How to Pass Multiple Arguments in Lambda Functions in Python
- Lambda Functions in Python
-
Pass Multiple Arguments in
Lambda
Functions -
Use
Lambda
Functions With Multiple Arguments in Different Contexts - Conclusion
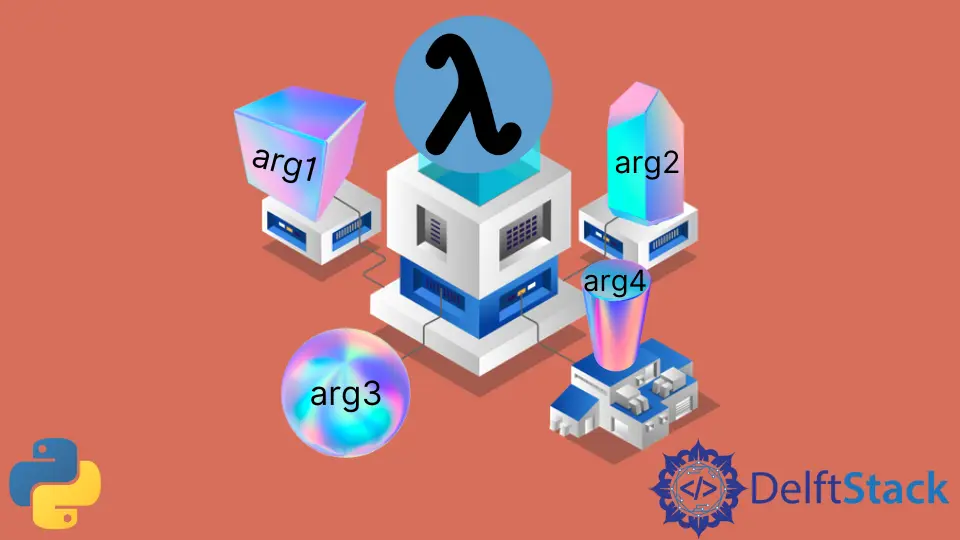
The lambda
forms or lambda
expressions are anonymous functions in Python. They are inline functions that can be created using the reserved lambda
keyword in Python.
In this article, readers can anticipate a comprehensive exploration of lambda
functions with multiple arguments in Python.
Lambda Functions in Python
In Python, a lambda
function is a concise way to create small, anonymous functions without the need for a formal def
statement. It is defined using the lambda
keyword, followed by a list of arguments, a colon, and an expression.
Lambda
functions are particularly useful for short-lived operations where a full function definition would be overkill. They are commonly employed for tasks like filtering, mapping, and sorting data.
The simplicity of lambda
functions lies in their compact syntax, making them suitable for quick, on-the-fly operations. However, it’s important to note that lambda
functions are limited to single expressions and are best suited for straightforward tasks that don’t require complex logic.
The syntax of a lambda
function in Python is quite simple. It follows the form:
lambda arguments: expression
In Python, a lambda
function is created using the lambda
keyword, and it consists of four main components.
Component | Description |
---|---|
lambda keyword |
Serves as an indicator for the creation of a lambda function. |
arguments |
Represents the input parameters the lambda function takes. The number of arguments can vary based on needs. |
: (colon) |
Acts as a separator, distinguishing the arguments from the final component, the expression . |
expression |
A single line of code that the lambda function evaluates and returns as its output. |
Understanding each of these components is crucial for effectively using lambda
functions, where simplicity and conciseness are key.
Let’s begin our exploration with a concrete example. Consider a scenario where we have a conventional function defined using the def
keyword, and we want to employ a lambda
function for a specific operation within this function.
The goal is to showcase the simplicity and efficiency of lambda
functions, especially when dealing with a single argument.
def process_data(numbers):
# Define a lambda function to square a number
def square(x):
return x**2
# Process each number using the lambda function
squared_numbers = [square(num) for num in numbers]
return squared_numbers
# Test the function
data = [1, 2, 3, 4, 5]
result = process_data(data)
print(result)
In this code snippet, we start with a traditional function, process_data
, declared using the def
keyword, designed to take a list of numbers as its sole parameter. Within this function, a lambda
function named square
is introduced using the lambda
keyword.
This brief lambda
function is tailored to operate on a single argument, x
, and computes its square, expressed as x ** 2
. The lambda
function’s effectiveness is exemplified in the subsequent step, where a list comprehension is employed.
The lambda
function dynamically processes each element in the input list (numbers
), generating a new list containing the squared numbers (squared_numbers
).
Finally, the function concludes by returning the computed list of squared numbers.
Output:
[1, 4, 9, 16, 25]
This concise and purpose-driven approach showcases the seamless integration of a lambda
function within a conventional function, making the code both readable and efficient.
Pass Multiple Arguments in Lambda
Functions
While single-argument lambda
functions are straightforward, the concept truly shines when we extend it to handle multiple arguments. This capability adds a layer of flexibility and utility, enabling developers to tackle a broader range of coding challenges.
