Daemon Threads in Python
-
Set the
daemon
Parameter to Create a Daemon Thread in Python -
Use the
setDaemon()
Function to Change a Thread to a Daemon Thread in Python
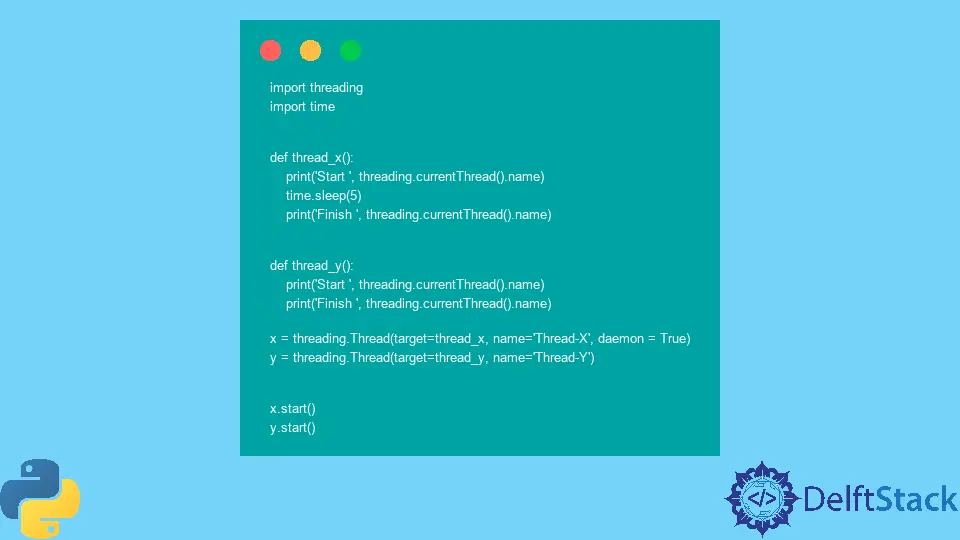
Threads are a small sequence of instructions to be processed, and we can have different threads running concurrently to increase efficiency. We have daemon threads and non-daemon threads.
This tutorial will discuss daemon threads in Python.
Set the daemon
Parameter to Create a Daemon Thread in Python
The threads which support the non-daemon and the main thread are called the daemon threads. They do not block the main thread from exiting. non-daemon threads may also execute after the main thread’s execution, but Daemon threads stop execution with the main thread.
It is why daemon threads act as good support to the main and non-daemon threads. We use daemon threads to perform basic functions like garbage collection, which will stop when the main program terminates, regardless of whether the process is completed or not.
To create a daemon thread, we set the threading.Thread()
function’s daemon
parameter as True
.
For example:
import threading
import time
def thread_x():
print("Start ", threading.currentThread().name)
time.sleep(5)
print("Finish ", threading.currentThread().name)
def thread_y():
print("Start ", threading.currentThread().name)
print("Finish ", threading.currentThread().name)
x = threading.Thread(target=thread_x, name="Thread-X", daemon=True)
y = threading.Thread(target=thread_y, name="Thread-Y")
x.start()
y.start()
Output:
Start Thread-X
Start Thread-Y
Finish Thread-Y
In the example, we can observe that thread x
, a daemon thread, stops execution when the non-daemon thread y
stops executing and the program terminates.
Use the setDaemon()
Function to Change a Thread to a Daemon Thread in Python
We can also use the setDaemon()
function to change a thread and make it a daemon thread. We need to pass True
as a parameter with this function.
The isDaemon()
function will return True
if a thread is a daemon thread; otherwise, it will return false
.
We will now use these methods in the following example.
import threading
import time
def thread_x():
print("Start ", threading.currentThread().name)
time.sleep(5)
print("Finish ", threading.currentThread().name)
def thread_y():
print("Start ", threading.currentThread().name)
print("Finish ", threading.currentThread().name)
x = threading.Thread(target=thread_x, name="Thread-X")
y = threading.Thread(target=thread_y, name="Thread-Y")
x.setDaemon(True)
print(x.isDaemon())
print(y.isDaemon())
x.start()
y.start()
Output:
True
False
Start Thread-X
Start Thread-Y
Finish Thread-Y
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn