Python에서 튜플 정렬 목록
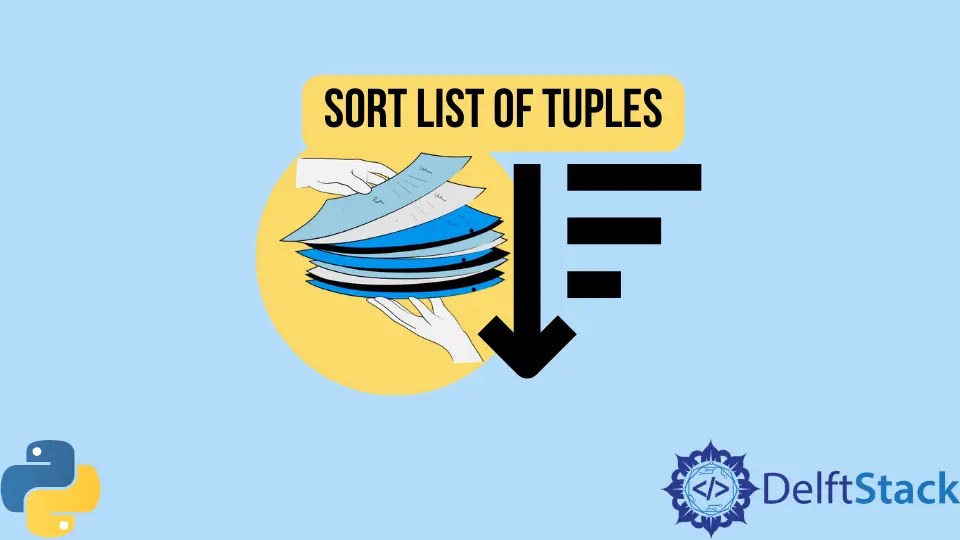
Python에서는 튜플을 사용하여 여러 항목을 단일 변수에 저장할 수 있습니다. 튜플 목록은 정수 목록처럼 정렬 할 수 있습니다.
이 튜토리얼에서는 튜플의 첫 번째, 두 번째 또는 i 번째 요소를 기반으로 튜플 목록을 정렬하는 다양한 방법에 대해 설명합니다.
list.sort()
함수를 사용하여 Python에서 튜플 목록을 정렬합니다
list.sort()
함수는 목록의 요소를 오름차순 또는 내림차순으로 정렬합니다. key
매개 변수는 정렬에 사용할 값을 지정합니다. key
는 각 목록 요소에 적용될 수있는 함수 또는 기타 콜 러블입니다.
다음 코드는 모든 튜플의 두 번째 요소를 기준으로 튜플을 정렬합니다.
list_students = [
("Vaibhhav", 86),
("Manav", 91),
("Rajesh", 88),
("Sam", 84),
("Richie", 89),
]
# sort by second element of tuple
list_students.sort(key=lambda x: x[1]) # index 1 means second element
print(list_students)
출력:
[('Sam',84), ('Vaibhhav',86), ('Rajesh',88), ('Richie',89), ('Manav',91)]
sort()
메소드의reverse
매개 변수를True
로 설정하여 내림차순으로 순서를 바꿀 수 있습니다.
다음 코드는reverse
매개 변수를 사용하여 내림차순으로 튜플 목록을 정렬합니다.
list_students = [
("Vaibhhav", 86),
("Manav", 91),
("Rajesh", 88),
("Sam", 84),
("Richie", 89),
]
# sort by second element of tuple
list_students.sort(key=lambda x: x[1], reverse=True)
print(list_students)
출력:
[('Manav',91), ('Richie',89), ('Rajesh',88), ('Vaibhhav',86), ('Sam',84)]
버블 정렬 알고리즘을 사용하여 Python에서 튜플 목록 정렬
거품 정렬는 가장 간단한 정렬 알고리즘 중 하나입니다. 순서가 잘못된 경우 목록에서 인접 요소를 교체하여 작동하며 목록이 정렬 될 때까지이 단계를 반복합니다.
다음 코드는 두 번째 요소를 기준으로 튜플을 정렬하고 버블 정렬 알고리즘을 사용합니다.
list_ = [("Vaibhhav", 86), ("Manav", 91), ("Rajesh", 88), ("Sam", 84), ("Richie", 89)]
# sort by second element of tuple
pos = 1
list_length = len(list_)
for i in range(0, list_length):
for j in range(0, list_length - i - 1):
if list_[j][pos] > list_[j + 1][pos]:
temp = list_[j]
list_[j] = list_[j + 1]
list_[j + 1] = temp
print(list_)
출력:
[('Sam',84), ('Vaibhhav',86), ('Rajesh',88), ('Richie',89), ('Manav',91)]
pos
변수는 정렬을 수행해야하는 위치를 지정합니다.이 경우 두 번째 요소입니다.
첫 번째 요소를 사용하여 튜플 목록을 정렬 할 수도 있습니다. 다음 프로그램이이를 구현합니다.
list_ = [("Vaibhhav", 86), ("Manav", 91), ("Rajesh", 88), ("Sam", 84), ("Richie", 89)]
# sort by first element of tuple
pos = 0
list_length = len(list_)
for i in range(0, list_length):
for j in range(0, list_length - i - 1):
if list_[j][pos] > list_[j + 1][pos]:
temp = list_[j]
list_[j] = list_[j + 1]
list_[j + 1] = temp
print(list_)
출력:
[('Manav',91), ('Rajesh',88), ('Richie',89), ('Sam',84), ('Vaibhhav',86)]
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn