Python sys.settrace() Method
-
Syntax of Python
sys.settrace()
Method -
Example Codes: Use the
sys.settrace()
Method in Python -
Example Codes: Call a Loop Function Recursively Using the
sys.settrace()
Method
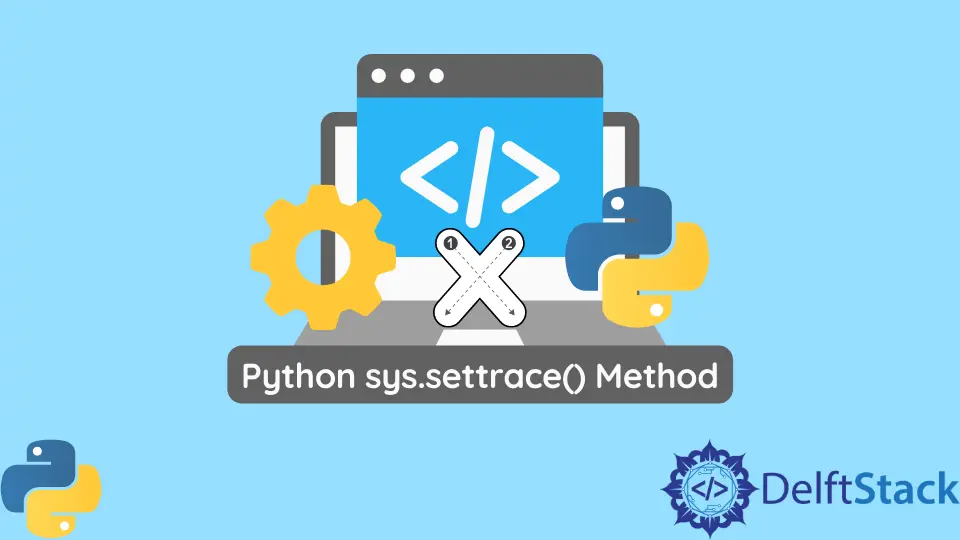
Python sys.settrace()
method is an efficient way of registering the traceback to the platform’s Python interpreter. A traceback call is the information returned when an event occurs in the code.
Syntax of Python sys.settrace()
Method
sys.settrace(frame, event, arg)
Parameters
frame |
It represents the current stack frame. |
event |
Keywords of string data type, such as call , line , return , exception , or opcode. The events have the following meaning.call : A function or a block of code is called.line : A line of code or a loop is about to be executed.return : A function or a block of code is returned.exception : An exception has occurred.opcode : A new opcode is to be executed by the interpreter. |
arg |
The type of event that has occurred. The events have the following arguments.return : The value to be returned or none.exception : The argument is a tuple consisting of exception , value , and traceback . |
Return
The return type of this method is a reference to the local trace function, which returns a reference to itself after execution.
Example Codes: Use the sys.settrace()
Method in Python
from sys import settrace
def tracer(frame, event, arg=None):
code = frame.f_code
func_name = code.co_name
line_no = frame.f_lineno
print(
f"A {event} has encountered in\
{func_name}() at the following line number {line_no}. "
)
return tracer
def function():
return "Python"
def system():
return function()
settrace(tracer)
system()
Output:
A call has encountered in system() at the following line number 19.
A line has encountered in system() at the following line number 20.
A call has encountered in function() at the following line number 16.
A line has encountered in function() at the following line number 17.
A return has encountered in function() at the following line number 17.
A return has encountered in system() at the following line number 20.
We used global trace functions in the above code to implement the local trace function, which returns itself.
Example Codes: Call a Loop Function Recursively Using the sys.settrace()
Method
import sys
from sys import settrace
def trace(frame, event, arg):
code = frame.f_locals["a"]
if code % 2 == 0:
frame.f_locals["a"] = code
def f(a):
print(a)
if __name__ == "__main__":
sys.settrace(trace)
for x in range(0, 10):
f(x)
Output:
0
1
2
3
4
5
6
7
8
9
In the above code, we modified the arguments sent to a function. The function uses a for
loop and counts the digit in a specified range.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn