How to Perform List Subtraction in Python
- Method 1: List Comprehensions
- Method 2: Using the Set Data Structure
- Method 3: The filter() Function
- Conclusion
- FAQ
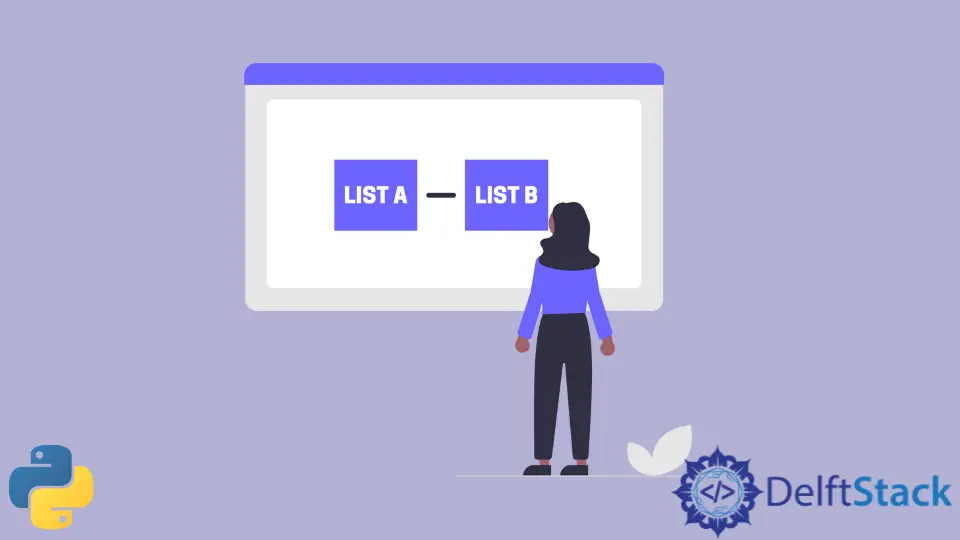
When working with lists in Python, you may encounter situations where you need to subtract one list from another. List subtraction can be particularly useful in various applications, such as data analysis, filtering, and cleaning datasets.
In this article, we will explore different methods to perform list subtraction in Python. We’ll cover techniques using list comprehensions, the set
data structure, and the filter()
function. Each method will be explained with clear examples, enabling you to understand how to implement list subtraction effectively. Whether you’re a beginner or an experienced programmer, this guide will enhance your Python skills and help you manipulate lists with ease.
Method 1: List Comprehensions
List comprehensions provide a concise way to create lists in Python. They allow you to generate a new list by applying an expression to each element in an existing list. To perform list subtraction, you can use a list comprehension to include only the elements from the first list that are not present in the second list.
Here’s how it works:
list_a = [1, 2, 3, 4, 5]
list_b = [2, 4]
result = [item for item in list_a if item not in list_b]
Output:
[1, 3, 5]
In this example, list_a
contains the numbers 1 through 5, while list_b
includes the numbers 2 and 4. The list comprehension iterates over each element in list_a
and checks if it is not in list_b
. If the condition is true, the element is included in the new list. The result is a new list containing only the elements from list_a
that are not found in list_b
. This method is efficient and easy to read, making it a popular choice for list subtraction in Python.
Method 2: Using the Set Data Structure
Another effective way to perform list subtraction in Python is by utilizing the set
data structure. Sets are unordered collections of unique elements, which makes them ideal for operations like subtraction. By converting lists to sets, you can easily find the difference between them.
Here’s how to do it:
list_a = [1, 2, 3, 4, 5]
list_b = [2, 4]
result = list(set(list_a) - set(list_b))
Output:
[1, 3, 5]
In this example, both list_a
and list_b
are converted to sets. The subtraction operation (-
) is performed between the two sets, resulting in a new set that contains only the elements present in list_a
but not in list_b
. Finally, the result is converted back to a list. This method is particularly efficient for larger datasets, as set operations are generally faster than list operations. However, keep in mind that converting lists to sets removes any duplicate elements, which may or may not be desirable based on your specific needs.
Method 3: The filter() Function
The filter()
function in Python allows you to filter elements from a list based on a specified condition. This function can also be used to achieve list subtraction by filtering out elements that are present in the second list.
Here’s an example:
list_a = [1, 2, 3, 4, 5]
list_b = [2, 4]
result = list(filter(lambda x: x not in list_b, list_a))
Output:
[1, 3, 5]
In this case, the filter()
function takes two arguments: a lambda function and the list to be filtered. The lambda function checks if each element x
from list_a
is not in list_b
. The filter()
function returns an iterator, which is then converted back to a list. The result is a new list containing only the elements from list_a
that are not found in list_b
. This method is quite flexible and can be easily adapted for more complex filtering conditions.
Conclusion
In this article, we explored three effective methods for performing list subtraction in Python: list comprehensions, the set data structure, and the filter()
function. Each method has its own advantages, and the choice of which to use may depend on your specific use case, such as performance considerations or the need to maintain duplicates. By mastering these techniques, you can enhance your ability to manipulate lists in Python, making your code cleaner and more efficient. Whether you’re working on data analysis, cleaning datasets, or simply exploring the capabilities of Python, these methods will serve you well.
FAQ
-
What is list subtraction in Python?
List subtraction refers to the process of removing elements from one list that are present in another list. -
Can I perform list subtraction with duplicate elements?
Yes, but using sets will remove duplicates. If you want to maintain duplicates, use list comprehensions or the filter() function. -
What is the fastest method for list subtraction?
Using sets is generally the fastest method for list subtraction, especially with larger datasets. -
Are there any limitations to using list comprehensions?
List comprehensions can become less readable with complex conditions, so it’s essential to balance readability and efficiency.
- Can I subtract more than one list from another?
Yes, you can extend the methods to handle multiple lists by iterating through them or using set operations.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python