Nested try...except Statements in Python
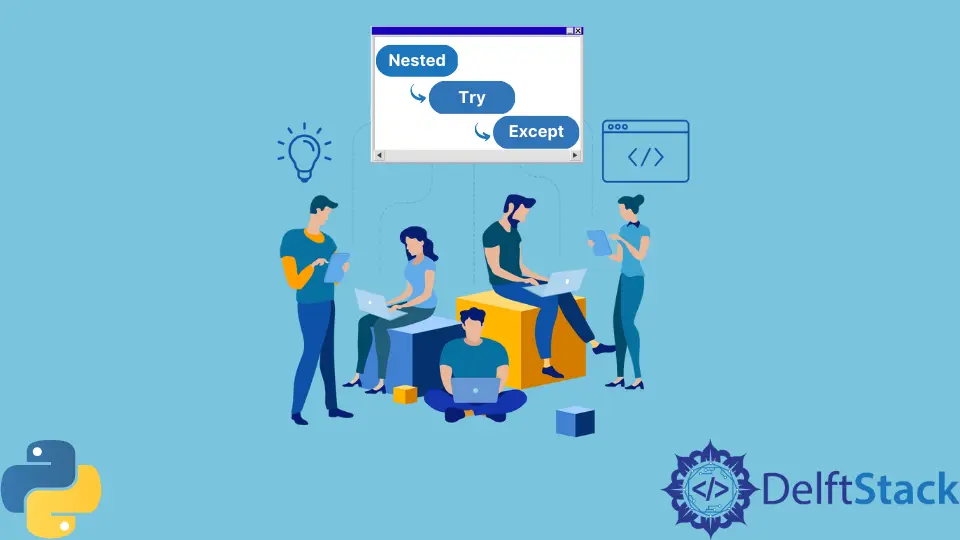
The try...except
statement is used in Python to catch exceptions or run some code prone to errors. Every programming language has this feature these days, but in Python, it goes by these words and is represented by try...except
keywords, respectively. Apart from try...except
, another keyword, namely, finally
, could also be used together with them.
Like for
loops, these try
, catch
, and finally
statements can also be nested, and In this article, we will talk about it.
Nested try...except
Statements in Python
As mentioned above, we can nest these statements the same way we nest for
loops. Refer to the following code for an example.
a = {"a": 5, "b": 25, "c": 125}
try:
print(a["d"])
except KeyError:
try:
print("a:", a["a"])
except:
print("No value exists for keys 'a' and 'd'")
finally:
print("Nested finally")
finally:
print("Finally")
Output:
a: 5
Nested finally
Finally
As we can see, the above program first initializes a dictionary with some key-value pairs and then tries to access the value for the key d
. Since no key-value pair exists, a KeyError
exception is raised and caught by the except
statement. Then the interpreters run the code under the nested try
block. Since a value exists for key a
, it is printed to the console, and the code under the nested finally
statement is executed. Lastly, the code under the outer finally
statement is executed.
This means that we can put try
, catch
, and finally
statements under any try
, catch
, and finally
statements. Let understand this with an example. We will write some code that has try
, catch
, and finally
statements and all these statements also have try
, catch
, and finally
statements under them.
a = {
"a": 5,
"b": 25,
"c": 125,
"e": 625,
"f": 3125,
}
try:
try:
print("d:", a["d"])
except:
print("a:", a["a"])
finally:
print("First nested finally")
except KeyError:
try:
print("b:", a["b"])
except:
print("No value exists for keys 'b' and 'd'")
finally:
print("Second nested finally")
finally:
try:
print("c:", a["c"])
except:
print("No value exists for key 'c'")
finally:
print("Third nested finally")
Output:
a: 5
First nested finally
c: 125
Third nested finally
As we can see, first, the outer try
block is executed. Since no value is found for key d
, the code under the nested except
statement is executed, and the nested finally
. Since the outer try
block did not get any exceptions during the execution, its except
block is skipped, and the code under the outer finally
block is executed.
We can even take this further as we want and create n
levels of nested try
, catch
, and finally
statements. But as the number of nested levels increases, the control flow or execution flow gets a bit complicated and unmanageable. It becomes challenging to navigate through the try
, catch
, and finally
statements.