How to Print Lists in Python
-
Use the
map()
Function to Print Lists in Python -
Use the
*
Operator to Print Lists in Python -
Use a
for
Loop to Print Lists in Python -
Use the
join()
Method to Print Lists in Python
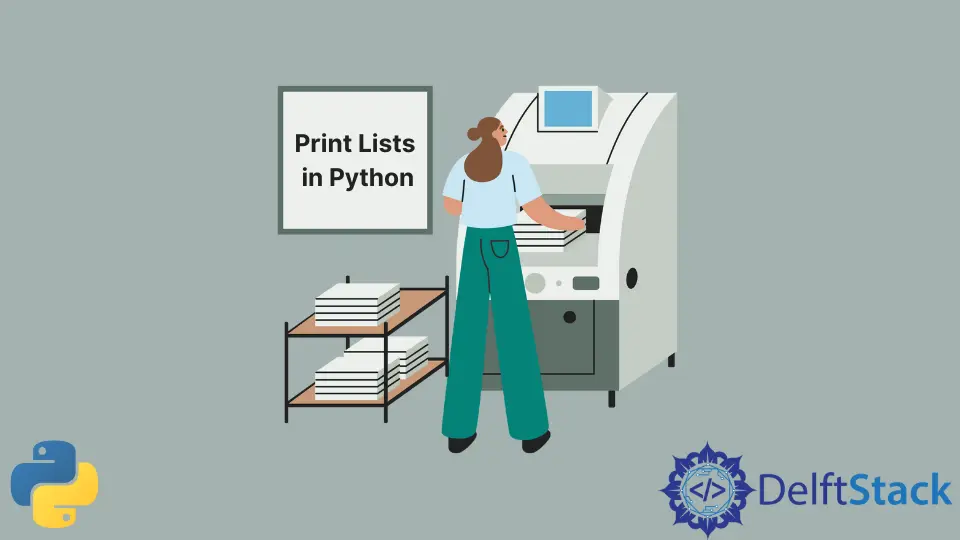
In Python, four types of built-in data types are used to store multiple elements as a collection. These are lists, tuples, sets, and dictionaries. Here, lists are used very often by any user. As lists already store data in sequence, there are different ways of printing them to make them look more presentable and easy to read.
This tutorial will demonstrate different ways of printing a list in Python.
Use the map()
Function to Print Lists in Python
The map()
function is a built-in feature in Python. This command, also known as mapping, is used to manipulate all the elements in an iteration or a sequence without using any kind of loop. This function basically converts one type of iterable into another type. See the example below.
list = [5, 10, 15, 20, 25]
print(list)
print("After using the mapping technique: ")
print("\n".join(map(str, list)))
Output:
[5, 10, 15, 20, 25]
After using the mapping technique:
5
10
15
20
25
In the program above, note that the join()
method was implemented. The join()
function in Python is used to join elements of any iterable with the help of a string separator. The string separator used above is \n,
, which is the new line character used to denote the end of a line. It’s why every element is in a different line in the output.
Use the *
Operator to Print Lists in Python
The *
operator is the most commonly used operator of the many operators present in Python. Except for performing multiplication, the *
operator is used to print each element of a list in one line with a space between each element.
Along with the *
operator, the new line character \n
can also be used with the help of the sep =
parameter in the print statement itself. The sep =
parameter basically provides a separator between the strings. Check out the sample code below.
list = [5, 10, 15, "Twenty", 25]
print(list)
print("After using the * operator: ")
print(*list)
Output:
[5, 10, 15, 'Twenty', 25]
After using the * operator:
5 10 15 Twenty 25
The new line character \n
can be used with the help of sep =
in the last print statement after placing a comma after *list
.
Use a for
Loop to Print Lists in Python
The for
loop is commonly used in any programming language. It is used to iterate over a sequence like a tuple, a dictionary, a list, a set, or a string and execute for each and every element present in the sequence.
Example:
list = [5, 10, 15, "Twenty", 25]
print("After using for loop:")
for l in list:
print(l)
Output:
[5, 10, 15, 'Twenty', 25]
After using for loop:
5
10
15
Twenty
25
Here, the for
loop executes over each and every element present in the given list.
Use the join()
Method to Print Lists in Python
The join()
function in Python is used to join elements of any iterable like a list, a tuple, or a string with the help of a string separator; this method returns a concatenated string as an output. Look at the example below.
list = ["Five", "Ten", "Fifteen", "Twenty"]
print(" ".join(list))
Output:
Five Ten Fifteen Twenty
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python