How to Sort List of Tuples in Python
-
Use the
list.sort()
Function to Sort List of Tuples in Python - Use the Bubble Sort Algorithm to Sort the List of Tuples in Python
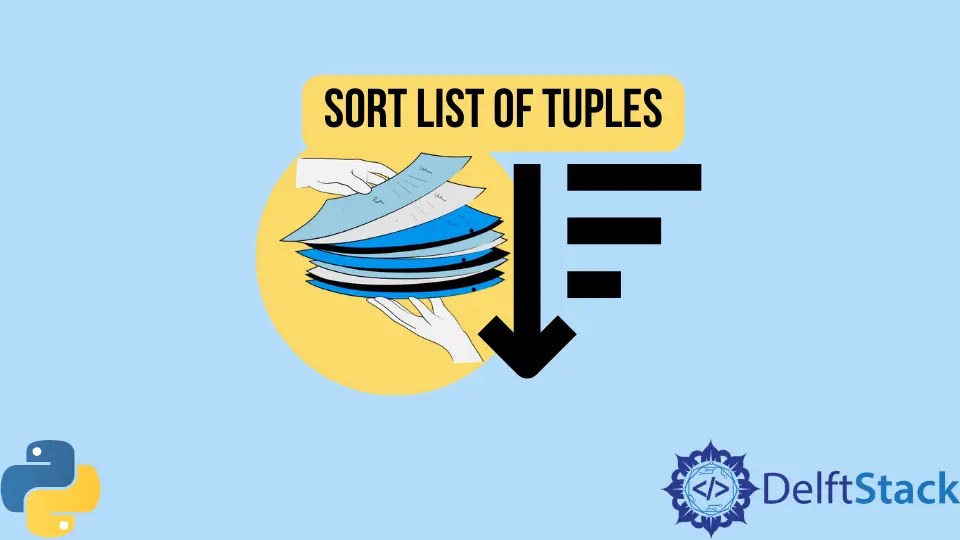
In Python, multiple items can be stored in a single variable using Tuples. A list of Tuples can be sorted like a list of integers.
This tutorial will discuss different methods to sort a list of tuples based on the first, second, or ith element in the tuples.
Use the list.sort()
Function to Sort List of Tuples in Python
The list.sort()
function sorts the elements of a list in ascending or descending order. Its key
parameter specifies the value to be used in the sorting. key
shall be a function or other callables that could be applied to each list element.
The following code sorts the tuples based on the second element in all the tuples.
list_students = [
("Vaibhhav", 86),
("Manav", 91),
("Rajesh", 88),
("Sam", 84),
("Richie", 89),
]
# sort by second element of tuple
list_students.sort(key=lambda x: x[1]) # index 1 means second element
print(list_students)
Output:
[('Sam',84), ('Vaibhhav',86), ('Rajesh',88), ('Richie',89), ('Manav',91)]
The order can be reversed to descending by setting the reverse
parameter of the sort()
method to be True
.
The following code sorts the list of tuples in descending order by using the reverse
parameter.
list_students = [
("Vaibhhav", 86),
("Manav", 91),
("Rajesh", 88),
("Sam", 84),
("Richie", 89),
]
# sort by second element of tuple
list_students.sort(key=lambda x: x[1], reverse=True)
print(list_students)
Output:
[('Manav',91), ('Richie',89), ('Rajesh',88), ('Vaibhhav',86), ('Sam',84)]
Use the Bubble Sort Algorithm to Sort the List of Tuples in Python
Bubble Sort is one of the simplest sorting algorithms; it works by swapping the adjacent elements in a list if they are in the wrong order and repeats this step until the list is sorted.
The following code sorts the tuples based on the second element and uses the bubble sort algorithm.
list_ = [("Vaibhhav", 86), ("Manav", 91), ("Rajesh", 88), ("Sam", 84), ("Richie", 89)]
# sort by second element of tuple
pos = 1
list_length = len(list_)
for i in range(0, list_length):
for j in range(0, list_length - i - 1):
if list_[j][pos] > list_[j + 1][pos]:
temp = list_[j]
list_[j] = list_[j + 1]
list_[j + 1] = temp
print(list_)
Output:
[('Sam',84), ('Vaibhhav',86), ('Rajesh',88), ('Richie',89), ('Manav',91)]
The pos
variable specifies the position by which sorting has to be carried out, which in this case, is the second element.
We can also use the first element to sort the list of tuples. The following program implements this.
list_ = [("Vaibhhav", 86), ("Manav", 91), ("Rajesh", 88), ("Sam", 84), ("Richie", 89)]
# sort by first element of tuple
pos = 0
list_length = len(list_)
for i in range(0, list_length):
for j in range(0, list_length - i - 1):
if list_[j][pos] > list_[j + 1][pos]:
temp = list_[j]
list_[j] = list_[j + 1]
list_[j + 1] = temp
print(list_)
Output:
[('Manav',91), ('Rajesh',88), ('Richie',89), ('Sam',84), ('Vaibhhav',86)]
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn