How to Read a File Without Newlines in Python
-
Use the
strip()
and therstrip()
Methods to Read a Line Without a Newline in Python -
Use the
splitlines()
and thesplit()
Methods to Read a Line Without a Newline in Python -
Use
slicing
or the[]
Operator to Read a Line Without a Newline in Python -
Use the
replace()
Method to Read a Line Without a Newline in Python - Conclusion
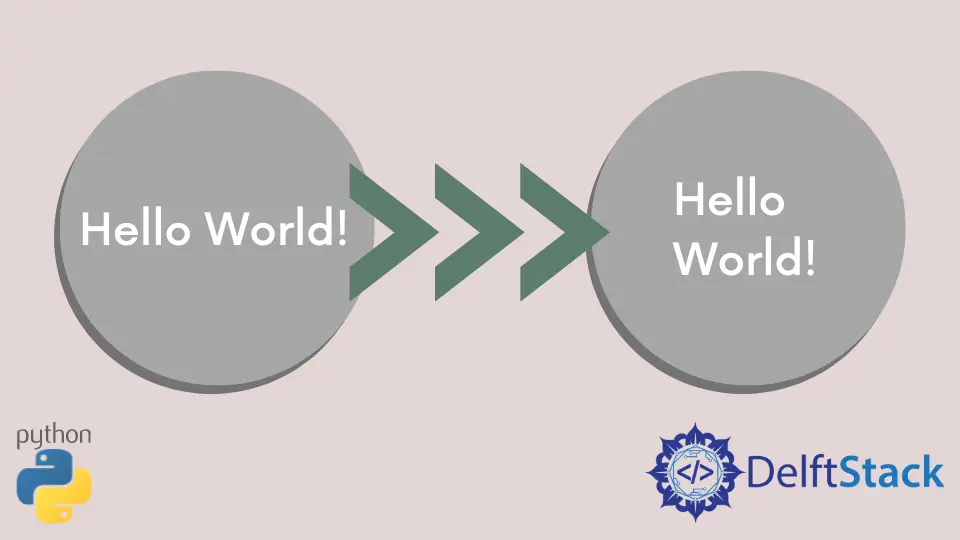
File handling (such as editing a file, opening a file, and reading a file) can easily be carried out in Python. Reading a file in Python is a very common task that any user performs before making any changes to the file.
While reading the file, the newline character \n
is used to denote the end of a file and the beginning of the next line. This tutorial will demonstrate how to read a line without a newline character in Python.
Use the strip()
and the rstrip()
Methods to Read a Line Without a Newline in Python
Python provides convenient methods like strip()
and rstrip()
to handle strings and effectively remove unwanted characters, including newlines.
The strip()
method removes characters (default is whitespace) from the beginning and end of a string. It takes an optional argument that specifies which characters to remove from the string.
Syntax:
string.strip([chars])
Parameters:
string
: The original string from which characters will be removed.chars
(optional): A string specifying the characters to remove. If not provided, the method will remove whitespace characters by default.
In the example below, we demonstrate how to read a file line by line and remove both spaces and newlines using strip()
:
with open("randomfile.txt", "r") as file:
newline_break = ""
for readline in file:
line_strip = readline.strip()
newline_break += line_strip
print(newline_break)
Here, we open the file using open()
and iterate through its lines. For each line, we use strip()
to remove any leading and trailing spaces, including the newline character (\n
).
Thus, we successfully read a line without the trailing newline.
However, if you want to preserve leading spaces and only remove the newline character, you can pass the newline character ('\n'
) as an argument to strip()
. This will ensure only newlines are removed while preserving other spaces:
with open("randomfile.txt", "r") as file:
newline_break = ""
for readline in file:
line_strip = line.strip("\n")
newline_break += line_strip
print(newline_break)
Alternatively, you can use the rstrip()
method, which specifically removes characters from the end (right) of a string. Since newlines typically appear at the end of strings, rstrip('\n')
will effectively remove the newline character:
Syntax:
string.rstrip([chars])
Parameters:
string
: The original string from which characters will be removed.chars
(optional): A string specifying the characters to remove from the end. If not provided, it will remove whitespace characters by default.
Take a look at the example below:
with open("randomfile.txt", "r") as file:
newline_break = ""
for readline in file:
line_strip = line.rstrip("\n")
newline_break += line_strip
print(newline_break)
Both strip()
and rstrip()
methods provide flexible ways to handle newlines in Python and tailor the output according to your requirements. Experiment with these methods to achieve the desired text processing results.
Use the splitlines()
and the split()
Methods to Read a Line Without a Newline in Python
The two methods, splitlines()
and split()
, also offer distinct ways to read lines without unwanted newline characters while working with the file content.
The splitlines()
method is particularly designed for splitting a string into a list of strings, where each element of the list corresponds to a line in the original string. This means that the splitlines()
method automatically detects newline characters and uses them as delimiters for the splitting operation.
Let’s delve into this with an illustrative code example:
with open("randomfile.txt", "r") as file:
readline = file.read().splitlines()
print(readline)
In this code block, we open the randomfile.txt
file in read mode and use the read()
method to obtain its contents as a single string. Then, we apply splitlines()
to this string.
The result is a list, readline
, where each element represents a line from the file content. The splitlines()
method automatically handles the newline characters, ensuring that each line is properly separated.
However, there may be scenarios where you need more precise control over where the string should be split rather than relying solely on newline characters. This is where the split()
method comes in handy.
While splitlines()
uses newline characters as its default delimiter, split()
allows you to specify any character(s) as the delimiter.
Consider the following code snippet:
with open("randomfile.txt", "r") as file:
readline = file.read().split("\n")
print(readline)
Here, we open the same randomfile.txt
file and read its contents as a single string. However, instead of relying on the automatic newline detection of splitlines()
, we explicitly specify \n
(newline character) as the delimiter for the split()
method.
This way, we gain finer control over where the string should be split, ensuring that the newline characters are excluded from the resulting list.
Use slicing
or the []
Operator to Read a Line Without a Newline in Python
The slicing
operator is a powerful tool for extracting specific segments of a sequence or a string. It allows us to precisely access different parts of the sequence or string by specifying a starting index, an ending index, and an optional step value within square brackets, encapsulating the desired range.
The syntax for slicing
is defined as follows:
string[starting index: ending index: step value]
In this structure, the starting index
denotes the position where the slicing begins, the ending index
signifies the position where the slicing concludes (exclusive), and the step value
dictates the interval between elements being selected.
Let’s delve into a practical example illustrating how this can be employed to read lines without including the trailing newline character:
with open("randomfile.txt", "r") as file:
newline_break = ""
for line in file:
line_strip = line[:-1] # Remove the last character (newline)
newline_break += line_strip
print(newline_break)
In this code block, we open the file randomfile.txt
in read mode and iterate through its lines. For each line, we utilize negative slicing with line[:-1]
to discard the last character, which is typically the newline character (\n
).
By excluding this character, we effectively read each line without including the trailing newline. The newline_break
variable accumulates these modified lines, and the result is printed, showing the lines devoid of newline characters.
Use the replace()
Method to Read a Line Without a Newline in Python
The replace()
method in Python is a versatile tool that allows for the replacement of substrings within a string with another specified substring. This functionality proves to be incredibly useful when it comes to modifying text and, in our case, reading lines from a file without including the trailing newline character.
Here’s a breakdown of how the replace()
method works:
with open("randomfile.txt", "r") as file:
newline_break = ""
for line in file:
line_strip = line.replace("\n", " ") # Replace newline with a space
newline_break += line_strip
print(newline_break)
For each line, the replace()
method is employed to substitute any newline character (\n
) with a space (" "
). This effectively removes the newline character while keeping the rest of the line intact.
The modified line is then concatenated to the newline_break
variable.
The replace()
method operates by scanning the input string (line
in this case) and replacing all occurrences of the specified substring (\n
) with the provided replacement string (a space in this scenario). It ensures that every newline character within the line is substituted, consequently allowing us to read each line without including the newline.
Conclusion
Python offers various methods to read lines from a file without including the trailing newline character. Techniques such as strip()
and rstrip()
to remove unwanted characters, splitlines()
and split()
for controlled splitting, slicing
or the []
operator for precise extraction, and the replace()
method for substring substitution provide flexible options to tailor the output according to specific requirements.
By understanding and implementing these methods, we can effectively handle file content and streamline text processing. Experimentation with these approaches enables efficient manipulation of file data while excluding unwanted newline characters.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn