How to Remove Newline From String in Python
-
Use the
str.strip()
Method to Remove a Newline Character From the String in Python -
Use the
replace()
Function to Remove Newline Characters From a String in Python -
Use the
re.sub()
Method to Remove a Newline Character From the String in Python -
Use the
str.translate()
Method to Remove a Newline Character From the String in Python -
Use
str.join()
andsplit()
to Remove a Newline Character From the String in Python - Conclusion
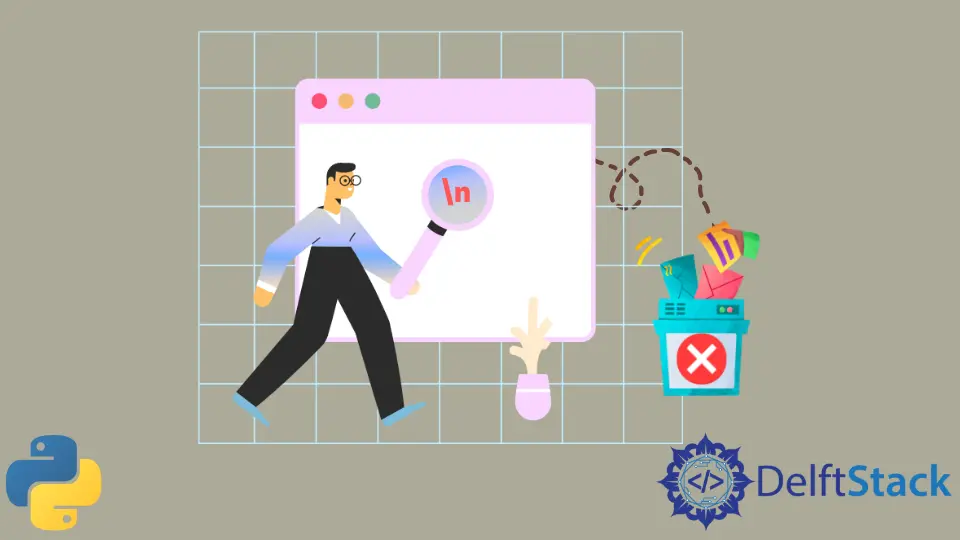
When dealing with text data in Python, it’s common to encounter newline characters (\n
) that might interfere with various string operations.
In this article, we’ll explore different methods to effectively remove newline characters from strings, providing a range of options suitable for various scenarios.
Use the str.strip()
Method to Remove a Newline Character From the String in Python
One straightforward method to remove newline characters (\n
) is by utilizing the str.strip()
method. By default, this method is designed to remove leading and trailing whitespaces from a string, but you can specify additional characters to remove by passing them as an argument.
In our case, we’ll pass \n
to eliminate newline characters specifically.
Its syntax is as follows:
new_string = original_string.strip("\n")
Here, original_string
is the string from which you want to remove leading and trailing whitespaces. By calling strip('\n')
, we instruct Python to remove any leading and trailing newline characters. The result is stored in new_string
.
Consider a scenario where we have a string with newline characters, and we want to remove them using str.strip()
:
original_string = "Hello\nWorld"
new_string = original_string.strip()
print("Original String:")
print(original_string)
print("\nNew String after Removing Newlines:")
print(new_string)
In this example, we start with the original string containing the newline character between Hello
and World
. The str.strip()
method is then applied to original_string
.
As a result, the leading and trailing newline characters are removed, and the modified string is stored in the variable new_string
.
Output:
Original String:
Hello
World
New String after Removing Newlines:
Hello
World
While str.strip()
is excellent for removing both leading and trailing whitespaces, including newline characters, there might be situations where you specifically want to remove only the trailing newline characters.
For this purpose, Python provides the str.rstrip()
method. This method has a syntax that is similar to str.strip()
Let’s enhance our previous example by using str.rstrip()
to remove only the trailing newline character:
original_string = "Hello\nWorld\n"
new_string = original_string.rstrip()
print("Original String:")
print(original_string)
print("\nNew String after Removing Trailing Newlines:")
print(new_string)
In this updated example, the original string contains a newline character at the end. We then apply str.rstrip()
to original_string
.
As a result, only the trailing newline character is removed, leaving the newline character between Hello
and World
intact.
Original String:
Hello
World
New String after Removing Trailing Newlines:
Hello
World
This distinction between str.strip()
and str.rstrip()
provides flexibility based on your specific requirements.
Use the replace()
Function to Remove Newline Characters From a String in Python
While str.strip()
and str.rstrip()
offer specific ways to handle newline characters, another versatile method for string manipulation is str.replace()
. This method allows us to replace occurrences of a specified substring within a string, making it suitable for removing newline characters as well.
The str.replace()
method takes two parameters: the substring to be replaced and the substring to replace it with. To remove newline characters, we replace them with an empty string.
The syntax is as follows:
new_string = original_string.replace("\n", "")
Here, original_string
is the input string, and "\n"
is the newline character we want to remove. The resulting string is stored in new_string
.
Let’s explore this approach with another example:
original_string = "Python\nProgramming\nIs\nFun"
new_string = original_string.replace("\n", "")
print("Original String:")
print(original_string)
print("\nNew String after Removing Newlines:")
print(new_string)
Here, original_string
is the input string, r"\n"
is the newline character pattern, and the resulting string is stored in new_string
.
Let’s delve into an example:
import re
original_string = "Data\nScience\nwith\nPython"
new_string = re.sub(r"\n", "", original_string)
print("Original String:")
print(original_string)
print("\nNew String after Removing Newlines:")
print(new_string)
In this example, the original string contains newline characters separating each term. By utilizing re.sub()
, we define the pattern r"\n"
to match newline characters.
The method then replaces each occurrence of this pattern with an empty string, effectively removing all newline characters from the input string.
Output:
Original String:
Data
Science
with
Python
New String after Removing Newlines:
DataSciencewithPython
Use the str.translate()
Method to Remove a Newline Character From the String in Python
For more advanced string manipulations, the str.translate()
method in Python offers a versatile solution. This method, when coupled with the str.maketrans()
function, allows us to remove newline characters and other specified characters from a string.
The str.translate()
method requires a translation table as an argument, which can be generated using the str.maketrans()
function. We specify the characters to be replaced and their corresponding replacements.
In the case of removing newline characters, the newline character ("\n"
) is mapped to None
.
The syntax is as follows:
translation_table = str.maketrans("", "", "\n")
new_string = original_string.translate(translation_table)
Here, original_string
is the input string, and "\n"
is the newline character to be removed. The resulting string is stored in new_string
.
Let’s dive into a comprehensive example:
original_string = "Artificial\nIntelligence\nin\nPython"
translation_table = str.maketrans("", "", "\n")
new_string = original_string.translate(translation_table)
print("Original String:")
print(original_string)
print("\nNew String after Removing Newlines:")
print(new_string)
In this example, we start with an original string containing newline characters between each term. Then, the translation table is created using str.maketrans("", "", "\n")
, specifying that newline characters should be replaced with None
.
The str.translate()
method is then applied to original_string
, utilizing the translation table to remove newline characters.
Output:
Original String:
Artificial
Intelligence
in
Python
New String after Removing Newlines:
ArtificialIntelligenceinPython
Use str.join()
and split()
to Remove a Newline Character From the String in Python
Python’s string manipulation capabilities extend further with the combination of the str.join()
and str.split()
methods.
The str.join()
method concatenates elements of an iterable, such as a list, into one string. On the other hand, str.split()
divides a string into a list of substrings based on a specified delimiter.
By splitting on newline characters ("\n"
), we can effectively remove them.
The syntax is as follows:
lines = original_string.split("\n")
new_string = "".join(lines)
Here, original_string
is the input string, "\n"
is the delimiter for splitting, and the resulting string is stored in new_string
.
Essentially, this approach allows us to split a string into a list of substrings based on a specified delimiter, remove newline characters, and then join the list back into a single string using the str.join()
method.
Let’s explore this approach with a different example:
original_string = "Machine\nLearning\nwith\nPython"
lines = original_string.split("\n")
new_string = "".join(lines)
print("Original String:")
print(original_string)
print("\nNew String after Removing Newlines:")
print(new_string)