How to Split List Into Sublists in Python
- Split List Into Sublists Using List Comprehensions and List Slicing in Python
-
Split List Into Sublists Using the
array_split()
Function in NumPy -
Split List Into Sublists Using the
itertools.groupby
Function - Split a List into Sublists in Python Using a Loop
- Conclusion
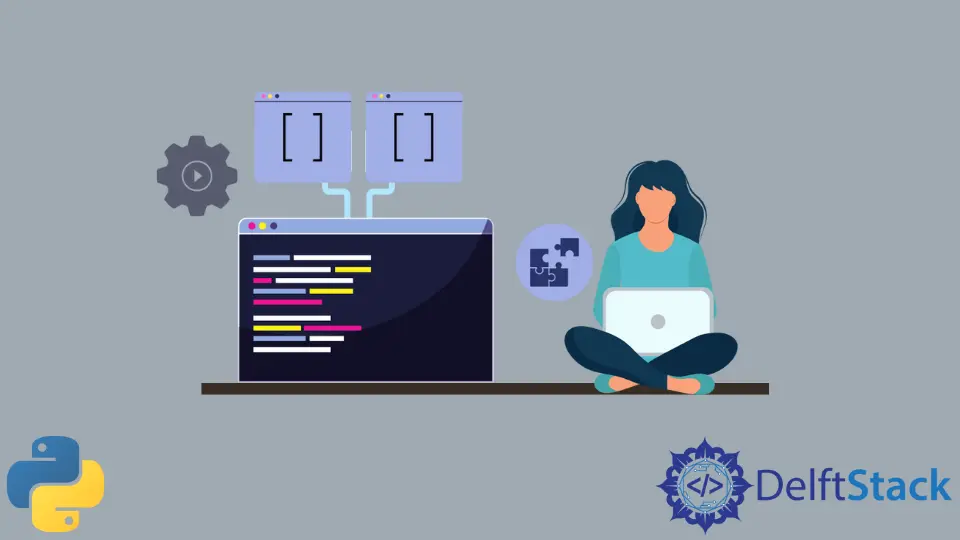
Splitting a list into sublists is a common and essential operation in Python programming. This article delves into various methods to accomplish this task efficiently.
From the simplicity of Python’s list comprehensions and loops to the power of NumPy and the versatility of the itertools.groupby
function, you’ll discover multiple approaches to suit your specific needs.
Split List Into Sublists Using List Comprehensions and List Slicing in Python
List comprehension is a concise and Pythonic way to create lists. It enables you to generate a new list by applying an expression to every element in an existing list while also providing filtering capabilities.
When it comes to splitting a list into sublists, list comprehension can be a powerful tool.
The fundamental idea behind splitting a list into sublists using list comprehension is to iterate through the original list and group elements into sublists of a specified size. Let’s break down the process into a few steps:
-
Determine the size of each sublist. This is often referred to as the chunk size and represents how many elements you want in each sublist.
-
Use list comprehension to iterate through the original list, creating sublists based on the chunk size.
-
The expression within the list comprehension will extract a slice of the original list for each sublist.
Now, let’s dive into the code and see how this can be accomplished.
Here’s a Python function that takes an input list and a chunk size as parameters and returns a list of sublists:
def split_list(input_list, chunk_size):
return [
input_list[i : i + chunk_size] for i in range(0, len(input_list), chunk_size)
]
Here, we define a function split_list
that takes two arguments: input_list
, the original list to be split, and chunk_size
, the desired size of each sublist.
Within the list comprehension, we then use a for
loop to iterate through the original list. The loop variable i
represents the starting index for each sublist.
We use range(0, len(input_list), chunk_size)
to create a range of starting indices separated by the specified chunk size.
For each starting index i
, we extract a slice of the original list from i
to i + chunk_size
. This slice represents one of the sublists.
The resulting sublists are collected in a new list, and finally, that list is returned.
Let’s see an example of how to use this function:
def split_list(input_list, chunk_size):
return [
input_list[i : i + chunk_size] for i in range(0, len(input_list), chunk_size)
]
mylist = [1, 2, 3, 4, 5, 6, 7, 8, 9]
chunk_size = 3
result = split_list(mylist, chunk_size)
print(result)
In this example, the mylist
is split into sublists of size 3
, resulting in the following output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
The beauty of using list comprehension to split a list into sublists is that it’s highly customizable. You can adjust the chunk size to control the size of the sublists.
Additionally, you can modify the expression within the list comprehension to apply more complex logic, such as filtering elements or grouping based on specific criteria.
For instance, you can split a list of numbers into sublists based on even and odd values:
def split_even_odd(input_list):
return [
[x for x in input_list if x % 2 == 0],
[x for x in input_list if x % 2 != 0],
]
mylist = [1, 2, 3, 4, 5, 6, 7, 8, 9]
result = split_even_odd(mylist)
print(result)
Output:
[[2, 4, 6, 8], [1, 3, 5, 7, 9]]
This function creates two sublists, one for even numbers and one for odd numbers, resulting in a partitioned list.
Split List Into Sublists Using the array_split()
Function in NumPy
NumPy is a popular library for numerical and scientific computing in Python. It is built around the ndarray
object, which is a multidimensional array that allows for efficient element-wise operations and advanced indexing.
While NumPy is typically used for working with numerical data, it can also be applied to general list manipulation tasks.
One of the main benefits of using NumPy for splitting lists into sublists is its efficiency. NumPy’s array operations are highly optimized, making it a great choice for handling large datasets.
Additionally, NumPy simplifies the code required to perform these tasks, resulting in clean and readable code.
To split a list into sublists using NumPy, you can take advantage of the numpy.array_split()
method. Here’s the detailed syntax of this method:
numpy.array_split(ary, indices_or_sections, axis=0)
ary
: This is the input array or list that you want to split into sublists. It can be a 1-D array, a list, or any iterable.indices_or_sections
: This parameter specifies how the array should be split. It can be an integer representing the number of equal-sized subarrays you want or a list of indices where the array should be split. For example, if you pass2
, the array will be split into two equal-sized subarrays. If you pass[2, 4]
, the array will be split at indices2
and4
.axis
(optional): This parameter is used to specify the axis along which the input array should be split. The default is0
, which means the array is split along its first axis (rows).
Let’s illustrate the usage of the numpy.array_split()
method with a practical example:
import numpy as np
def split_list(input_list, chunk_size):
return np.array_split(input_list, len(input_list) // chunk_size)
In this example:
-
We import NumPy with the statement
import numpy as np
. -
We define a function
split_list
that takes two arguments:input_list
, the original list to be split, andchunk_size
, the desired size of each sublist. -
Within the function, we use the
np.array_split()
method. It takes the input listinput_list
and divides it into subarrays based on the chunk sizechunk_size
. -
The function returns a list of subarrays, each containing the specified number of elements. These subarrays are the equivalent of sublists in the context of NumPy.
Let’s see an example of how to use this function:
import numpy as np
def split_list(input_list, chunk_size):
return np.array_split(input_list, len(input_list) // chunk_size)
mylist = [1, 2, 3, 4, 5, 6, 7, 8, 9]
chunk_size = 3
result = split_list(mylist, chunk_size)
print(result)
This example defines a function split_list
that uses NumPy to split a given list into chunks of a specified size. The example demonstrates splitting the list [1, 2, 3, 4, 5, 6, 7, 8, 9]
into chunks of size 3
.
Output:
[array([1, 2, 3]), array([4, 5, 6]), array([7, 8, 9])]
NumPy provides various functions for array manipulation and data processing, making it highly customizable and flexible. You can adjust the chunk size to control the size of the sublists, and you can use other NumPy functions for further data analysis and manipulation.
For example, you can use NumPy to perform mathematical operations on the subarrays, apply filtering criteria, or conduct statistical analysis on the data within each sublist. This flexibility makes NumPy a versatile tool for handling a wide range of data-splitting tasks.
Split List Into Sublists Using the itertools.groupby
Function
The groupby
function from the itertools
module allows you to group elements from an iterable based on a specified key function. This is incredibly useful for tasks like splitting a list into sublists where you want to group elements that share some common characteristic.
The groupby
function groups consecutive elements from the iterable that have the same key according to the key function you provide. It returns an iterator that yields pairs of the key and the corresponding group of elements.
This functionality makes it an elegant choice for dividing lists into sublists.
The syntax of the itertools.groupby
function is as follows:
itertools.groupby(iterable, key=None)
iterable
: This is the input iterable, such as a list, that you want to split into sublists.key
(optional): This is a key function that is applied to each element in the iterable to determine how the elements should be grouped. Ifkey
is not specified or isNone
, the elements are grouped based on their identity, meaning consecutive equal elements are grouped.
Let’s illustrate the usage of the itertools.groupby
function with a practical example:
import itertools
def split_list(input_list, key_func):
sorted_list = sorted(input_list, key=key_func)
return [list(group) for key, group in itertools.groupby(sorted_list, key_func)]
In this example:
-
We import the
itertools
module using the statementimport itertools
. -
We define a function called
split_list
, which takes two arguments:input_list
, the original list to be split, andkey_func
, the key function that determines how elements are grouped into sublists. -
Within the function, we utilize the
itertools.groupby
function. It takes the sorted version of theinput_list
and groups its elements based on the providedkey_func
. -
The function returns a list of sublists, with each sublist containing elements that share the same key according to the
key_func
.
Now, let’s see an example of how to use this function:
import itertools
def split_list(input_list, key_func):
sorted_list = sorted(input_list, key=key_func)
return [list(group) for key, group in itertools.groupby(sorted_list, key_func)]
mylist = [1, 2, 3, 4, 5, 6, 7, 8, 9]
def key_func(x):
return (x - 1) // 3
result = split_list(mylist, key_func)
print(result)
In this example, we use a key_func
lambda function to split the mylist
into sublists based on the integer division by 3
. The output will be as follows:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
The elements in the input list are grouped into sublists based on the results of the key function.
itertools.groupby
offers great flexibility, as you can define the key_func
to specify the criteria for grouping elements. You can use more complex key functions for more specific grouping requirements, allowing you to split lists into sublists based on various conditions.
Additionally, since itertools.groupby
is an iterator, it is memory-efficient and can be used with very large datasets without consuming excessive memory.
Split a List into Sublists in Python Using a Loop
One simple and effective way to split a list into sublists is by using a loop. The fundamental concept is to iterate through the original list and group elements into sublists based on specified criteria, such as a fixed chunk size.
Here’s the basic idea:
-
Determine the size of each sublist, often referred to as the chunk size. This defines how many elements you want in each sublist.
-
Use a loop, typically a
for
loop, to iterate through the original list, creating sublists based on the chunk size. -
Inside the loop, you extract a slice of the original list for each sublist.
Now, let’s see how this can be accomplished through code. Here’s a Python function that splits a list into sublists using a loop:
def split_list(input_list, chunk_size):
sublists = []
for i in range(0, len(input_list), chunk_size):
sublists.append(input_list[i : i + chunk_size])
return sublists
Let’s break down the code step by step:
-
We define a function
split_list
that takes two arguments:input_list
, the original list to be split, andchunk_size
, the desired size of each sublist. -
Within the function, we use a
for
loop to iterate through the original list. The loop variablei
represents the starting index for each sublist. We userange(0, len(input_list), chunk_size)
to create a range of starting indices separated by the specified chunk size. -
For each starting index
i
, we extract a slice of the original list fromi
toi + chunk_size
. This slice represents one of the sublists. -
The resulting sublists are collected in a new list, and that list is returned.
Let’s see an example of how to use this function:
def split_list(input_list, chunk_size):
sublists = []
for i in range(0, len(input_list), chunk_size):
sublists.append(input_list[i : i + chunk_size])
return sublists
mylist = [1, 2, 3, 4, 5, 6, 7, 8, 9]
chunk_size = 3
result = split_list(mylist, chunk_size)
print(result)
In this example, the mylist
is split into sublists of size 3
, resulting in the following output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
One of the advantages of using a loop to split a list into sublists is the flexibility it offers. You can easily adjust the chunk size to control the size of the sublists, and you can modify the loop to implement more complex logic, such as filtering elements or grouping based on specific criteria.
For example, you can split a list of items into sublists based on specific attributes, or you can create sublists with overlapping elements. The possibilities are endless, and you can tailor the code to suit your specific needs.
Conclusion
Throughout this article, we’ve explored a range of techniques and tools, each with its strengths and use cases.
List comprehensions and loops provide simplicity and customization, while NumPy offers efficiency and performance for large datasets. The itertools.groupby
function showcases elegance and adaptability for grouping elements based on specific criteria.
These methods cater to a diverse set of requirements, from basic chunking to advanced data processing. Whether you’re working with numerical data, applying complex criteria, or seeking a straightforward solution, this article has brought you the options available to efficiently divide your lists into manageable sublists, enhancing your data processing capabilities.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python