Here’s the general syntax for a lambda
function with multiple arguments:
lambda argument1, argument2, ..., argumentN: expression
Feel free to replace argument1, argument2, ..., argumentN
with the actual names you want to use for your function arguments.
Let’s dive into a concrete example that illustrates the prowess of lambda
functions with multiple arguments.
Consider a scenario where we have a list (alist
) and two integers (add_value
and subtract_value
). The objective is to use a lambda
function to add add_value
and subtract subtract_value
from each element in the list.
def process_list_with_lambda(alist, add_value, subtract_value):
# Define a lambda function with two parameters
def process_element(x, y):
return x + add_value - subtract_value
# Use lambda function with map to process each element in the list
processed_list = list(
map(lambda elem: process_element(elem, subtract_value), alist)
)
return processed_list
# Test the function
input_list = [1, 2, 3, 4, 5]
result = process_list_with_lambda(input_list, 10, 2)
print(result)
In this code segment, we kick off with the definition of the process_list_with_lambda
function using the def
keyword. This function is designed to operate on three parameters: alist
, representing the list to be processed, add_value
, an integer to be added to each element; and subtract_value
, an integer to be subtracted from each element.
Inside this function, we introduce a lambda
function named process_element
. This compact lambda
function takes two parameters, x
and y
, and yields the result of the expression x + add_value - subtract_value
.
The true strength of lambda
functions with multiple arguments comes to light as we employ the map
function. Through this, we seamlessly apply the lambda
function to each element in the input list (alist
), dynamically transforming each element based on the specified expression.
Executing the provided code with the test data (input list [1, 2, 3, 4, 5]
, add_value
as 10
, and subtract_value
as 2
) would yield the following output:
[9, 10, 11, 12, 13]
This concise and purpose-driven approach showcases the efficacy of lambda
functions in encapsulating complex operations within a concise and readable form.
Use Lambda
Functions With Multiple Arguments in Different Contexts
Mastering lambda functions with multiple arguments is crucial for concise, readable code, especially in functional programming and higher-order functions. This knowledge empowers developers to simplify complex operations, utilize lambda
functions in list comprehensions, and enhance code modularity.
Use Case | Code Snippet | Description |
---|---|---|
Lambda Function for Addition |
add = lambda x, y: x + y |
The first lambda function, add , takes two arguments, x and y , and returns their sum. |
Lambda Function as an Argument to map |
numbers = [1, 2, 3, 4, 5] squared = list(map(lambda x: x**2, numbers)) |
The map function applies the lambda function to square each element in the numbers list, producing a new list called squared . |
Conditional Operation in a Lambda Function |
check_even = lambda x: 'Even' if x % 2 == 0 else 'Odd' |
The lambda function check_even checks if a number is even or odd and returns the corresponding string. |
Lambda Function with Default Values |
power = lambda x, n=2: x**n |
The power lambda function calculates the power of x to the given exponent n , defaulting to 2 if n is not provided. |
Using Lambda with the sorted Function |
students = [('Alice', 25), ('Bob', 22), ('Charlie', 28)] sorted_students = sorted(students, key=lambda student: student[1]) |
The sorted function is used to sort the students list based on the second element of each tuple (age) using a lambda function. |
Lambda Function in max and min Functions |
find_max = lambda x, y: x if x > y else y |
The find_max lambda function is employed to find the maximum of two numbers. |
Lambda Function for Arithmetic Operations |
arithmetic_operation = lambda x, y, z: x + y * z |
The arithmetic_operation lambda function performs a basic arithmetic operation with three arguments. |
Lambda Function in List Comprehension |
numbers = [1, 2, 3, 4, 5] squared_even = [x**2 for x in numbers if (lambda x: x % 2 == 0)(x)] |
A lambda function is used within a list comprehension to square only the even numbers in the numbers list. |
This tabular format provides a clear overview of each use case, the associated code snippet, and a brief description of the purpose of each lambda
function.
These examples demonstrate various ways to use lambda
functions with multiple arguments in different contexts. Keep in mind that lambda
functions are generally suitable for short, simple operations, and for more complex tasks, regular named functions may be more appropriate.
Conclusion
Lambda
functions in Python, whether with single or multiple arguments, offer a concise and expressive way to write short-lived, efficient code. Their simplicity and flexibility make them valuable for tasks where a full function definition may be unwarranted.
With single arguments, lambda
functions excel in straightforward operations, while multiple arguments enhance versatility in various programming scenarios. Whether used for simplicity in arithmetic, functional programming constructs, or list comprehensions, lambda
functions empower developers to write clear and efficient code with minimal syntax.
Related Article - Python Function
- How to Exit a Function in Python
- Optional Arguments in Python
- How to Fit a Step Function in Python
- Built-In Identity Function in Python
- Arguments in the main() Function in Python
- Python Functools Partial Function