Max Int in Python
- Maximum Integer Value in Python 2
- Maximum Integer Value in Python 3
- Practical Implications of Integer Limits
- Conclusion
- FAQ
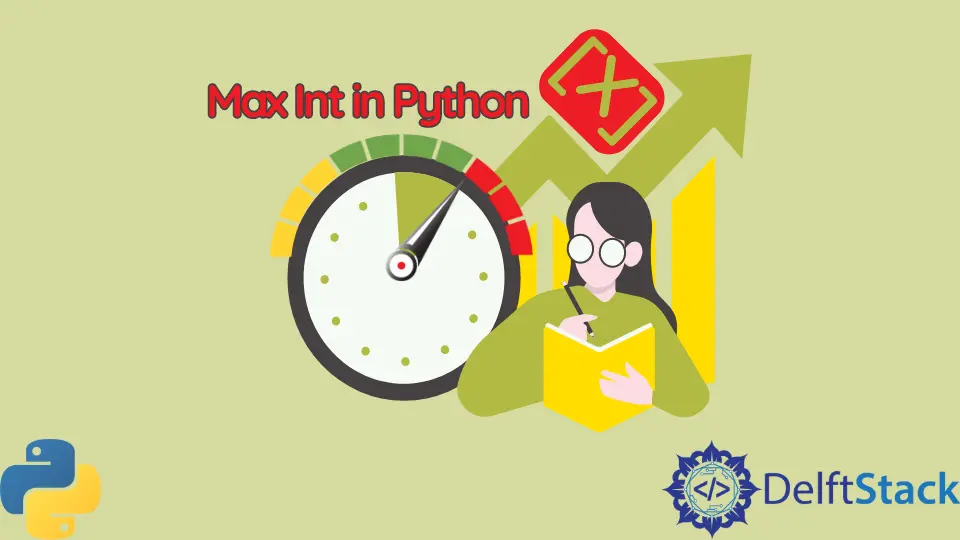
In the world of programming, understanding data types and their limits is crucial for writing efficient and error-free code. One such data type that often raises questions is the integer. Specifically, many developers wonder about the maximum integer value that can be handled in different versions of Python.
This tutorial will delve into the concept of maximum integer values in Python, exploring how they have evolved from Python 2 to Python 3. Whether you are a beginner or an experienced programmer, this guide will help you grasp the nuances of integer limits in Python and how to work with them effectively.
Maximum Integer Value in Python 2
In Python 2, the maximum integer value is determined by the system architecture. Essentially, there are two types of integers: int
and long
. The int
type is limited to a maximum value of 2,147,483,647 (for a 32-bit system) or 9,223,372,036,854,775,807 (for a 64-bit system). However, if you exceed this limit, Python automatically converts the integer to a long
type, which can grow as large as the memory allows.
Here’s how you can check the maximum integer value in Python 2:
import sys
max_int = sys.maxint
print(max_int)
Output:
2147483647
This code snippet utilizes the sys
module to access the maxint
attribute, which contains the maximum value for an integer. By running this code, you can quickly determine the maximum integer value for your specific Python 2 environment. It’s important to note that Python 2 has reached its end of life, so transitioning to Python 3 is highly recommended for better performance and newer features.
Maximum Integer Value in Python 3
In contrast to Python 2, Python 3 simplifies the integer handling significantly. The int
type in Python 3 is unbounded, meaning it can grow as large as the memory allows. This change eliminates the need for a separate long
type, making it easier for developers to work with large integers without worrying about data type conversions.
To see the maximum integer value in Python 3, you can use the following code:
import sys
max_int = sys.maxsize
print(max_int)
Output:
9223372036854775807
In this code, the sys.maxsize
attribute gives you the maximum integer value that can be used for indexing containers, which is generally 2^63 - 1 on a 64-bit system. This change allows for greater flexibility and efficiency when handling large numbers, making Python 3 a more robust choice for developers working with numerical data.
Practical Implications of Integer Limits
Understanding the maximum integer values in Python is not just an academic exercise; it has real-world implications for developers. For example, if you are working on applications that require high-performance computations, such as scientific simulations or data analysis, knowing the limits of integer values helps you avoid overflow errors.
In Python 2, if you attempt to perform arithmetic operations that exceed the maximum integer value, Python will switch to the long
type automatically. This can sometimes lead to unexpected behavior in your programs, especially if you’re not aware of the conversion.
In Python 3, the unbounded int
type eliminates this issue, allowing you to perform operations without the fear of hitting a limit. However, it’s essential to keep in mind that while Python can handle large integers, the performance can slow down as the numbers grow larger due to increased memory consumption.
Conclusion
In summary, understanding the maximum integer values in Python is essential for writing efficient code and avoiding common pitfalls associated with integer overflow. Python 2’s limitations regarding integer types can lead to complications, while Python 3’s unbounded int
type simplifies the process significantly. As you continue your programming journey, keeping these differences in mind will help you make informed decisions about data types and their implications on performance. Transitioning to Python 3 not only enhances your coding experience but also equips you with a powerful tool for handling numerical data effectively.
FAQ
-
what is the maximum integer value in Python 2?
The maximum integer value in Python 2 is 2,147,483,647 for a 32-bit system and 9,223,372,036,854,775,807 for a 64-bit system. -
how does Python 3 handle integers differently than Python 2?
Python 3 uses an unboundedint
type, allowing integers to grow as large as memory allows, eliminating the need for a separatelong
type. -
what happens if I exceed the maximum integer value in Python 2?
If you exceed the maximum integer value in Python 2, the integer will automatically convert to along
type, which can handle larger values. -
is it necessary to transition to Python 3 for integer handling?
Yes, transitioning to Python 3 is recommended for better performance and simplified integer handling, as it eliminates the limitations of Python 2. -
can Python 3 handle very large integers efficiently?
While Python 3 can handle very large integers, performance may decrease due to increased memory usage as the numbers grow larger.