How to Sort a List in Descending Order in Python
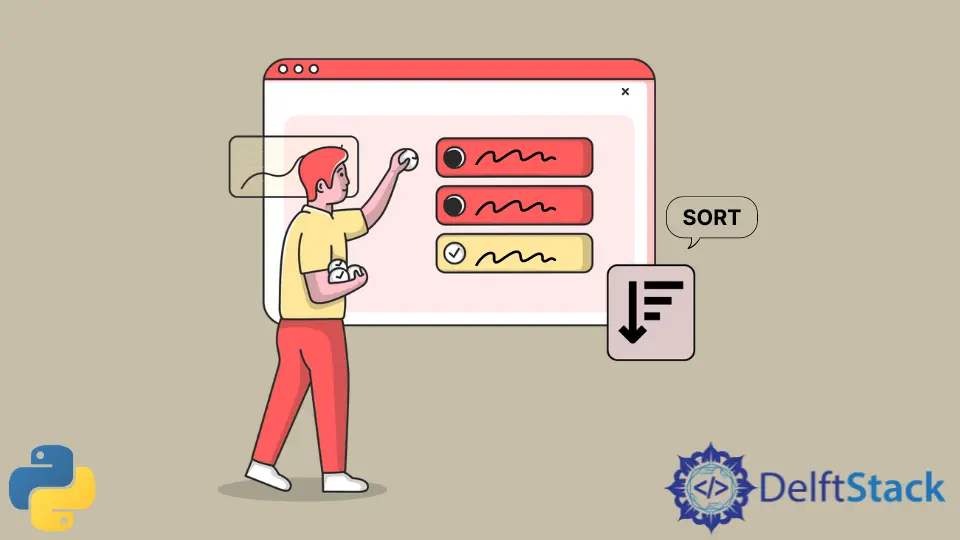
This tutorial demonstrates how to sort a list in descending order in Python.
Use the sort()
Method to Sort a List in Descending Order in Python
Python has a built-in function called sort()
, which, by default, arranges the list in ascending order. This method simply sorts the contents of the given list. It doesn’t have any required parameters, but it has optional parameters:
key
- determines which index or position is sorted inside a multidimensional array.reverse
- IfTrue
, the list is sorted in descending order.
Let’s see how this method sorts the list both with and without parameters:
- No parameters:
colors = ["pink", "blue", "black", "white"]
colors.sort()
print(colors)
Output:
['black', 'blue', 'pink', 'white']
The list orders strings based on their ASCII values which are the integer counterparts for a single character. If both strings in comparison have the same ASCII value, it then proceeds to compare the next characters of both strings until there isn’t anything left to compare.
- Using the
reverse
parameter:
colors = ["pink", "blue", "black", "white"]
colors.sort(reverse=True)
print(colors)
Output:
['white', 'pink', 'blue', 'black']
Setting the reverse
parameter to True
sorts a list in descending order.
Sorting integers and floating-point numbers are sorted based on which is greater and lesser. Let’s try it out with another example, this time sorting integers and decimals in descending order:
numbers = [55, 6, -0.05, 0.07, 2.5, -7, 2.99, 101, 0.78]
numbers.sort(reverse=True)
print(numbers)
Output:
[101, 55, 6, 2.99, 2.5, 0.78, 0.07, -0.05, -7]
From the output, sorting numbers using the sort()
function takes note of decimals as well as negative numbers.
This type of sorting also works on dates formatted like YYYY-MM-DD HH:MM:SS
. Let’s use a list of timestamps as an example to prove this.
timestamps = [
"2021-04-15 09:08:30",
"2021-04-14 08:09:38",
"2021-04-18 12:10:52",
"2021-04-21 23:39:22",
"2021-04-13 14:40:22",
"2021-04-14 13:59:46",
"2021-04-15 19:22:37",
"2021-04-18 07:00:58",
"2021-04-17 04:01:50",
"2021-04-22 01:17:13",
"2021-04-25 24:22:13",
"2021-04-14 25:36:38",
]
timestamps.sort(reverse=True)
print(timestamps)
Output:
['2021-04-25 24:22:13', '2021-04-22 01:17:13', '2021-04-21 23:39:22', '2021-04-18 12:10:52', '2021-04-18 07:00:58', '2021-04-17 04:01:50', '2021-04-15 19:22:37', '2021-04-15 09:08:30', '2021-04-14 25:36:38', '2021-04-14 13:59:46', '2021-04-14 08:09:38', '2021-04-13 14:40:22']
Observe that the output has successfully been sorted in descending order, confirming that timestamps can also be sorted properly by using the sort()
function with the reverse
parameter.
In summary, using the built-in sort()
function and setting the reverse
parameter to True
can be used to sort Python lists in descending order.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python