在 Python 中从字符串中删除换行符
-
在 Python 中使用
strip()
函数从字符串中删除换行符 -
在 Python 中使用
replace()
函数从字符串中删除换行符 -
在 Python 中使用
re.sub()
函数从字符串中删除换行符
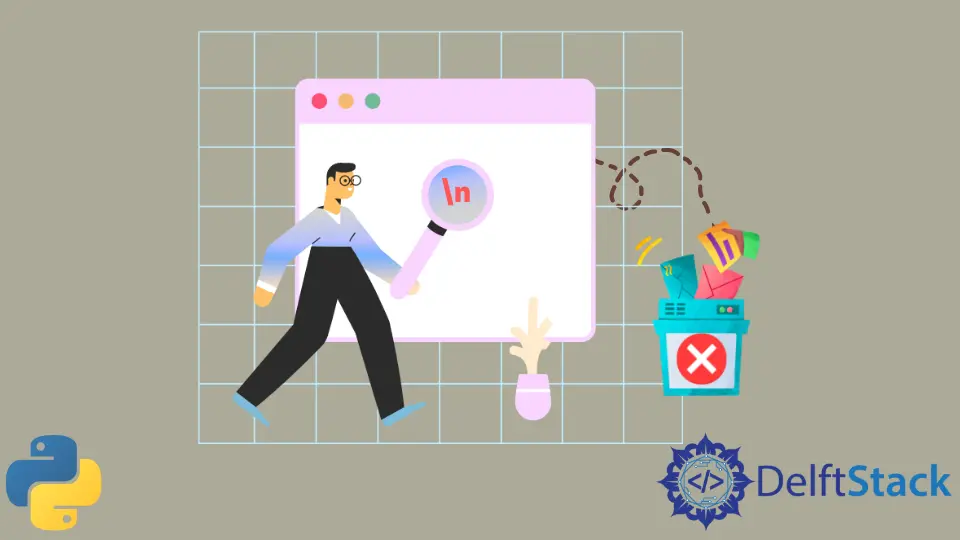
Python 中的字符串可以定义为用单引号或双引号括起来的 Unicode 字符簇。
与其他流行的编程语言一样,Python 也有一个由 \n
表示的换行符。它主要用于跟踪一行的顶点和字符串中新行的出现。
换行符也可以在 f 字符串中使用。此外,根据 Python 文档,print 语句默认在字符串末尾添加换行符。
本教程将讨论在 Python 中从字符串中删除换行符的不同方法。
在 Python 中使用 strip()
函数从字符串中删除换行符
strip()
函数用于从正在操作的字符串中删除尾随和前导换行符。它还删除字符串两侧的空格。
以下代码使用 strip()
函数从 Python 中的字符串中删除换行符。
str1 = "\n Starbucks has the best coffee \n"
newstr = str1.strip()
print(newstr)
输出:
Starbucks has the best coffee
如果只需要删除尾随的换行符,可以使用 rstrip()
函数代替 strip 函数。前导换行符不受此函数影响并保持原样。
以下代码使用 rstrip()
函数从 Python 中的字符串中删除换行符。
str1 = "\n Starbucks has the best coffee \n"
newstr = str1.rstrip()
print(newstr)
输出:
Starbucks has the best coffee
在 Python 中使用 replace()
函数从字符串中删除换行符
也称为蛮力方法,它使用 for
循环和 replace()
函数。我们在字符串中寻找换行符\n
作为字符串,并在 for
循环的帮助下从每个字符串中手动替换它。
我们使用字符串列表并在其上实现此方法。列表是 Python 中提供的四种内置数据类型之一,可用于在单个变量中存储多个项目。
以下代码使用 replace()
函数从 Python 中的字符串中删除换行符。
list1 = ["Starbucks\n", "has the \nbest", "coffee\n\n "]
rez = []
for x in list1:
rez.append(x.replace("\n", ""))
print("New list : " + str(rez))
输出:
New list : ['Starbucks', 'has the best', 'coffee ']
在 Python 中使用 re.sub()
函数从字符串中删除换行符
re
模块需要导入到 python 代码中才能使用 re.sub()
函数
re
模块是 Python 中的内置模块,用于处理正则表达式。它有助于执行在给定的特定字符串中搜索模式的任务。
re.sub()
函数本质上用于获取子字符串并将其在字符串中的出现替换为另一个子字符串。
以下代码使用 re.sub()
函数从 Python 中的字符串中删除换行符。
# import the regex library
import re
list1 = ["Starbucks\n", "has the \nbest", "coffee\n\n "]
rez = []
for sub in list1:
rez.append(sub.replace("\n", ""))
print("New List : " + str(rez))
输出:
New List : ['Starbucks', 'has the best', 'coffee ']
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn