How to Multiply List by Scalar in Python
- Multiply List Elements by a Scalar Using List Comprehensions in Python
-
Multiply List Elements by a Scalar Using the
map()
Function in Python - Multiply List Elements by a Scalar Using Lambda Functions in Python
-
Multiply List Elements by a Scalar Using
NumPy
Arrays in Python - Conclusion
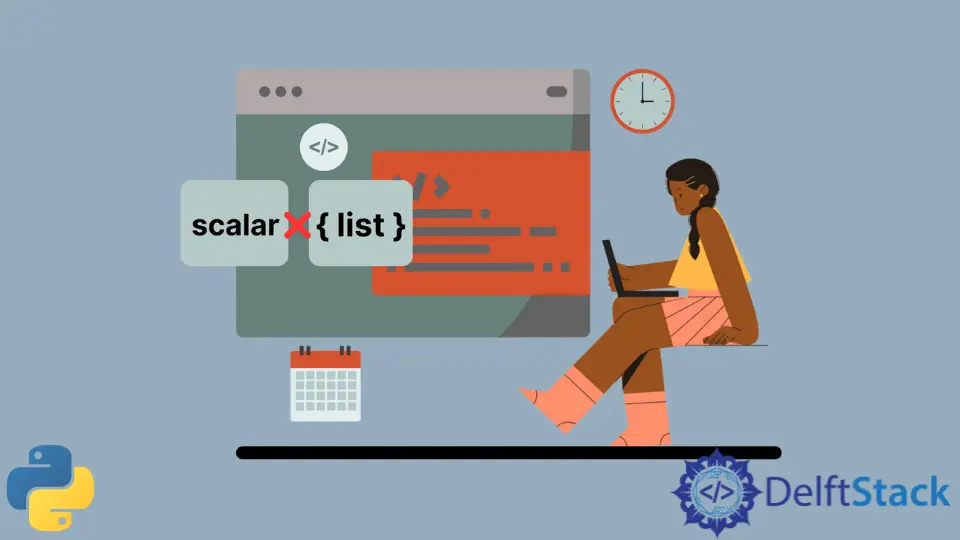
In Python, a common and versatile task is multiplying all elements of a list by a scalar. This operation finds applications in data scaling, mathematical calculations, and data transformations.
This tutorial will discuss the ways to multiply all the list elements with a scalar multiple in Python.
Multiply List Elements by a Scalar Using List Comprehensions in Python
List comprehensions are a way to perform operations on each list element without using a for
loop in Python. List comprehensions are superior to loops because they have faster execution time and less code.
Syntax:
result_list = [expression for element in original_list]
Parameters:
result_list
: The new list that will store the results of the operation.expression
: The mathematical expression or operation you want to apply to each element of theoriginal_list
.element
: This variable represents each element in theoriginal_list
.original_list
: The list you want to transform by multiplying its elements with a scalar.
In the syntax, we created a new list called result_list
. Then, for each element
in the original_list
, apply the expression
to that element
.
Lastly, we store the result of the operation in result_list
.
The following code snippet shows how to multiply all the list elements with a scalar quantity with list comprehensions in Python.
li = [1,2,3,4]
multiple = 2.5
li = [x*multiple for x in li]
print(li)
In the code above, we have a list li
, which contains the numbers [1, 2, 3, 4]
. We also have a variable multiple
, which is set to 2.5
, and this variable represents the number we want to multiply each element in our list by.
Now, we apply a list comprehension, an efficient way of doing a loop. In this comprehension, we go through each element x
in our list li
.
For each element x
, we multiply it by multiple
, which is 2.5
. In simpler terms, for each number in our list, we multiply it by 2.5
.
The new list is stored back in the variable li
. So, li
now holds our modified list with the results of the multiplication.
Output:
[2.5, 5.0, 7.5, 10.0]
We see that the modified list li
contains: [2.5, 5.0, 7.5, 10.0]
. Each number in this new list is the result of our multiplication operation.
Multiply List Elements by a Scalar Using the map()
Function in Python
The map()
function is used to apply a user-defined function on each element of a particular iterable. The map()
function takes the name of the method and iterable as input parameters and applies the method to each element of the iterable.
The map()
function returns a map object for each element and is converted into a list with the list()
function in Python.
Syntax:
result_list = list(map(function, original_list))
Parameters:
result_list
: The new list that will store the results of the operation.function
: A custom function that defines the operation to be performed on each element from theoriginal_list
.original_list
: The list you want to process and transform.
In the syntax above, we use the map()
function to apply our custom function
to each element in original_list
. Then, we convert it to a list, and the list()
function turns the results into a list, which we store in result_list
.
The following code example shows how we can use the map()
function with a user-defined method to multiply all list elements with a scalar in Python.
li = [1,2,3,4]
multiple = 2.5
def multiply(le):
return le*multiple
li = list(map(multiply,li))
print(li)
In the code above, we start with a list, li
, containing values [1, 2, 3, 4]
. Then, we define a variable named multiple
with the value 2.5
for multiplying elements in the list.
Next, we create a custom function, multiply
, to perform the multiplication operation. Using map()
, we apply multiply
to each element in li
, effectively multiplying by multiple
.
The line li = list(map(multiply, li))
updates li
with the modified values. Finally, print(li)
displays the modified list.
Output:
[2.5, 5.0, 7.5, 10.0]
In the output, we use our custom multiply
function to calculate the results.
We repeat this for all elements, creating a new list with each element multiplied by 2.5
. Then, we use print(li)
to show the modified list.
Multiply List Elements by a Scalar Using Lambda Functions in Python
Lambda functions in Python are anonymous and one-liner functions that we only need to use once in our whole code. By anonymous, we mean that these functions have no name.
We can specify these functions in Python with the lambda keyword. We can use these lambda functions inside the previously discussed map()
function to apply them to each list element.
Syntax:
result_list = list(map(lambda x: x * scalar, original_list))
Parameters:
result_list
: The new list that will store the results of the operation.x
: The variable representing each element in theoriginal_list
.scalar
: The scalar value that you want to use for multiplication.original_list
: The list you want to process and transform.
In the syntax above, we use a lambda function to multiply each element, represented by x
, in the original_list
by a scalar value scalar
. Then, the results are collected into a new list, result_list
, using map()
.
This allows us to quickly multiply every element in original_list
and store the results in result_list
.
The following code example shows how we can use lambda functions inside the map()
function to multiply each list element by a scalar in Python.
li = [1,2,3,4]
multiple = 2.5
li = list(map(lambda x: x*multiple, li))
print(li)
In the code above, we start with a list, li
, containing numbers [1, 2, 3, 4]
. Then, we set multiple
to 2.5
, the number we want to use for multiplication.
Next, we apply a lambda function. It multiplies each element x
in li
by multiple
, essentially multiplying every number in the list by 2.5
.
We update li
with the results. So, now li
holds the modified list with the results of the multiplications.
Lastly, we use print(li)
to display the content of our modified list.
Output:
[2.5, 5.0, 7.5, 10.0]
The output above reveals our new list, [2.5, 5.0, 7.5, 10.0]
. Each number in this list is the result of our multiplication operation.
This method is much superior if compared to the previous method because we probably don’t need to use this function anywhere else in our code. Lambda functions are preferable over conventional ones when we need to use them only once in our code.
Multiply List Elements by a Scalar Using NumPy
Arrays in Python
All the methods discussed previously perform operations on lists in Python. The previous methods work great when the list is small.
The only drawback of using the previous methods is that list operations are generally slower than NumPy
arrays.
Multiplying list elements by a scalar using NumPy
arrays in Python involves performing element-wise multiplication on a Python list that has been converted into a NumPy
array. This operation allows you to multiply each element of the list by the same scalar value efficiently.
In this method, we first convert our list into a NumPy
array, multiply that array with a scalar, and then convert the resultant array back to a list with the tolist()
function of NumPy
arrays.
Syntax:
arr = np.array(original_list) * scalar
Parameters:
original_list
: This is the list you want to convert into aNumPy
array and then multiply by the scalar.scalar
: It represents the value by which you want to multiply each element in theNumPy
array.
In the syntax above, we initialize arr
, where we store the result of our operation. Then, the np.array(original_list)
converts our original list into a NumPy
array.
Next, we multiply each NumPy
array element by the scalar value on the right.
This method is preferable when the list size is huge and requires a lot of computation. The following code example demonstrates the working of this method in Python.
import numpy as np
li = [1,2,3,4]
multiple = 2.5
arr = np.array(li)
arr = arr * multiple
li = arr.tolist()
print(li)
In the code above, we converted the list li
into the array arr
with the np.array(li)
function. We then multiplied the array with the scalar multiple
.
Unlike lists, we can easily multiply each element of a NumPy
array with a scalar using arr = arr * multiple
. After that, we converted our new array back into a list with the arr.tolist()
function.
Output:
[2.5, 5.0, 7.5, 10.0]
In the end, the results after multiplying each element of the list li
with 2.5
are displayed in the output. This method should not be used when the list size is small.
Conclusion
Python provides you with a variety of methods for multiplying list members by a scalar, allowing you to select the way that best meets your individual needs. Whether you prefer the simplicity of list comprehensions, the versatility of the map()
function, the brevity of lambda functions, or the efficiency of NumPy
arrays, Python provides adaptable tools for your data manipulation tasks.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python