How to Print Without Newline in Python
-
Print Without the Newline in Python Using the
end
Parameter in theprint()
Function -
Print Without the Newline in Python Using the
sys.stdout.write()
Function
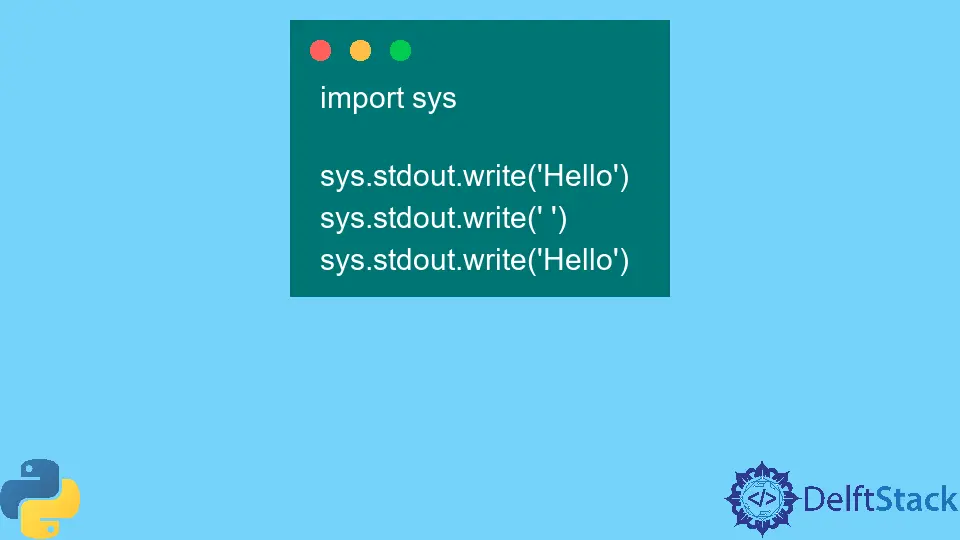
This tutorial will demonstrate various methods to print the text without a newline in Python. The print()
function in Python 2 and 3, adds the newline at the end of the input text each time it is called. This tutorial will explain how we can print the text in the same line with and without a space in Python 2 and 3.
Print Without the Newline in Python Using the end
Parameter in the print()
Function
The print(object(s), sep, end)
function in Python 3, takes one or more object
as input, converts it into the string, and then prints it. The sep
parameter (default value ' '
) represents the separator used by the print()
function to separate the input objects
if multiple objects are provided. The end
parameter (default value \n
) represents the value the print()
function prints at the end of the last object
.
To print the text without the newline, we can pass an empty string as the end
argument to the print()
function. Similarly, we can pass the empty string as the sep
argument if we do not want a space between each object
.
The example code below demonstrates how to print without a newline using the print()
function in Python 3:
print("Hello", end="", sep="")
print(" ", end="", sep="")
print("Hello", end="", sep="")
Output:
Hello Hello
print_function
from the future
module to use the above code.Example code:
from __future__ import print_function
print("Hello", end="", sep="")
print(" ", end="", sep="")
print("Hello", end="", sep="")
Output:
Hello Hello
Print Without the Newline in Python Using the sys.stdout.write()
Function
The sys.stdout.write()
function prints the text provided as input on the screen. It does put the newline \n
at the end of the text.
Therefore, to print the text without the newline in Python, we can pass the text to the sys.stdout.write()
function as shown in the below example code:
import sys
sys.stdout.write("Hello")
sys.stdout.write(" ")
sys.stdout.write("Hello")
Output:
Hello Hello
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn