How to Randomly Select Item From a List in Python
-
Use Module
random
to Select a Random Item From a List in Python -
Use Module
secrets
to Select a Random Item From a List in Python -
Use Module
NumPy
to Select a Random Item From a List in Python
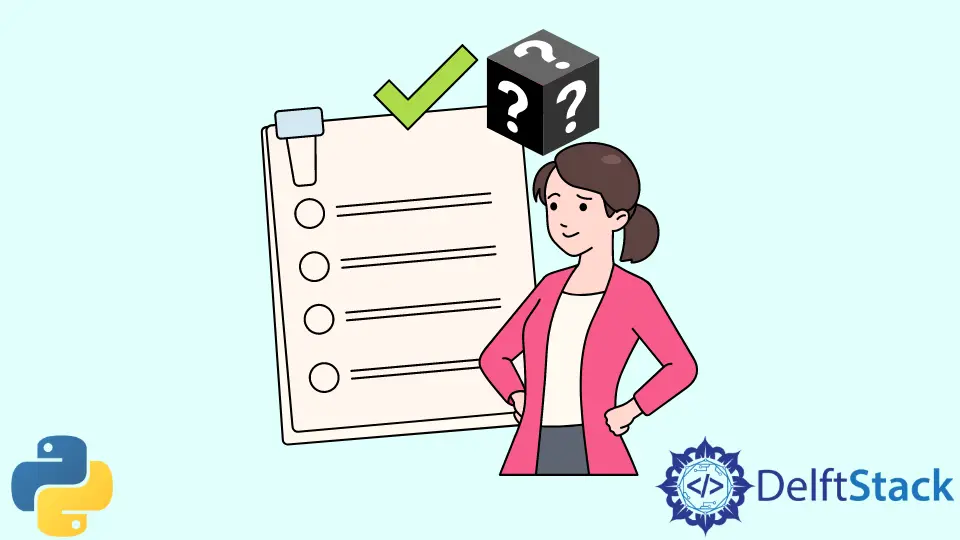
This tutorial shows you how to select a random item from a list in Python. There are multiple simple ways to achieve this, all of them involving the import of Python modules.
This tutorial will cover solutions that require the modules random
, secrets
, and NumPy
.
Take note that all of these solutions that will be presented will make use of pseudo random number generators (PRNG).
Use Module random
to Select a Random Item From a List in Python
The most common module used for randomizing is the module random
. This module implements pseudo-random utility functions to support operations involving randomization.
Let’s say we want to pick a random name out of a list as if it were a ballot.
["John", "Juan", "Jane", "Jack", "Jill", "Jean"]
To pick out a random name from this list, we’ll use random.choice()
, which will select an item from the available data that is given.
import random
names = ["John", "Juan", "Jane", "Jack", "Jill", "Jean"]
def selectRandom(names):
return random.choice(names)
print("The name selected is: ", selectRandom(names))
The output, of course, will be variable and random. So it can be any of the six names that are stored within variable names
.
Use Module secrets
to Select a Random Item From a List in Python
The secrets
module essentially is used for the same purpose as random
. However, secrets
provides a cryptographically secure method of implementing PRNG.
In real life applications like storing passwords, authentication, encryption and decryption, and tokens. secrets
is much safer than using random
, as it is good only for simulation or operations that do not handle sensitive data.
In this problem, both modules provide the same value, as we aren’t handling any sensitive data and are doing this for simulation.
We will use the same list of names
for this example. secrets
also has a version of the function choice()
which produces the same variable output as random.choice()
.
import secrets
names = ["John", "Juan", "Jane", "Jack", "Jill", "Jean"]
def selectRandom(names):
return secrets.choice(names)
print("The name selected is: ", selectRandom(names))
Use Module NumPy
to Select a Random Item From a List in Python
The NumPy
module also has utility functions for randomizing and has a few expansive tools as arguments for its choice()
function.
Again, we’ll use the same list names
to demonstrate the function numpy.random.choice()
.
import numpy
names = ["John", "Juan", "Jane", "Jack", "Jill", "Jean"]
def selectRandom(names):
return numpy.random.choice(names)
print("The name selected is: ", selectRandom(names))
The function will return the same variable output that the two other modules produced.
NumPy
also provides other arguments for choice()
to generate multiple outputs in the form of a list.
The second argument accepts an integer value to determine how many random items to return. Let’s say we want to return 4 random items from the list names
.
def selectRandom(names):
return numpy.random.choice(names, 4)
print("The names selected are: ", selectRandom(names))
Sample output:
The names selected are: ['John', 'Jill', 'Jill', 'Jill']
There is a likelihood that the same item will be repeated more than once in the randomized result.
If you prefer the result items to be unique, we can pass a third boolean argument, enabling random sampling without replacement.
def selectRandom(names):
return numpy.random.choice(names, 4, False)
Sample output:
The names selected are: ['Jill', 'John', 'Jack', 'Jean']
The function will always produce a unique list without any duplicate items.
One major drawback if we add the third argument is the function’s runtime since it executes an added task to check for duplicates and replace them with an item that doesn’t exist within the results yet.
In summary, selecting random items from a Python list can be achieved by using one of these three modules: random
, secrets
, or NumPy
. Each one has its benefits and drawbacks.
If you want to have a cryptographically secure PRNG method, then secrets
is the best module for that. If your purpose is only for simulation or non-sensitive data manipulation, use either random
or NumPy
. If you want more than a single random result, then use NumPy
.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python