How to Swap Elements of a List in Python
- Use the Assignment Operator With Tuple Unpacking to Swap Elements of a List in Python
- Use a Temporary Variable to Swap Elements of a List in Python
-
Use the
pop()
andinsert()
Functions to Swap Elements of a List in Python - Use the List Slicing Feature to Swap Elements of a List in Python
- Conclusion
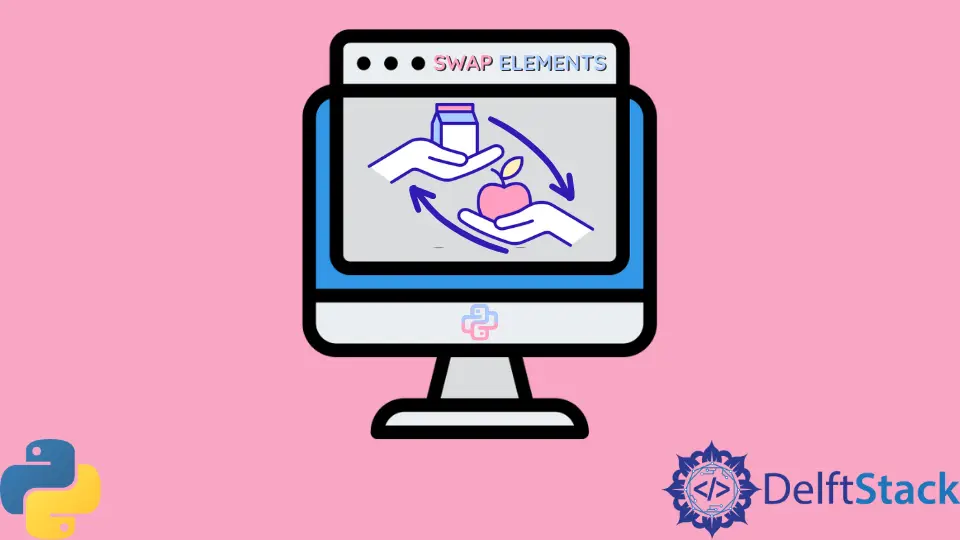
Swapping elements in a Python list refers to the process of interchanging the positions or values of two elements within the list. This can be useful in various situations, such as reordering elements, sorting algorithms, or making specific modifications to the list.
In this article, we will look at the different ways to swap the elements of a list.
Use the Assignment Operator With Tuple Unpacking to Swap Elements of a List in Python
One of the easiest and most commonly used methods to swap a list of elements is through the assignment operator with tuple unpacking.
Using the assignment operator with tuple unpacking is like a shortcut in Python. It helps you quickly swap values between variables or elements in a list, making your code shorter and easier to understand.
Instead of using a temporary variable, you can directly switch values in just one line of code. This technique is commonly employed for tasks like swapping values between variables or elements in a sequence, such as a list.
Syntax:
variable1, variable2 = value2, value1
In the syntax, the variable1
and variable2
are the variables on the left side of the assignment operator. The value2
and value1
are the values on the right side of the assignment operator.
The values on the right are packed into a tuple, and the assignment operator unpacks them into the corresponding variables on the left.
Example Code 1:
list = [6, 2, 7, 8]
print("list before swapping:", list)
list[1], list[3] = list[3], list[1]
print("list after swapping:", list)
Output:
list before swapping: [6, 2, 7, 8]
list after swapping: [6, 8, 7, 2]
In the code above, we begin with the list [6, 2, 7, 8]
. We print the original list using print("list before swapping:", list)
, and the output at this stage is list before swapping: [6, 2, 7, 8]
.
We then employ the assignment operator with tuple unpacking to swap the values of elements at indices 1
and 3
. The line list[1], list[3] = list[3], list[1]
accomplishes this in a single step.
Finally, we print the modified list with print("list after swapping:", list)
, resulting in the output list after swapping: [6, 8, 7, 2]
.
Using the assignment operator, we can swap the values of only two variables at a time. If we want to exchange multiple values, we can do it using a loop.
Let’s look at the following example.
Example Code 2:
list = [
6,
2,
7,
8,
5,
9,
10,
3,
]
print("list before swapping:", list)
for i, j in [(0, 3), (4, 6)]:
list[i], list[j] = list[j], list[i]
print("list after swapping:", list)
Output:
list before swapping: [6, 2, 7, 8, 5, 9, 10, 3]
list after swapping: [8, 2, 7, 6, 10, 9, 5, 3]
In this code, we begin with a list: [6, 2, 7, 8, 5, 9, 10, 3]
. Before swapping, we print the original list: list before swapping: [6, 2, 7, 8, 5, 9, 10, 3]
.
Using a loop, we iterate through pairs of indices [(0, 3), (4, 6)]
and swap the corresponding values. For instance, in the first iteration, we swap values at indices 0
and 3
.
After the loop, we print the modified list: list after swapping: [8, 2, 7, 6, 10, 9, 5, 3]
.
Use a Temporary Variable to Swap Elements of a List in Python
Using a temporary variable in programming in the context of swapping elements in a list involves employing an additional variable to temporarily store the value of one element before another overwrites it. This approach is commonly used when you need to exchange the values of two variables or elements.
Example Code:
list = [6, 2, 7, 8]
print("list before swapping:", list)
temp = list[1]
list[1] = list[3]
list[3] = temp
print("list after swapping:", list)
Output:
list before swapping: [6, 2, 7, 8]
list after swapping: [6, 8, 7, 2]
In the code, we begin with the list [6, 2, 7, 8]
. Before swapping, we print the original list: list before swapping: [6, 2, 7, 8]
.
Using a temporary variable(temp
), we swap the values at indices 1
and 3
. We assign the value at index 1
to temp
, replace the value at index 1
with the value at index 3
, and then assign the stored value of temp
to index 3
.
The list is then printed after swapping: list after swapping: [6, 8, 7, 2]
.
Use the pop()
and insert()
Functions to Swap Elements of a List in Python
Using the pop()
and insert()
functions in Python is a straightforward approach to swapping elements within a list. The pop()
function is employed to remove elements from specific indices, and the insert()
function is then used to insert those elements back into the desired positions.
In the following code, we have popped two elements from the list using their index and stored the returned values into two variables.
Example Code:
list = [6, 2, 7, 8]
print("list before swapping:", list)
val1 = list.pop(1)
val2 = list.pop(2)
list.insert(1, val2)
list.insert(2, val1)
print("list after swapping:", list)
Output:
list before swapping: [6, 2, 7, 8]
list after swapping: [6, 8, 2, 7]
In this code, we start with a list [6, 2, 7, 8]
. To observe the original state, we print the list: list before swapping: [6, 2, 7, 8]
. Using the pop()
function, we remove elements at indices 1
and 2
, storing their values in val1
and val2
.
We then use insert()
to place these values back into the list at indices 1
and 2
, effectively swapping them. Finally, we print the modified list: list after swapping: [6, 8, 7, 2]
.
Use the List Slicing Feature to Swap Elements of a List in Python
List slicing is a feature in Python that allows you to extract a portion (or slice) of a list. It is achieved by specifying a range of indices within square brackets.
Syntax:
list[start:stop:step]
Parameters:
start
: The index from where the slicing begins (inclusive).stop
: The index where the slicing ends (exclusive).step
: An optional parameter that specifies the step or increment between indices.
Example Code:
my_list = [6, 2, 7, 8]
print("list before swapping:", my_list)
my_list[1:4] = my_list[3:0:-1]
print("list after swapping:", my_list)
Output:
list before swapping: [6, 2, 7, 8]
list after swapping: [6, 8, 7, 2]
In this code, we initialize a list my_list
with values [6, 2, 7, 8]
. To see the original state, we print the list: list before swapping: [6, 2, 7, 8]
.
Next, using a compact approach with list slicing, we swap the elements at indices 1
to 3
. The line my_list[1:4] = my_list[3:0:-1]
achieves this by replacing the targeted slice with a reversed sublist.
After the swap, we print the modified list: list after swapping: [6, 8, 7, 2]
.
Conclusion
This article explored various methods to swap elements in a Python list. We covered the use of the assignment operator with tuple unpacking, providing a concise and clear way to interchange values within a list.
Additionally, we delved into employing a temporary variable for swapping and demonstrated its practical application. The article also introduced the pop()
and insert()
functions, showcasing a straightforward approach to element swapping.
Lastly, the use of list slicing was explained as a powerful feature, exemplified by a concise code snippet for efficient element swapping. These techniques offer programmers versatile options to manipulate lists, whether for reordering elements, sorting algorithms, or other list modifications.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python