Python Multi-Line if Condition
- the Importance of Readable Code
-
Python Multiline
if
Condition: Backslash at the End of Each Line -
Python Multiline
if
Condition: Knuth’s Style -
Python Multiline
if
Condition: Defining avariable
- Conclusion
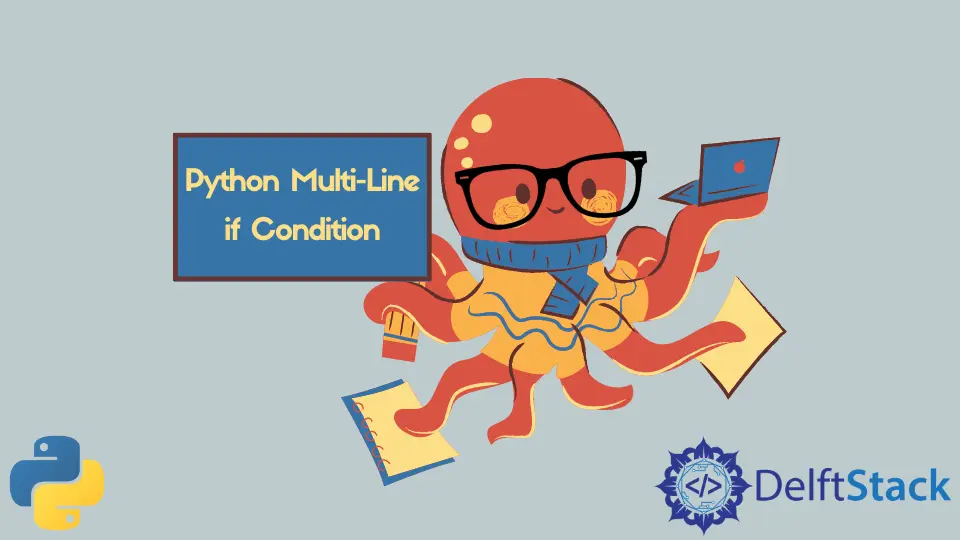
One of the key principles of writing clean and maintainable code in Python is adhering to style guidelines, and this extends to how we structure our conditional statements.
In particular, when dealing with multi-line conditions within if
statements, employing a consistent and readable style becomes paramount. This article explores the best practices for styling multi-line conditions in Python if
statements, with a focus on clarity and adherence to PEP8 guidelines.
the Importance of Readable Code
Readable code is crucial for collaboration and maintainability. It allows developers to quickly understand the logic and intentions behind the code, making it easier to debug, modify, and extend.
When dealing with complex conditions in if
statements, adopting a clean and consistent style not only adheres to best practices but also enhances the overall readability of the codebase.
For starters, the multiple condition statements should not be placed in a single line. Instead, split this single line of the multiple conditions and wrap them in parentheses.
Example code:
# Assume these are the conditions (for illustration purposes)
firstcondition = "something"
secondcondition = "something else"
thirdcondition = "something different"
# Check if all conditions are met
if (
firstcondition == "something"
and secondcondition == "something else"
and thirdcondition == "something different"
):
# Perform some action when conditions are met
print("All conditions are met. Performing the action.")
else:
# This block will be executed if conditions are not met
print("Conditions are not met. No action performed.")
Output:
All conditions are met. Performing the action.
The code snippet above outputs "All conditions are met. Performing the action."
since it has met all the conditions inside the if
statement.
PEP8 guides the use of continuation lines to separate the multi-line condition statements. These lines should be vertically aligned and spaced with a four-space indentation from the beginning of the new line. The closing parenthesis and the colon clearly show the end of the condition.
Apparently, Python has an automatic way of creating a four-space indentation whenever you combine a two-character keyword like if
, a single space, and an opening parenthesis. Therefore, there is no need to add extra indentation in the condition break.
Example code:
if (this_matches_condition, this_also_matches_condition): # end of conditions
get_something_done()
If you style the multiple conditions like above, there is an advantage of easy readability of code. It also makes the complex conditions have a cleaner expression.
Python Multiline if
Condition: Backslash at the End of Each Line
Using a backslash (\
) at the end of each line is a method to visually break a long line of code into multiple lines, making it more readable. This is particularly useful when dealing with complex conditions in if
statements.
Also, the backslash at the end of each line indicates a continuation of the condition. It tells Python that the line should be treated as a logical continuation of the previous line.
The indentation ensures the conditions are visually aligned, contributing to better readability.
Example code:
# Assume these are the conditions (for illustration purposes)
condition1 = True
condition2 = False
condition3 = True
# Check if all conditions are met
if condition1 and condition2 and condition3:
# Perform some action when conditions are met
print("All conditions are met. Performing the action.")
else:
# This block will be executed if conditions are not met
print("Conditions are not met. No action performed.")
Output:
All conditions are met. Performing the action.
The code snippet above outputs "All conditions are met. Performing the action."
since it has met all the conditions inside the if
statement.
Python Multiline if
Condition: Knuth’s Style
In a scenario where logic operator keywords like and
and or
are included in the conditions, it is highly advisable, according to the PEP8 guide, to place the logic operator(and, or) before the continuation condition lines.
This style is referred to as Knuth’s style. It enhances the readability of the conditions due to the automatic four-space indentation set by Python to make all conditions vertically line up.
It also makes the conditions more visible within the parenthesis and brings along the advantage of short-circuit evaluation.
Example code:
# Assume these are the statements and name (for illustration purposes)
sttmt1 = True
sttmt2 = False
sttmt3 = True
name = "Goodman"
# Check the conditions
if sttmt1 and (sttmt2 or sttmt3) or name == "Goodman":
# Something happens when the conditions are met
print("Something happens.")
else:
# This block will be executed if conditions are not met
print("No action performed.")
<!--
test:
command: python
expected_output: |
Something happens.
-->
Output:
```text
Something happens.
The code snippet above outputs "Something happens.."
since it has met all the conditions inside the if
statement.
Python Multiline if
Condition: Defining a variable
An alternative way to style these multi-line condition statements is by defining a variable
and assigning these condition expressions to it.
This method is not entirely encouraged because it tends to limit subsequent refactoring. However, the code will look clearer if a variable is used, and it also provides a better understanding of the program control flow.
Example code:
# Assume these are the conditions (for illustration purposes)
condition_one = "number1"
condition_two = "number2"
condition_three = "number3"
<!--undrads7-->
# Check the conditions
condition_list = (
condition_one == "number1"
and condition_two == "number2"
and condition_three == "number4"
)
if condition_list:
# Implement something when conditions are met
print("Implementing something.")
else:
# Do something else when conditions are not met
print("Doing something else.")
Output:
Doing something else.
The if statement in the above code ensures that something is implemented if and only if the entire condition_list
is true. Otherwise, something else is implemented when even one of the conditions in the condition_list
is false.
We encountered the Doing something else.
as output since the condition in the variable condition_three
has not been met.
Conclusion
Readable code is crucial for collaboration and ease of maintenance, especially when dealing with complex conditions. Whether using parentheses, line continuation with backslashes, Knuth’s style, or defining variables, each approach has its merits.
The automatic four-space indentation in Python simplifies the process, leading to cleaner and more expressive code.
Adhering to consistent and readable styles in Python is essential for clean and maintainable code, particularly when structuring multi-line conditions in if
statements. Following PEP8 guidelines ensures clarity and adherence to best practices.
Choose a styling method that aligns with the project’s guidelines to enhance code aesthetics and understanding.