if Statement With Strings in Python
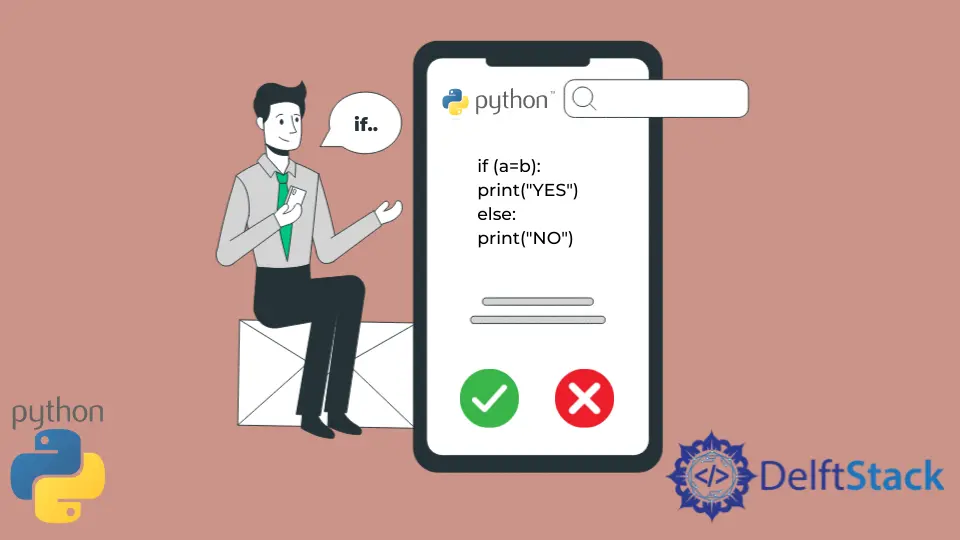
In Python, the if
statement executes a block of code when a condition is met. It is usually used with the else
keyword, which runs a block if the condition in the if
statement is not met.
This article will discuss the use of the if
statement with strings in Python.
A string is a chain of characters, where every character is at a particular index and can be accessed individually.
We can check a string against a set of strings using the in
keyword. The set of strings can be in the form of a list, and even if one element matches, it will execute the if
block.
For example,
a = "y"
if a in ["y", "Y", "yes", "Yes", "YES"]:
print("Match")
else:
print("No match")
Output:
Match
We have to be sure of all the possible matches since Python is case-sensitive. The other way to save time is by eliminating the uppercase or lowercase inputs by converting them into one form before checking the condition in the if
statement. We can use the lower()
or upper()
function to convert the string to a single case.
For example,
a = "YES"
if a.lower() in ["y", "yes"]:
print("Match")
else:
print("No match")
Output:
Match
We can perform string comparisons using the if
statement. We can use relational operators with the strings to perform basic comparisons.
See the code below.
a = "Hamed"
b = "Mark"
if a != b:
print("Not equal")
else:
print("Equal")
if a < b:
print("Two is greater")
else:
print("One is greater")
Output:
Not equal
Two is greater
We performed various operations in the above example.
We can also check whether the characters are unordered but the same by using the sorted()
function in the equality operation. The is
operator can also be used on strings. It checks whether the two objects refer to the same object or not.
For example,
a = "mnba"
b = "nbam"
c = b
if sorted(a) == sorted(b):
print("Equal")
else:
print("Not equal")
if c is b:
print("True")
Output:
Equal
True
In the above example, the two strings were equal when sorted in the proper order. Also, the strings c
and b
refer to the same string. That is why the is
operator returns True
.