How to Save Python Variable to File
- Make Use of String Concatenation to Save a Variable to a File in Python
- Make Use of String Formatting to Save a Variable to a File in Python
-
Make Use of the
pickle
Library to Save a Variable to a File in Python -
Make Use of the
NumPy
Library to Save a Variable to a File in Python -
Make Use of the
JSON
Library to Save a Variable to a File in Python -
Make Use of the
csv
Library to Save a Variable to a File in Python - Conclusion
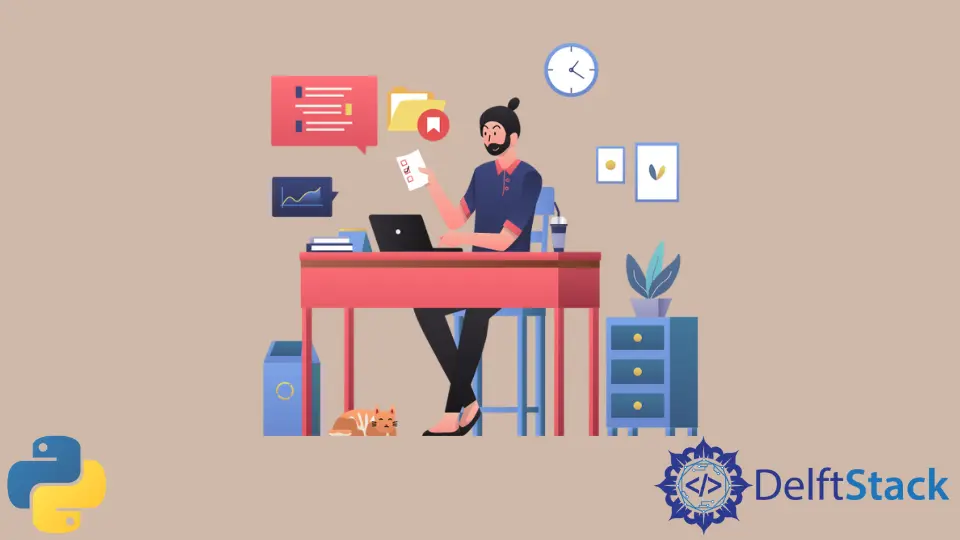
Python is perfectly capable of supporting file handling. It allows programmers to deal with files of different types, perform basic operations like reading and writing, and provide other file-handling options to operate on files.
This tutorial discusses the several methods available to save a variable to a file in Python.
We should note that we will use the open()
function with its mode set to w
in all the methods, which specifies that the given file is opened for writing.
Make Use of String Concatenation to Save a Variable to a File in Python
In Python, string concatenation involves combining strings using the +
operator. For this illustration, we’ll consider a basic scenario where a user’s name is stored in a variable, and we want to save it to a file.
Example:
# Define a variable
user_name = "JohnDoe"
# Save variable to file using string concatenation
file_name = "username.txt"
with open(file_name, "w") as file:
file.write("User Name: " + user_name)
We start by defining a variable user_name
and assigning it the value JohnDoe
. This variable represents the data we want to save.
Then, we specify the name of the file (username.txt
) where we want to store the variable. This enhances code readability and flexibility.
We write with concatenation using file.write("User Name: " + user_name)
line. Here, the +
operator concatenates the string User Name:
with the content of the user_name
variable.
If we examine the content of the username.txt
file after running this code, it would contain this output:
User Name: JohnDoe
The result is a string that combines the static text User Name:
with the actual user name, creating a meaningful representation in the file.
Make Use of String Formatting to Save a Variable to a File in Python
String formatting supplies a huge variety of customizations for the programmer to select from in the code. The %
sign is commonly referred to as the interpolation operator, which is utilized to implement string formatting.
Although there are other ways to implement string formatting in Python, the %
sign is the oldest and works pretty much on all available versions of Python, making it the most versatile out of the lot.
The %
sign, along with a letter representing the conversion type, is marked as a placeholder for the variable.
The following code uses string formatting to save a variable to a file in Python.
# Define a variable
user_name = "JohnDoe"
# Save variable to file using string formatting
file_name = "username.txt"
with open(file_name, "w") as file:
file.write("User Name: %s" % user_name)
We begin by defining a variable user_name
and assigning it the value JohnDoe
. This variable represents the data we want to save.
Then, we specify the name of the file (username.txt
) where we want to store the variable. This enhances code readability and provides flexibility.
File writing with string formatting is done using the file.write("User Name: %s" % user_name)
line. Here, %s
serves as a placeholder for the variable user_name
.
The %
operator, followed by the variable to be formatted (user_name
), inserts its value into the string.
If we examine the content of the username.txt
file after running this code, it would contain:
User Name: JohnDoe
The resulting string is a combination of the static text User Name:
and the actual user name, formatted using %s
.
Make Use of the pickle
Library to Save a Variable to a File in Python
The pickle
module can be utilized in Python to serialize and de-serialize any structure of an object. However, it can also be implemented to save a variable to a file in Python simply.
The pickle
module needs to be imported to the Python code to implement this method without any errors. This method is usually utilized when we need to store multiple variables in a file in Python.
The following code uses the pickle library to save a variable to a file in Python.
import pickle
# Define a variable
user_data = {"name": "John Doe", "age": 30, "city": "Example City"}
# Save variable to file using pickle
file_name = "userdata.pkl"
with open(file_name, "wb") as file:
pickle.dump(user_data, file)
with open(file_name, "rb") as file:
loaded_data = pickle.load(file)
print(loaded_data)
The first step is to import the pickle
module, which provides the necessary functions for serialization and deserialization.
We define a variable user_data
as a dictionary containing information about a user. This could represent any complex data structure.
Then we specify the name of the file (userdata.pkl
) where we want to store the variable. The file extension .pkl
is commonly used for pickle files.
We save the variable to a file using pickle.dump(user_data, file)
line. This line serializes the user_data
dictionary and writes it to the specified file.
The 'wb'
mode stands for write binary
, which is necessary when working with pickle files.
After running this code, a file named userdata.pkl
is created with the serialized data. If we were to examine the content of this file, it would contain a binary representation of the user_data
dictionary.
This would output:
{'name': 'John Doe', 'age': 30, 'city': 'Example City'}
The output is the user_data
dictionary stored in a binary format within the specified file.
Make Use of the NumPy
Library to Save a Variable to a File in Python
NumPy
, which is an abbreviation for Numerical Python, is a library that makes it possible to work with arrays and supplies several functions for fluent operation on these arrays. However, that is not all; it can also be utilized to save a variable to a file in Python.
We need to generate a list and save this list to the text file of the given name in the code. We will use the numpy.savetxt()
function to carry out this process.
The following code uses the NumPy
library to save a variable to a file in Python.
import numpy as np
# Define a NumPy array
numeric_data = np.array([1, 2, 3, 4, 5])
# Save NumPy array to file using savetxt
file_name = "numeric_data.txt"
np.savetxt(file_name, numeric_data)
The process starts with importing the numpy
library, often aliased as np
for brevity.
We define a NumPy array named numeric_data
containing numerical values. This array could represent any numerical data structure, such as a dataset or a mathematical sequence.
Then we specify the name of the file with numeric_data.txt
. This is the file to which we want to save the NumPy array.
We save the NumPy array to a file using np.savetxt(file_name, numeric_data)
. This function takes the file name and the NumPy array as arguments, and it saves the array to the specified text file.
After running this code, a file named numeric_data.txt
is created in the working directory. This file contains the numerical data represented by the NumPy array.
The content of the numeric_data.txt
file will look like:
1.000000000000000000e+00
2.000000000000000000e+00
3.000000000000000000e+00
4.000000000000000000e+00
5.000000000000000000e+00
Each line corresponds to an element of the NumPy array, and the values are written in scientific notation.
Make Use of the JSON
Library to Save a Variable to a File in Python
The json
module in Python facilitates the encoding and decoding of JSON data. Saving Python variables to a file in JSON format is advantageous when dealing with structured data, such as dictionaries or lists.
The following code uses the json
library to save a variable to a file in Python.
import json
# Define a Python dictionary
user_info = {"name": "John Doe", "age": 30, "city": "Example City"}
# Save dictionary to file using JSON
file_name = "user_info.json"
with open(file_name, "w") as file:
json.dump(user_info, file)
The process begins with importing the json
module, a standard library in Python.
Then, we define a Python dictionary named user_info
containing key-value pairs. This dictionary represents structured data that we want to save.
We specify the name of the file with user_info.json
. Using the .json
extension is a convention for JSON files.
Then we save the dictionary to a file using json.dump(user_info, file)
. This method takes the Python dictionary (user_info
) and writes its JSON representation to the specified file.
After running this code, a file named user_info.json
is created in the working directory. This file contains the JSON representation of the user_info
dictionary.
The resulting user_info.json
file contains the JSON representation of the dictionary:
{"name": "John Doe", "age": 30, "city": "Example City"}
The content of the file is a JSON-formatted string representing the key-value pairs of the original Python dictionary.
Make Use of the csv
Library to Save a Variable to a File in Python
CSV (Comma Separated Values) is a popular choice for this purpose, offering a straightforward way to represent tabular data. In Python, the csv
module provides functionality to work with CSV files, making it convenient to save and load variables.
The following code uses the csv
library to save a variable to a file in Python.
import csv
# Define a Python list representing a row of data
user_data = ["John Doe", 30, "Example City"]
# Save list to CSV file
file_name = "user_data.csv"
with open(file_name, "w", newline="") as file:
csv_writer = csv.writer(file)
csv_writer.writerow(user_data)
The process begins with importing the csv
module, a standard library in Python.
We define a Python list named user_data
, representing a row of data. This list can contain any combination of data types.
Then we specify The name of the file (user_data.csv
) is specified. Using the .csv
extension is a convention for CSV files.
We save the list to a CSV file using with open(file_name, 'w', newline='') as file
block. The csv.writer
is used to create a CSV writer object, and csv_writer.writerow(user_data)
writes the content of the user_data
list as a row in the CSV file.
After running this code, a file named user_data.csv
is created in the working directory. This file contains a single row of data representing the user_data
.
The resulting user_data.csv
file contains the following:
John Doe,30,Example City
The values in the list are separated by commas, conforming to the standard CSV format, where each comma-separated value represents a field in the row.
Conclusion
In Python, the diverse methods explored—string concatenation, string formatting, pickle
, NumPy
, JSON
, and csv
—provide an array of options for saving variables to files. String operations offer simplicity, while libraries like Pickle enable serialization for complex structures.
Additionally, NumPy excels in handling numerical data, JSON provides a human-readable format, and CSV is optimal for tabular data. Armed with these techniques, you can tailor your approach to match the nature of your variables, ensuring efficient and effective data storage in Python.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn