The Short Circuit Evaluation in Python
- Logical Operators in Python
- What Is Short-Circuiting
-
Short-Circuiting Using
AND
Operator in Python -
Short-Circuiting Using
OR
Operator in Python
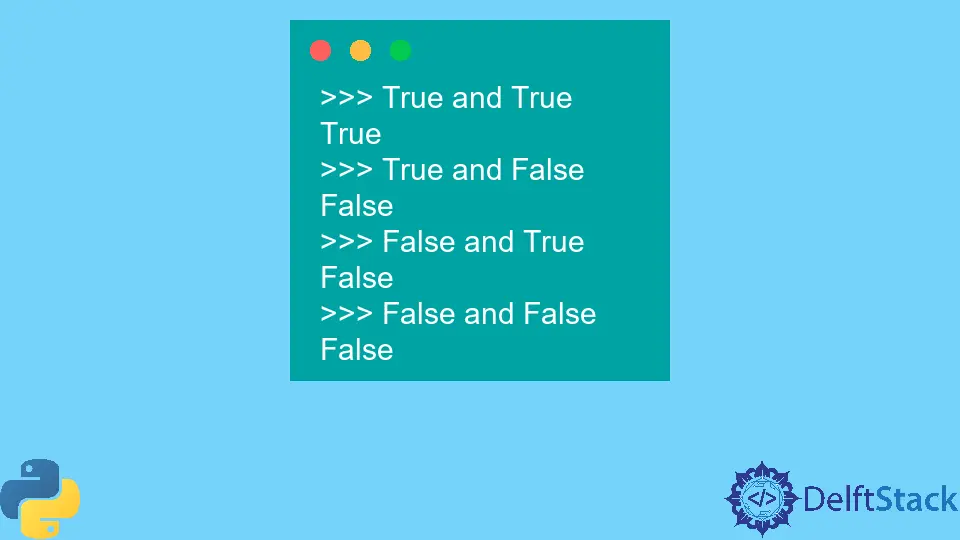
This article is about showing short-circuiting behavior in Python using logical operators.
Logical Operators in Python
the OR
Operator
OR
: Both operands are evaluated using the Python or
operator. The or
operator returns True
if either of the operands is True
.
However, the or
operator will return False
only if all the given expression or operands returns False
.
Logic table of OR
operator:
First Value | Second Value | Output |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
Representation of the above OR
operator in the Python console:
>>> True or True
True
>>> True or False
True
>>> False or True
True
>>> False or False
False
Python also allows us to compare multiple expressions using the OR
operator.
>>> (5 < 10) or (8 < 5)
True
>>> (5 < 10) or (8 < 5) or (2 == 2) or (9 != 8)
True
the AND
Operator
AND
: When using the Python and
operator, both operands are evaluated, and if any given expression or operator is not true, then False
is returned. The and
operator only returns True
when both the given expression or operands is True
.
Logic table of AND
operator:
First Value | Second Value | Output |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
Representation of the above AND
operator in the Python console:
>>> True and True
True
>>> True and False
False
>>> False and True
False
>>> False and False
False
Comparing multiple expressions using the AND
operator.
>>> (5 < 10) and (8 < 5)
False
>>> (5 < 10) and (8 < 5) and (2 == 2) and (9 != 8)
False
>>> (10 == 10) and (8 != 5) and (2 == 2) and (9 != 8)
True
What Is Short-Circuiting
Short-circuiting refers to the termination of a Boolean operation when the expression’s truth value has already been established. The Python interpreter evaluates the expression in a left-to-right fashion.
Python’s numerous Boolean operators and functions allow short-circuiting.
def exp(n):
print("Hello")
return n
To see how short-circuiting takes place, we will use the above function as one of the operands or expressions, which will print the word "Hello"
when the Python interpreter executes it.
Short-Circuiting Using AND
Operator in Python
Using the and
operator:
>>> True and exp(1)
Hello
1
The Python interpreter evaluates the code from left to right in the above code. According to the logic table of the AND
operator, the expression must be True
to get a True
Boolean value.
The Python interpreter evaluates our function only because the first value is set to True
.
What if we set the initial value to False
? Observe the below code:
>>> False and exp(1)
False
Since the initial value is set to False
, the Python interpreter ignores the latter expression, saving the execution time.
Swapping the Expression:
>>> exp(1) and True
Hello
True
>>> exp(1) and False
Hello
False
In these codes, our initial expression is the function we created earlier. The Python interpreter evaluates the given function first, resulting in the output "Hello"
.
Note: The function we have created produces no error or
False
Boolean value. Hence, the Python interpreter will always have to evaluate and latter expression when the former is the given function when theand
operator is used.
Short-Circuiting Using OR
Operator in Python
Using the or
operator:
>>> True or exp(1)
True
Evaluating the code from left to right. Setting the initial value to True
allows the Python interpreter to ignore the execution of the latter expression, which is the given function.
Hence, according to the given logic table of the OR
operator, the output is True
.
>>> False or exp(1)
Hello
1
The Python interpreter executes the function in the above code because the former value is set to False
.
Swapping the Expression:
>>> exp(1) or True
Hello
1
>>> exp(1) or False
Hello
1
When we swap the expressions, our created function is executed every time since it is the first operand that the Python interpreter evaluates.
Considering either of the above code examples, the or
operator will return a True
Boolean value.
However, if we get a False
Boolean value using the or
operator, we can tweak the created function a bit.
def exp():
print("Hello")
return False
>>> exp()
Hello
False
After the above changes, whenever we call the exp()
function, it will only return the False
Boolean value after printing Hello
to the console.
>>> exp() or True
Hello
True
>>> exp() or False
Hello
False
Since the function only returns a False
Boolean value on every call, the Python interpreter must evaluate the latter expression or operand.