Python Multiple if Statements on One Line
-
Write Multiple
if
Statements on One Line in Python -
Use Ternary Conditional Expression to Write Multiple
if
Statements on One Line in Python -
Use the
any
Function and a List Comprehension to Write Multipleif
Statements on One Line in Python - Conclusion
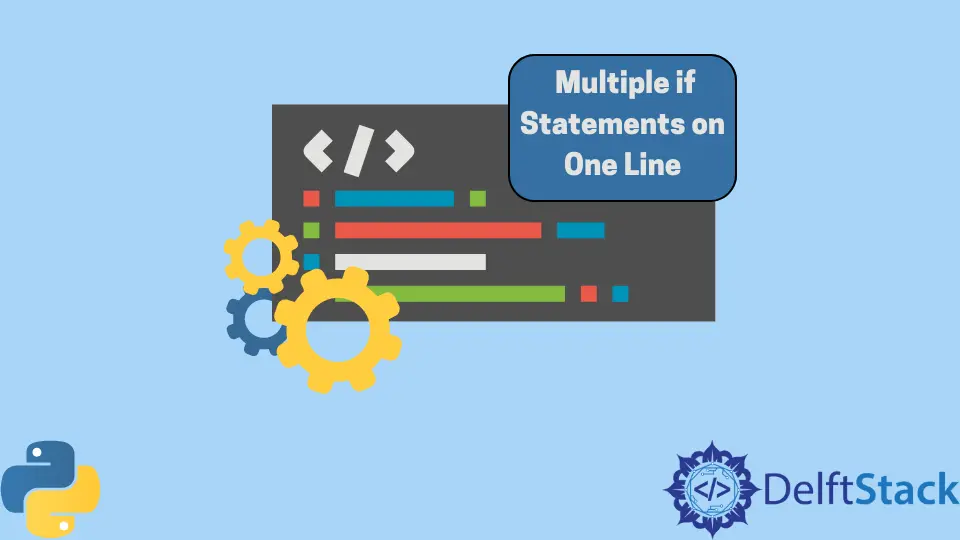
Whenever we write an if-elif-else
block, we write them in separate lines. But there is a way to write those statements in one line, too.
However, it’s not recommended because it reduces the readability and understandability of the code. But for general knowledge, we can write those statements in one line of code.
We’ll discuss in this tutorial how to write an if-elif-else
statement on one line in Python.
Write Multiple if
Statements on One Line in Python
In Python, it is possible to write multiple if
statements on one line. This allows you to create a concise and compact code structure to handle multiple conditions.
But first, we will see how to write an if-elif-else
in separate lines, like in the following example.
Example Code:
a = 2
b = 3
if a < b:
print("a is less than b")
elif a == b:
print("a is equal to b")
else:
print("a is greater than b")
Output:
a is less than b
Now, if we want to write the same if-elif-else
block of code in a single line, we can write the code as follows.
Example Code:
a = 2
b = 3
result = (
"a is less than b"
if a < b
else ("a is equal to b" if a == b else "a is greater than b")
)
print(result)
Output:
a is less than b
In the following example, the if-else
statement is nested inside another if-else
statement. It allows for a sequence of conditions to be checked hierarchically.
This is useful when we have multiple conditions that are not mutually exclusive.
In the code, the first condition a < b
is checked.
If true, the result is set to "a is less than b"
. If the first condition is false, the second condition a == b
is checked, and if true, the result is set to "a is equal to b"
.
If both conditions are false, the result is set to "a is greater than b"
. Since a
is less than b
, the first condition a < b
is true, and the output would be a is less than b
.
Use Ternary Conditional Expression to Write Multiple if
Statements on One Line in Python
The ternary conditional expression allows us to write a more concise one-liner for simple conditional checks. It is particularly useful when we have mutually exclusive conditions and want to select one value based on the evaluation of these conditions.
The nested ternary conditional expression checks the first condition, and if it’s true, the corresponding result is assigned. If the first condition is false, the second condition is checked, and so on.
Example Code:
a = 2
b = 3
result = (
"a is less than b"
if a < b
else "a is equal to b"
if a == b
else "a is greater than b"
)
print(result)
Output:
a is less than b
In this code, we have two variables, a
and b
. We use a ternary conditional expression to check three conditions in a single line.
The first condition, a < b
, evaluates to true, so the result is set to a is less than b
. Therefore, when we print the result using print(result)
, the output is a is less than b
.
This concise expression allows us to determine the relationship between a
and b
and output the corresponding result in just a single line of code.
Use the any
Function and a List Comprehension to Write Multiple if
Statements on One Line in Python
The any
function in Python is a built-in function that returns True
if at least one element of an iterable (such as a list, tuple, or other iterable) is true. If the iterable is empty, it returns False
.
Here, we use the any
function along with a list comprehension to check if at least one condition is true.
a = 2
b = 3
result_with_any = next(
(
msg
for msg in [
"a is less than b" if a < b else None,
"a is equal to b" if a == b else None,
"a is greater than b" if a > b else None,
]
if any(
msg is not None
for msg in [
"a is less than b" if a < b else None,
"a is equal to b" if a == b else None,
"a is greater than b" if a > b else None,
]
)
),
None,
)
print(result_with_any)
Output:
a is less than b
In this code, we have two variables, a
and b
. The result_with_any
variable is assigned the first non-None value from a list comprehension that contains messages based on the comparison of a
and b
.
The conditions include whether a
is less than b
, equal to b
, or greater than b
. The any
function is used to check if at least one condition is true.
The print
statement outputs the result, which is a is less than b
since a
is indeed less than b
.
However, it’s important to note that using such complex one-liners may sacrifice readability. In practical scenarios, it’s often better to use more explicit and clear code, especially when dealing with multiple conditions.
Conclusion
We explored different methods to write multiple if
statements on one line in Python. We first highlighted the traditional approach of using separate lines for if-elif-else
blocks, emphasizing that condensing them into a single line is possible but not recommended due to reduced code readability.
We presented an example of the conventional block structure followed by an output. Subsequently, we delved into two concise alternatives: using a ternary conditional expression and employing the any
function with a list comprehension.
These methods provide a more compact syntax for simple conditions, but it’s crucial to exercise caution as overly complex one-liners might compromise code readability. Each example was accompanied by relevant code snippets and their respective outputs to illustrate the practical implications of these approaches.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn