How to Kill a Python Thread
- Understanding Python Thread and Their Behavior
- Create/Reset a Stop Flag to Kill a Thread in Python
-
Use
trace
to Kill a Thread in Python - Set the Given Thread as a Daemon Thread to Kill a Thread in Python
-
Use the
multiprocessing
Module to Kill a Thread in Python -
Use the Hidden
_stop()
Function to Kill a Thread in Python - Raise Exceptions in a Thread to Kill a Thread in Python
- Best Practices for Thread Termination in Python
- Faq
- Conclusion
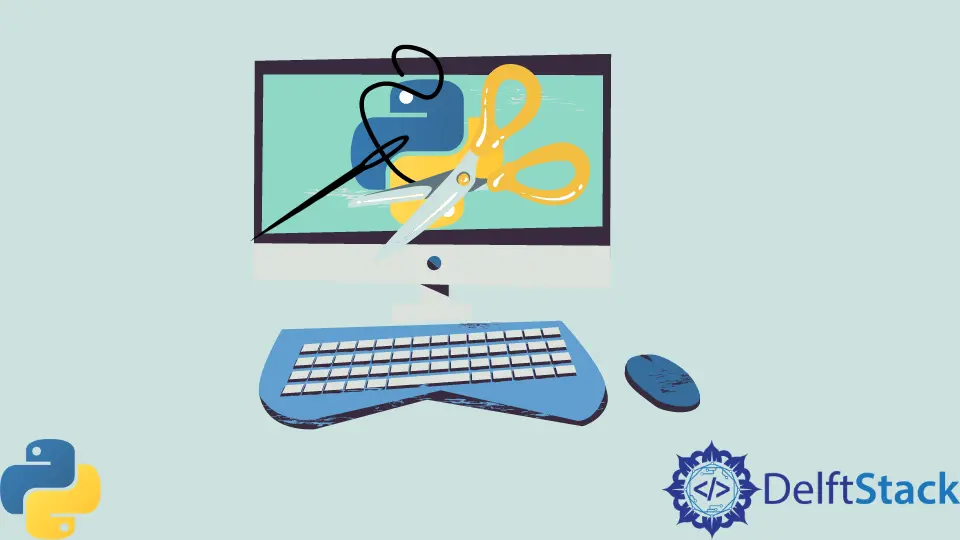
Killing a thread in Python is generally considered bad programming practice due to potential issues it can introduce. While it might be necessary in some cases, abruptly terminating a thread can leave tasks unfinished in the background, leading to problems such as resource leaks.
Notably, Python lacks a direct mechanism for terminating threads, requiring developers to employ alternative methods. This tutorial explores various approaches to terminate threads in Python, highlighting the challenges associated with such actions.
Understanding Python Thread and Their Behavior
Python threads offer a means of concurrent execution, allowing developers to perform multiple tasks simultaneously. Key concepts include:
1. Thread Creation:
- Create threads with custom function using
threading.Thread
and override therun
method for custom behavior.
2. Global Interpreter Lock (GIL):
- The GIL permits only one thread to execute Python bytecode at a time, limiting true parallelism.
3. Concurrency vs. Parallelism:
- Threads provide concurrent execution, but GIL hinders true parallelism for CPU-bound tasks.
4. Thread Safety:
- Ensure thread safety using locks (e.g.,
threading.Lock
) for shared resource access.
5. Daemon Threads:
- Flag threads as daemons for automatic termination on main program exit.
6. Thread Termination:
- Gracefully terminate threads using flags, events, or synchronization mechanisms.
7. Thread Communication:
- Threads communicate via queues, shared variables, or synchronization primitives.
Understanding these concepts is vital for effective thread utilization, considering GIL impact and synchronization needs. Alternative approaches like multiprocessing or asynchronous programming may be explored based on task requirements.
Now, we will focus on and explain the several ways we can kill a thread in Python.
Create/Reset a Stop Flag to Kill a Thread in Python
A stop flag can be declared in the code, which will make it stop the thread’s execution when encountered by the thread. This flag serves as a signal for the thread to cease execution when encountered.
The following code creates a stop flag to kill a thread in Python.
Code Example:
import threading
import time
def frun():
while True:
print("thread running")
global stop_threads
if stop_threads:
break
stop_threads = False
x = threading.Thread(target=frun)
x.start()
time.sleep(1)
stop_threads = True
x.join()
print("killed the thread.")
In this code example, we efficiently manage to kill a thread using a stop flag. The frun()
function, representing the thread’s primary logic, continuously prints "thread running"
within its infinite loop.
The global variable stop_threads
serves as our stop flag, initially set to False
to enable uninterrupted thread execution. After starting the thread with threading.Thread
and introducing a brief delay, we set the stop flag to True
, signaling the thread to exit its loop gracefully.
The x.join()
method ensures we wait for the thread to complete, and the print
statement "killed the thread"
signifies successful termination all the threads. This method provides a concise and effective way to control thread execution in Python.
Output:
The output will include several repetitions of "thread running"
until the stop_threads
flag is set to True
. Once the flag is set, the thread breaks out of the loop, and the program prints "killed the thread."
.
The actual number of "thread running"
lines printed may vary depending on the timing of the flag change and the thread execution.
Use trace
to Kill a Thread in Python
Another approach to kill a thread in Python involves utilizing the trace module to set traces on specific events during the execution of Python code, such as line executions, function calls, or exceptions.
In the context of ending a thread, the trace
module can introduce a trace that triggers a specific action, like raising an exception, when a particular condition is met. This action effectively interrupts the thread’s execution, leading to its termination.
The following example uses traces to kill a thread in Python.
Code Example:
import sys
import time
import threading
import trace
class KThread(threading.Thread):
def __init__(self, *args, **keywords):
threading.Thread.__init__(self, *args, **keywords)
self.killed = False
def start(self):
self.__run_backup = self.run
self.run = self.__run
threading.Thread.start(self)
def __run(self):
sys.settrace(self.globaltrace)
self.__run_backup()
self.run = self.__run_backup
def globaltrace(self, frame, why, arg):
if why == "call":
return self.localtrace
else:
return None
def localtrace(self, frame, why, arg):
if self.killed:
if why == "line":
raise SystemExit()
return self.localtrace
def kill(self):
self.killed = True
def exfu():
print("The function begins")
for i in range(1, 100):
print(i)
time.sleep(0.2)
print("The function ends")
x = KThread(target=exfu)
x.start()
time.sleep(1)
x.kill()
In this code example, the KThread
class is introduced, which modifies the behavior of a thread. While it does not directly subclass the standard threading.Thread
class, it overrides its start
and run
methods.
The custom thread class incorporates a kill()
method to set the killed attribute and a modified run
method that utilizes Python’s sys.settrace
to alter the thread’s behavior.
A globaltrace
function is employed to determine when to activate the localtrace function, which, when triggered, raises a SystemExit
exception to effectively kill the thread.
Output:
The output shows "The function begins"
as the initial message when the exfu
function is executed within the KThread
instance. The subsequent numbers 1 through 5 are printed in the loop with a short delay.
However, the thread is deliberately terminated using the kill
method, which triggers a trace mechanism to raise a SystemExit
exception. As a result, the loop is abruptly interrupted after printing the first five numbers, and the program concludes without reaching the "The function ends"
message.
Keep in mind that using signals and traces for thread termination can have complexities and may not work seamlessly in all situations. It’s essential to thoroughly test and understand the implications of such an approach in your specific use case.
Set the Given Thread as a Daemon Thread to Kill a Thread in Python
Daemon threads are threads that automatically get killed when the main program is terminated. We can set a given thread as a daemon thread to kill the particular thread in Python.
The following code sets the given thread as a daemon thread to kill a thread in Python.
Code Example:
import threading
import time
import sys
def exfu():
while True:
time.sleep(0.5)
print("Thread alive, but it will die on program termination")
x = threading.Thread(target=exfu)
x.daemon = True
x.start()
time.sleep(2)
sys.exit()
In this code, we create a daemon thread named x
using the threading
module. The thread executes the exfu
function, which runs an infinite loop with a 0.5-second sleep interval and prints a message indicating its liveliness.
By setting x.daemon
to True
, we designate the thread as a daemon, allowing it to terminate abruptly when the main program exits. After starting the daemon thread, we introduce a 2-second delay using time.sleep(2)
and then invoke sys.exit()
to terminate the main program.
As a result, the daemon thread is forcefully terminated upon program termination, providing a concise way to manage the thread’s lifecycle in the context of the program.
Output:
The repeated appearance of the message "Thread alive, but it will die on program termination"
in the output reflects the behavior of the daemon thread (x
). The thread executes a loop in the exfu
function for a 2-second duration before the main program terminates.
Being a daemon thread, x
is promptly terminated along with the main program’s conclusion. The message repeats in the output, indicating the thread’s iterative execution within the defined time frame before termination.
Please note that daemon threads are abruptly terminated when the main program exits. If your thread requires any cleanup actions or if you want to ensure a more controlled termination, consider using a flag or event mechanism, as discussed in the previous examples.
Use the multiprocessing
Module to Kill a Thread in Python
The multiprocessing
module is imported, which facilitates the creation and management of parallel processes in Python.
It’s important to note that the multiprocessing
module is primarily designed for managing processes, not threads. Terminating processes using terminate()
is considered a relatively safer and less complex approach compared to terminating threads directly.
The following code uses the multiprocessing
module to kill a thread in Python.
Code Example:
import multiprocessing
import time
def cp():
while True:
for i in range(20):
print("Process: ", i)
time.sleep(0.05)
x = multiprocessing.Process(target=cp)
x.start()
time.sleep(0.5)
x.terminate()
print("Terminated the child process")
In this code example, we utilize the multiprocessing
module to manage parallel processes. We define a function cp
that represents the main logic of the process, involving an infinite loop where the process prints a message ("Process: i"
) 20 times with a slight delay using time.sleep(0.05)
between each iteration.
Next, we create an instance x
of the Process
class, specifying the target function as cp
. By calling x.start()
, we initiate the execution of the process.
To allow the process to run for a short duration, we introduce a delay of 0.5 seconds using time.sleep(0.5)
. Finally, we terminate the process abruptly with x.terminate()
.
This method forcefully ends the process and the subsequent print
statement, which indicates that the child process has been successfully terminated.
Output:
In the output, we can see the messages for the first ten iterations. The "Process: 0" to "Process: 9"
.
After a brief delay of 0.5 seconds, the main program abruptly kills the child process (x
) using the x.terminate()
method. This action forcefully ends the child process, and the termination message is printed ("Terminated the child process"
).
The termination of the child process is expected, as indicated by the use of x.terminate()
after a short delay. Keep in mind that abruptly killing of threads may lead to unexpected behavior, and proper synchronization mechanisms should be considered for more controlled terminations.
Use the Hidden _stop()
Function to Kill a Thread in Python
Although undocumented, a hidden _stop()
function can implement the task of killing a thread in Python. The following code uses the hidden _stop()
function to kill a thread in Python.
Code Example:
import time
import threading
class th1(threading.Thread):
def __init__(self, *args, **kwargs):
super(th1, self).__init__(*args, **kwargs)
self._stop_event = threading.Event()
def stop_thread(self):
self._stop_event.set()
def stopped(self):
return self._stop_event.isSet()
def run(self):
while True:
if self.stopped():
return
print("Hello, world!")
time.sleep(1)
x = th1()
x.start()
time.sleep(5)
x.stop_thread()
x.join()
In the code above, we have created a custom thread class, th1
, that extends the Thread
class from the threading
module. This class has methods to stop the thread (stop_thread()
) and check if it is stopped (stopped()
), implemented using a threading.Event
(_stop_event
).
We then create an instance x
of this thread
class and start it. The thread runs in a loop, printing "Hello, world!"
every second, but it continually checks whether it has been stopped using the stopped method, and if the thread is stopped, it returns, effectively ending its execution.
After starting the thread, we wait for 5 seconds using time.sleep(5)
, giving the thread time to print multiple "Hello, world!"
messages. We then stop the thread by calling the stop_thread()
method.
The join()
method is used to wait for the thread to complete its execution. In our case, since the thread checks for the stop condition repeatedly, it will exit gracefully when the stop_thread()
method is called.
The join()
call ensures that we wait for the thread to finish before moving on.
Output:
We can see in the output is a series of "Hello, world!"
messages printed by the thread during the 5-second sleep period. After calling stop_thread()
, the thread will exit, and the program will proceed, completing the execution of the main thread.
Using the hidden stop()
function to kill a thread is not recommended. The stop()
method is considered private and is not part of the public API for the threading module.
Using it directly may lead to unexpected behavior and is not guaranteed to work across different Python implementations or versions.
Raise Exceptions in a Thread to Kill a Thread in Python
Using PyThreadState_SetAsyncExc
for Exception-Based Termination
This method utilizes the PyThreadState_SetAsyncExc()
function, which raises an exception in the given thread asynchronously. The PyThreadState_SetAsyncExc()
function is a low-level Python C-API function that allows you to asynchronously raise an exception in a specific Python thread, and this function is part of the Python C-API, which provides a set of interfaces for integrating C code with Python.
Exception-based termination using PyThreadState_SetAsyncExc
in Python provides a mechanism to asynchronously raise an exception in a specific Python thread. While this method may be employed to terminate a thread, it is essential to understand its low-level nature and potential variations across different Python implementations.
Code Example:
import threading
import ctypes
import time
class Twe(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
try:
while True:
print("running " + self.name)
finally:
print("ended")
def get_id(self):
if hasattr(self, "_thread_id"):
return self._thread_id
for id, thread in threading._active.items():
if thread is self:
return id
def raise_exception(self):
thread_id = self.get_id()
resu = ctypes.pythonapi.PyThreadState_SetAsyncExc(
thread_id, ctypes.py_object(SystemExit)
)
if resu > 1:
ctypes.pythonapi.PyThreadState_SetAsyncExc(thread_id, 0)
print("Exception raised successfully")
x = Twe("Thread A")
x.start()
time.sleep(2)
x.raise_exception()
x.join()
Output:
In this first example, the PyThreadState_SetAsyncExc
method is used to raise a SystemExit
exception in the thread, attempting to terminate its execution. This method is considered less reliable and may not work consistently across different Python implementations due to its low-level nature and platform-specific behavior.
The raise_exception
method attempts to terminate the thread using this approach, but as we can see in the output, the message "running Thread A"
prints continuously.
Using Thread-Safe Event
for Termination
Terminating a thread in Python often involves signaling the thread to gracefully exit its execution. One effective and thread-safe approach is using an event mechanism.
Code Example:
import threading
import time
class Twe(threading.Thread):
def __init__(self, name, stop_event):
threading.Thread.__init__(self)
self.name = name
self.stop_event = stop_event
def run(self):
try:
while not self.stop_event.is_set():
print("running " + self.name)
finally:
print("ended")
def raise_exception(self):
print("Terminating the thread...")
self.stop_event.set()
stop_event = threading.Event()
x = Twe("Thread A", stop_event)
x.start()
time.sleep(2)
x.raise_exception()
x.join()
Output:
In this second code example, a thread-safe Event
object (stop_event
) is used to signal the termination of the thread. The run
method of the thread continuously checks whether the event is set and terminates its execution if the event is set.
The raise_exception
method sets the event, indicating to the thread that it should terminate gracefully.
the Difference Between Using Thread-Safe Event
and PyThreadState_SetAsyncExc
for Exception-Based Termination
In the initial code example, our endeavor to terminate the thread involves raising a SystemExit
exception using the PyThreadState_SetAsyncExc
method.
However, this method is deemed less reliable and may exhibit inconsistent behavior across various Python implementations. Consequently, it is not the preferred approach when compared to using events or flags for thread termination.
In the subsequent code example, we adopt a more reliable and portable strategy by employing a thread-safe Event
to signal termination. This method stands out as a best practice for controlling thread termination in Python.
By utilizing events, we ensure a robust and consistent means of signaling threads to gracefully conclude their execution. This approach enhances the maintainability and compatibility of thread termination mechanisms in Python applications.
Best Practices for Thread Termination in Python
While understanding various methods to terminate threads in Python, it is crucial to emphasize best practices to ensure clean and controlled thread termination. Here are some recommendations:
Graceful Termination With Flags or Events
:
Prefer using flags or events to signal thread termination. This allows threads to gracefully exit their execution loop and perform necessary cleanup tasks.
Avoid Abrupt Termination:
Whenever possible, avoid abruptly terminating threads. Abrupt termination can lead to resource leaks and unexpected behavior.
Instead, use mechanisms like flags or events to give threads an opportunity to complete their tasks.
Thread-Safe Data Access:
Ensure thread safety when accessing shared data. Use synchronization mechanisms such as locks (e.g., threading.Lock
) to prevent data corruption when multiple threads are involved.
Cleanup Actions:
If your threads perform tasks that require cleanup (closing files, releasing resources), implement these cleanup actions in a controlled manner before thread termination.
Use Daemon Threads Wisely:
Daemon threads are automatically terminated when the main program exits. While they provide a convenient way for background tasks, be cautious if cleanup actions are necessary, as daemon threads do not wait for these actions to complete.
Consider Alternatives:
Evaluate whether using threads is the most suitable approach for your task. Depending on the nature of your application, alternatives like multiprocessing
or asynchronous programming may offer better solutions.
Exception Handling:
Implement proper exception handling within threads to handle unexpected situations gracefully. This includes catching exceptions and taking appropriate actions rather than allowing threads to crash abruptly.
Avoid Low-Level Termination Methods:
Minimize the use of low-level methods like PyThreadState_SetAsyncExc
for exception-based termination. These methods can have platform-specific behavior and may not work consistently across different Python implementations.
By following these best practices, you can enhance the reliability and maintainability of your multithreaded Python applications. Thread termination should be approached with caution, and a well-thought-out strategy ensures that your application remains robust and free from unexpected issues related to thread management.
Faq
Q-1 Why Might Killing a Thread in Python Be Necessary, and What Challenges Does It Pose?
A-1: While killing a thread is generally discouraged as a programming practice, there are situations where it becomes necessary. The article emphasizes the challenges associated with abruptly ending a thread, such as leaving tasks open in the background, potentially causing problems.
Python lacks a direct method to kill a thread abruptly, requiring developers to employ alternative methods.
Q-2 Why Is Abruptly Terminating a Python Thread Considered Bad Practice?
A-2: Abruptly terminating a thread is generally discouraged due to potential issues it can introduce, such as leaving tasks unfinished and resource leaks. Python lacks a direct mechanism to kill threads, emphasizing the importance of using alternative methods like flags or daemon threads for more controlled termination.
Q-3 How Does Setting a Thread as a Daemon Differ From Using Flags or Events for Termination?
A-3: Setting a thread as a daemon allows it to automatically terminate when the main program exits. However, daemon threads are abruptly terminated, and if cleanup actions are needed, using flags or events provides a more controlled and customizable approach to manage thread lifecycles.
Conclusion
In conclusion, terminating threads in Python demands careful consideration to avoid potential issues associated with abrupt termination. While generally discouraged, there are several methods available for terminating threads when necessary.
Understanding key concepts such as thread creation, the Global Interpreter Lock (GIL), concurrency, and thread safety is crucial. Various methods, including flag-based termination, trace-based termination, daemon threads, multiprocessing
, and event-based termination, were explored.
Best practices emphasize graceful termination, avoiding abrupt termination, ensuring thread-safe data access, implementing cleanup actions, using daemon threads wisely, considering alternatives, and handling exceptions properly. Adherence to these practices enhances the reliability and maintainability of multithreaded Python applications, ensuring effective thread management.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn