How to Find Maximum Value in a List in Python
-
Use the
for
Loop to Find Max Value in a List in Python -
Use the
max()
Function to Find Max Value in a List in Python - Find Max Value in a Nested List in Python
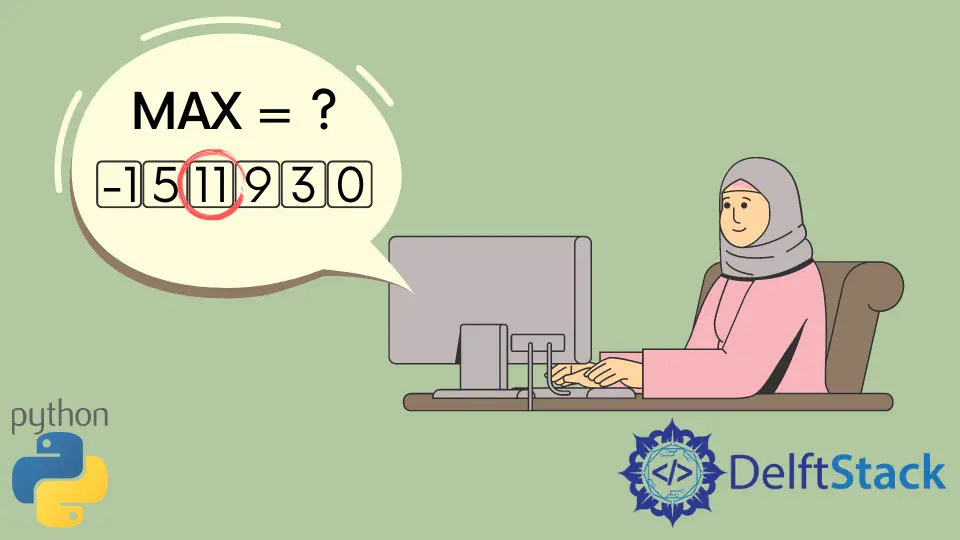
This tutorial will demonstrate how to find the maximum value in a list in Python.
A few scenarios and data types will be covered, from a simple integer list to a more complex structure such as an array within an array.
Use the for
Loop to Find Max Value in a List in Python
The Python for
loop can be used to find the max value in a list by comparing each value in the array and storing the largest value in a variable.
For example, let’s declare an array of random integers and print out the max value. Also, declare a variable max_value
to store the maximum value and print it out after the loop is finished.
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = None
for num in numbers:
if max_value is None or num > max_value:
max_value = num
print("Maximum value:", max_value)
Output:
Maximum value: 104
If the index of the maximum value is needed to further manipulate the output, simply use the Python function enumerate
, which enables the for
loop to accept a second argument indicating the index of the current iteration of the loop.
Add a new variable, max_idx
, to store the max index of the maximum value.
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = None
max_idx = None
for idx, num in enumerate(numbers):
if max_value is None or num > max_value:
max_value = num
max_idx = idx
print("Maximum value:", max_value, "At index: ", max_idx)
Output:
Maximum value: 104 At index: 4
Use the max()
Function to Find Max Value in a List in Python
Python has a pre-defined function called max()
that returns the maximum value in a list.
Finding the maximum value using max()
will only need a single line.
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = max(numbers)
print("Maximum value:", max_value)
Output:
Maximum value: 104
If you need the index of the maximum value, we can call the built-in index()
function in a Python list. The function will return the index of the specified element within the array.
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = max(numbers)
print("Maximum value:", max_value, "At index:", arr.index(max_value))
Output:
Maximum value: 104 At index: 4
Use max()
to Find Max Value in a List of Strings and Dictionaries
The function max()
also provides support for a list of strings and dictionary data types in Python.
The function max()
will return the largest element, ordered by alphabet, for a list of strings. The letter Z is the largest value, and A is the smallest.
strings = ["Elephant", "Kiwi", "Gorilla", "Jaguar", "Kangaroo", "Cat"]
max_value = max(strings)
print("Maximum value:", max_value, "At index:", strings.index(max_value))
Output:
Maximum value: Kiwi At index: 1
Find Max Value in a Nested List in Python
A more complicated example is to find the maximum value of a nested list in Python.
First, let’s declare random values in a nested list.
nst = [[1001, 0.0009], [1005, 0.07682], [1201, 0.57894], [1677, 0.0977]]
From this nested list, we want to get the maximum value of the 2nd element. We also want to print out the 1st element and treat it as an index. The nested list is treated more like a key-value pair within a list.
To find the maximum value of this complex structure, we can use the function max()
with its optional argument key
, which takes note of the element within the nested list to compare.
nst = [[1001, 0.0009], [1005, 0.07682], [1201, 0.57894], [1677, 0.0977]]
idx, max_value = max(nst, key=lambda item: item[1])
print("Maximum value:", max_value, "At index:", idx)
The key
argument accepts a lambda function and will allow the function max()
to return a key-value pair.
Output:
Maximum value: 0.57894 At index: 1201
In summary, this tutorial covered different methods of finding the max values within different types of lists in Python. You can read more about the function max()
and its different arguments here. Aside from max()
there are built-in functions like min()
, all()
, and any()
that manipulate iterables in Python.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python