How to Make a Pascal's Triangle in Python
- Pascal’s Triangle Algorithm in Python
- The Program for Pascal’s Triangle in Python
- Print Pascal’s Triangle Using the Binomial Coefficient in Python
-
Print Pascal’s Triangle by Computing the Power of
11
in Python
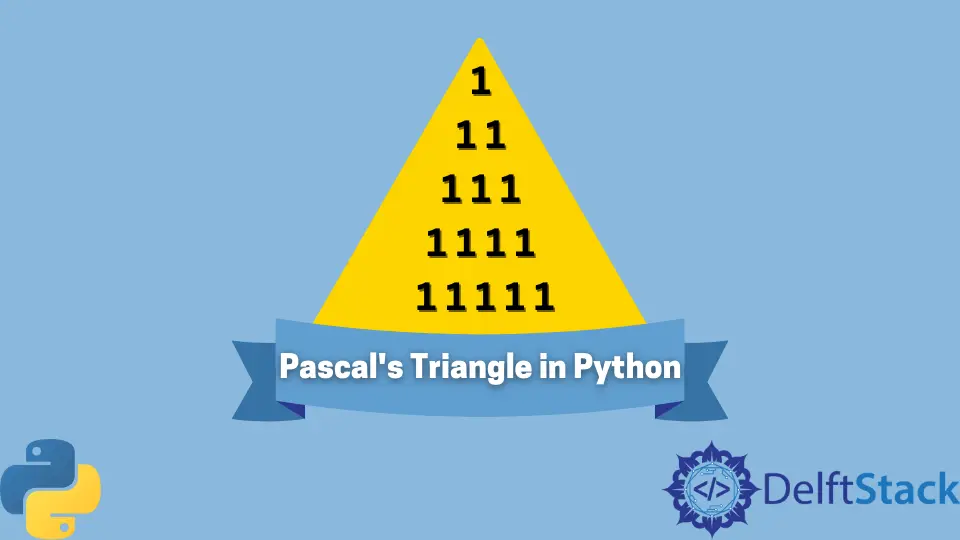
Pascal’s triangle is defined as a type of number pattern in which numbers are arranged so that they look like a triangle. A triangular array is formed in this Mathematics concept, formed by numbers that are the sum of the adjacent row. Additionally, the external edges are always 1.
Pascal’s Triangle Algorithm in Python
To form a pascal triangle in Python, there is a stepwise in the software.
- Firstly, an input number is taken from the user to define the number of rows.
- Secondly, an empty list is defined, which is used to store values.
- Then, a
for
loop is used to iterate from0
ton-1
that append the sub-lists to the initial list. - After that,
1
is appended to the list. - Then, a
for
loop is used again to put the values of the number inside the adjacent row of the triangle. - Finally, the Pascal Triangle is printed according to the given format.
The Program for Pascal’s Triangle in Python
input_num = int(input("Enter the number of rows: "))
list = [] # an empty list
for n in range(input_num):
list.append([])
list[n].append(1)
for m in range(1, n):
list[n].append(list[n - 1][m - 1] + list[n - 1][m])
if input_num != 0:
list[n].append(1)
for n in range(input_num):
print(" " * (input_num - n), end=" ", sep=" ")
for m in range(0, n + 1):
print("{0:5}".format(list[n][m]), end=" ", sep=" ")
print()
Output:
Enter the number: 5
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Print Pascal’s Triangle Using the Binomial Coefficient in Python
In this method, every line in the triangle consists of just 1
, and the nth
number in a row is equal to the Binomial Coefficient. Look at the example program below.
num = int(input("Enter the number of rows:"))
for n in range(1, num + 1):
for m in range(0, num - n + 1):
print(" ", end="")
# first element is always 1
B = 1
for m in range(1, n + 1):
# first value in a line is always 1
print(" ", B, sep="", end="")
# using Binomial Coefficient
BC = B * (n - m) // m
print()
Output:
Enter the number of rows:5
1
1 1
1 1 1
1 1 1 1
1 1 1 1 1
In this method, the formula used for the Binomial Coefficient is:
BC = B(line(m), n-1) * (line(m) - n + 1) / n
Print Pascal’s Triangle by Computing the Power of 11
in Python
This method is completely based on the power of the number 11
as the increasing values of the power on the number 11
form the Pascal Triangle pattern.
Mathematically, here’s how it goes.
11 * 0 = 1
11 * 1 = 11
11 * 2 = 121
11 * 3 = 1331
11 * 4 = 14641
Now applying this technique in Python, refer to the code block below.
num = int(input("Enter the number of rows:"))
for n in range(num):
print(" " * (num - n), end="")
print(" ".join(map(str, str(11 ** n))))
Output:
Enter the number of rows:5
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn