put()
method.
The while
loop starts the threads in the queue one by one, and the join()
method is used to wait for all threads to finish.
In this example, only 3 threads are running simultaneously; the rest will wait in the queue until they get a chance to run. We can adjust the maximum size of the queue and the number of threads to suit our own needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn