How to Join Threads in Python
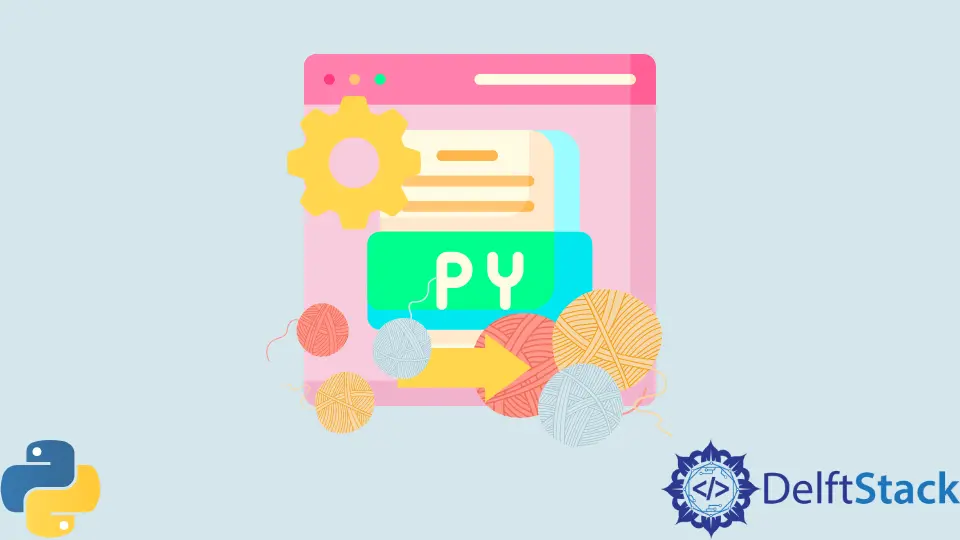
Multithreading enables us to get complete CPU optimization.
Threads do not require excess memory overhead, and multiple threads can also communicate and share information. In Python, we use the threading
module to work with threads.
We will now discuss the join()
method with threads in Python. We use this function to block the calling thread until the thread on it is terminated.
It can normally terminate or due to some exception and timeout. The timeout value can be mentioned in the join()
function also if required.
Let us now discuss this with an example.
import threading
import time
class sample(threading.Thread):
def __init__(self, time):
super(sample, self).__init__()
self.time = time
self.start()
def run(self):
print(self.time, " starts")
for i in range(0, self.time):
time.sleep(1)
print(self.time, "has finished")
t3 = sample(3)
t2 = sample(2)
t1 = sample(1)
t3.join()
print("t3.join() has finished")
t2.join()
print("t2.join() has finished")
t1.join()
print("t1.join() has finished")
Output:
3 starts
2 starts
1 starts
1 has finished
2 has finished
3 has finished
t3.join() has finished
t2.join() has finished
t1.join() has finished
In the above example, note that the other two threads are over when t3
finishes. However, the join()
function holds the main thread, and the other threads wait for it to terminate.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn